How to Enforce Types for Indexed Members in TypeScript Objects
- Use Mapped Types to Enforce Types for Indexed Members in TypeScript Objects
- Use Generic Types With Mapped Types to Enforce Types for Indexed Members in TypeScript Objects
-
Use the
Record
Type to Enforce Types for Indexed Members in TypeScript Objects
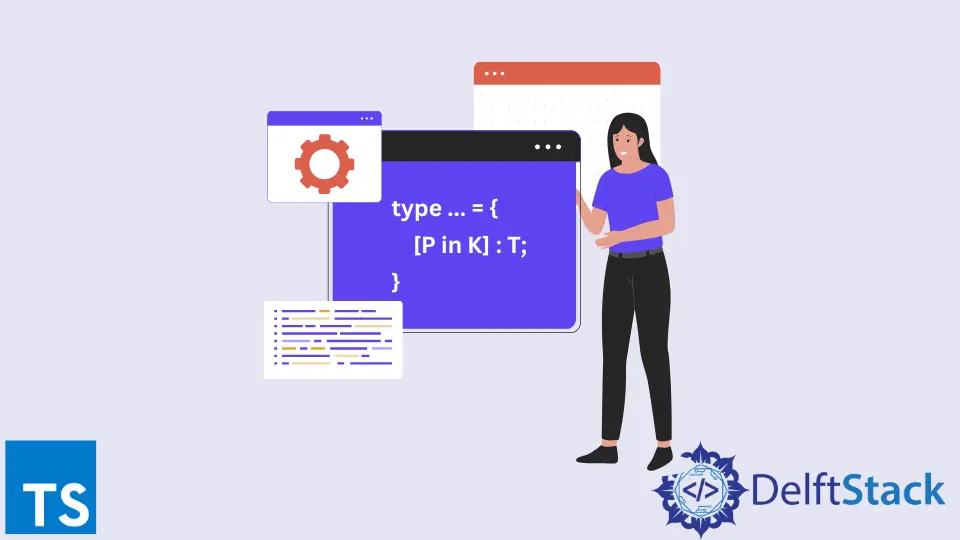
TypeScript is a strongly typed language, and all the constructs used as part of this language are strongly typed. There are constructs like interfaces or types, which are primitive and user-defined types combined.
One can even have complex types like maps or index types, which are under the category of mapped types in TypeScript. This tutorial will demonstrate how mapped types can be used in TypeScript.
Use Mapped Types to Enforce Types for Indexed Members in TypeScript Objects
Mapped types can represent objects with a fixed schema having fixed types for the keys and the values in an object in TypeScript. They are built on the syntax for index signatures.
type NumberMapType = {
[key : string] : number;
}
// then can be used as
const NumberMap : NumberMapType = {
'one' : 1,
'two' : 2,
'three' : 3
}
Other than fixing the type of the keys of an object, mapped types can be used to change the type of all the keys in an object using index signatures.
interface Post {
title : string;
likes : number;
content : string;
}
type PostAvailable = {
[K in keyof Post] : boolean;
}
const postCondition : PostAvailable = {
title : true,
likes : true,
content : false
}
The following uses the keys of the interface Post
and maps them to a different type, Boolean.
Use Generic Types With Mapped Types to Enforce Types for Indexed Members in TypeScript Objects
Mapped types can also be used for creating generic typed objects. Generic types may be used to create types with dynamic types.
enum Colors {
Red,
Yellow,
Black
}
type x = keyof typeof Colors;
type ColorMap<T> = {
[K in keyof typeof Colors] : T;
}
const action : ColorMap<string> = {
Black : 'Stop',
Red : 'Danger',
Yellow : 'Continue'
}
const ColorCode : ColorMap<Number> = {
Black : 0,
Red : 1,
Yellow : 2
}
In the above example, the enum Colors
have been used in the mapped typed object, and different types have been enforced according to the generic type supported by ColorMap<T>
.
Use the Record
Type to Enforce Types for Indexed Members in TypeScript Objects
The Record
type is a built-in type in TypeScript and can be used to enforce types on objects in TypeScript. The Record
type accepts two fields: the types for the keys and the values in an object.
It can be seen as a map found in other programming languages.
const LogInfo : Record<string, string> = {
'debug' : 'Debug message',
'info' : 'Info message',
'warn' : 'Warning message',
'fatal' : 'Fatal error'
}
const message = LogInfo['debug'] + " : Hello world";
console.log(message);
Output:
Debug message : Hello world