How to Use AND Operator in IF Statement in VBA
- Understanding the AND Operator in VBA
- Combining Multiple Conditions
- Using AND Operator in Nested IF Statements
- Conclusion
- FAQ
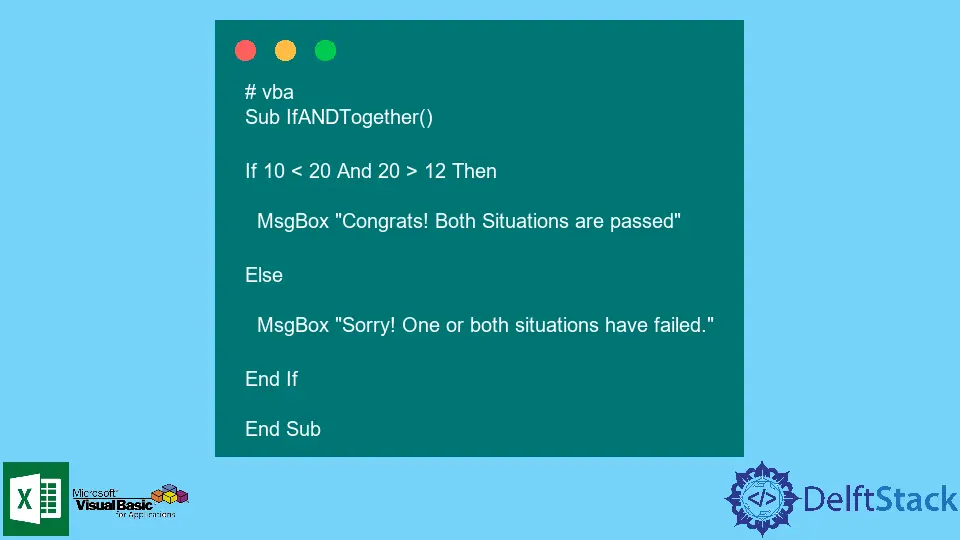
In the world of programming, logic plays a crucial role in decision-making processes. For those working with Visual Basic for Applications (VBA), understanding how to use the AND operator in IF statements is essential for creating robust and efficient code. The AND operator allows you to evaluate multiple conditions simultaneously, leading to more precise control over the flow of your program.
In this tutorial, we will explore the syntax and practical applications of the AND operator within IF statements in VBA. Whether you are a beginner or looking to refine your skills, this guide aims to provide clear examples and explanations to enhance your coding journey.
Understanding the AND Operator in VBA
The AND operator is a logical operator that combines two or more conditions in an IF statement. If all the conditions are true, the overall expression evaluates to true; if any condition is false, it evaluates to false. This functionality is particularly useful when you need to check multiple criteria before executing a block of code.
Basic Syntax of IF Statement with AND Operator
The basic syntax for using the AND operator in an IF statement in VBA is as follows:
If condition1 And condition2 Then
' Code to execute if both conditions are true
End If
In this structure, condition1
and condition2
are the logical expressions you want to evaluate. If both conditions are met, the code within the IF block will execute.
Example of Using AND Operator
Let’s look at a practical example. Suppose you want to check if a user’s age is greater than 18 and if they have a valid ID before allowing them to enter a restricted area. Here’s how you would implement that in VBA:
Dim age As Integer
Dim hasID As Boolean
age = 20
hasID = True
If age > 18 And hasID = True Then
MsgBox "Access Granted"
End If
Output:
Access Granted
In this example, both conditions are true: the age is 20, which is greater than 18, and the user has a valid ID. Therefore, the message box displays “Access Granted.” If either of these conditions were false, the message box would not appear.
Combining Multiple Conditions
You can extend the use of the AND operator to combine more than two conditions. This can be particularly useful when you need to validate a range of criteria before proceeding with an action.
Example of Multiple Conditions
Imagine a scenario where you want to determine if a student qualifies for a scholarship based on their GPA and extracurricular activities. Here’s how you could structure that in VBA:
Dim GPA As Double
Dim extracurriculars As Integer
GPA = 3.5
extracurriculars = 3
If GPA >= 3.0 And extracurriculars >= 2 Then
MsgBox "Scholarship Awarded"
End If
Output:
Scholarship Awarded
In this case, the student has a GPA of 3.5 and participates in three extracurricular activities. Since both conditions are satisfied, the message box confirms that the scholarship is awarded.
Using AND Operator in Nested IF Statements
While the AND operator is powerful on its own, you can also use it in conjunction with nested IF statements for more complex decision-making processes. This approach allows you to evaluate a primary condition and then check additional criteria within that context.
Example of Nested IF Statements
Consider a situation where you want to check if a customer is eligible for a discount based on their membership status and purchase amount. Here’s how you could implement this:
Dim isMember As Boolean
Dim purchaseAmount As Double
isMember = True
purchaseAmount = 150.0
If isMember Then
If purchaseAmount > 100 Then
MsgBox "Discount Applied"
End If
End If
Output:
Discount Applied
In this example, we first check if the customer is a member. If they are, we then check if their purchase amount exceeds $100. Since both conditions are true, the message box indicates that the discount has been applied. This structure allows for clear and logical flows in your code.
Conclusion
Mastering the use of the AND operator in IF statements in VBA is a fundamental skill that can significantly enhance your programming capabilities. By enabling the evaluation of multiple conditions simultaneously, you can create more efficient and effective code. Throughout this tutorial, we explored the syntax, practical examples, and even nested conditions that showcase the versatility of the AND operator. Whether you’re developing applications in Excel, Access, or other Microsoft Office tools, understanding this concept will undoubtedly improve your coding proficiency.
FAQ
-
What is the purpose of the AND operator in VBA?
The AND operator is used to combine multiple conditions in an IF statement, requiring all conditions to be true for the statement to execute. -
Can I use the AND operator with more than two conditions?
Yes, you can combine multiple conditions using the AND operator in a single IF statement. -
What happens if one of the conditions is false?
If any condition combined with the AND operator is false, the entire expression evaluates to false, and the code within the IF block will not execute. -
Is it possible to nest IF statements with the AND operator?
Absolutely! You can use the AND operator in nested IF statements for more complex decision-making.
- Can I use the AND operator with other logical operators?
Yes, the AND operator can be combined with other logical operators like OR and NOT to create more intricate conditions.