OnClick Event Type in TypeScript
-
the
onClick MouseEvent
Interface in TypeScript -
Use the
onClick MouseEvent
in React -
Extend the
MouseEvent
Interface in TypeScript
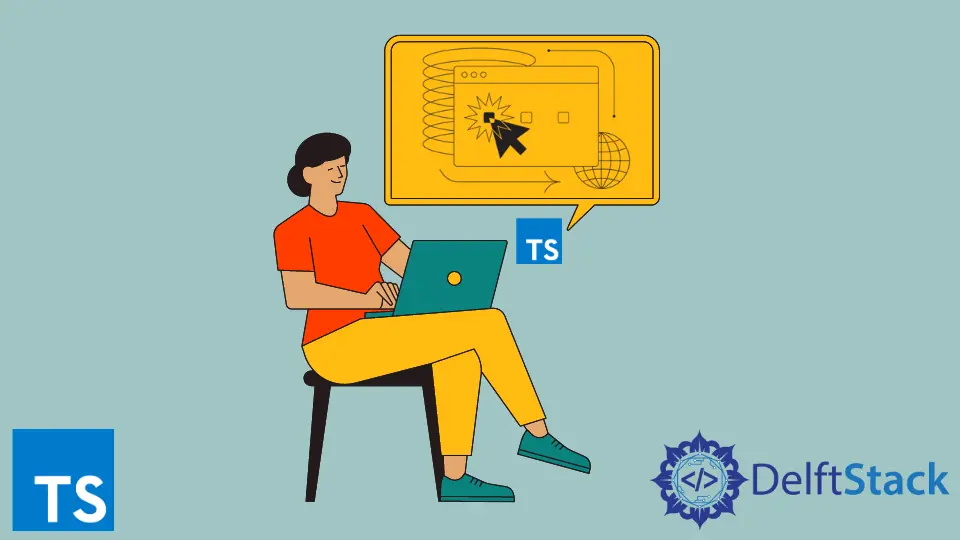
The onClick
event is triggered when the user clicks on some part of an HTML page which can be text, image, or number. Many attributes are associated with a click event, some of which can be used to achieve specific functionalities.
There are various ways to work around onClick
events in TypeScript. This tutorial will demonstrate some of the ways this can be achieved.
the onClick MouseEvent
Interface in TypeScript
The onClick
or MouseEvent
interface is a rich interface with many properties which can be used to add functionality to the code.
interface MouseEvent<T = Element, E = NativeMouseEvent> extends UIEvent<T,E> {
altKey: boolean;
button: number;
buttons: number;
clientX: number;
clientY: number;
ctrlKey: boolean;
movementX: number;
movementY: number;
pageX: number;
pageY: number;
relatedTarget: EventTarget | null;
screenX: number;
screenY: number;
shiftKey: boolean;
}
Various attributes refer to different keypress events or location information associated with a mouse click event. The altKey
indicates whether the Alt key was pressed or not when a given mouse event occurs.
The button
indicates which mouse button was pressed during a click event: 0
for the main button, 1
for the auxiliary button, etc. More information about the button
attribute can be found here.
clientX
and clientY
refer to the floating-point values of the horizontal and vertical coordinate within the application’s viewport at which the event occurred. ctrlKey
indicates whether the alt key was pressed or not when a given mouse event occurs.
pageX
and pageY
refer to the floating-point values of the horizontal and vertical coordinates at which the mouse was clicked. This is relative to the entire document (includes any portion of the document not visible).
screenX
and screenY
refer to the floating-point values of the horizontal and vertical coordinates of the mouse pointer in global (screen) coordinates. The movementX
and movementY
attributes refer to the differences between the current mousemove
event and the previous mousemove
event.
Use the onClick MouseEvent
in React
The MouseEvent
can be triggered on an onClick
event in React. The following code segment will demonstrate how this works:
const ButtonComponent = () => {
const handleButtonClick = (event : React.MouseEvent<HTMLButtonElement, MouseEvent>) => {
console.log(event.target);
console.log(event.currentTarget);
}
return (
<div>
<button onClick={handleButtonClick}>Click me</button>
</div>
)
}
The currentTarget
property on the event gives us access to the element that the event listener is attached to. The target
property on the event refers to the element that triggered the event.
The MouseEvent
can be generalized to HTMLElement
to accept all HTML tags or for any other special tags like HTMLDivElement
.
const DivComponent = () => {
const handleDivClick = (event : React.MouseEvent<HTMLDivElement, MouseEvent>) => {
console.log(event.target);
console.log(event.currentTarget);
}
return (
<div>
<div onClick={handleDivClick}>Hi I am a div</div>
</div>
)
}
Extend the MouseEvent
Interface in TypeScript
interface CustomEventHandler extends MouseEvent<HTMLElement> {
extraField : number
}
Thus the extraField
attribute can be used for handling special events when clicking on some elements in the DOM.