Global Variable in TypeScript
- Create a New Project in TypeScript
- What Is a Global Object
- Declare Global Variables in TypeScript
- Access Global Variables in TypeScript
- Conclusion
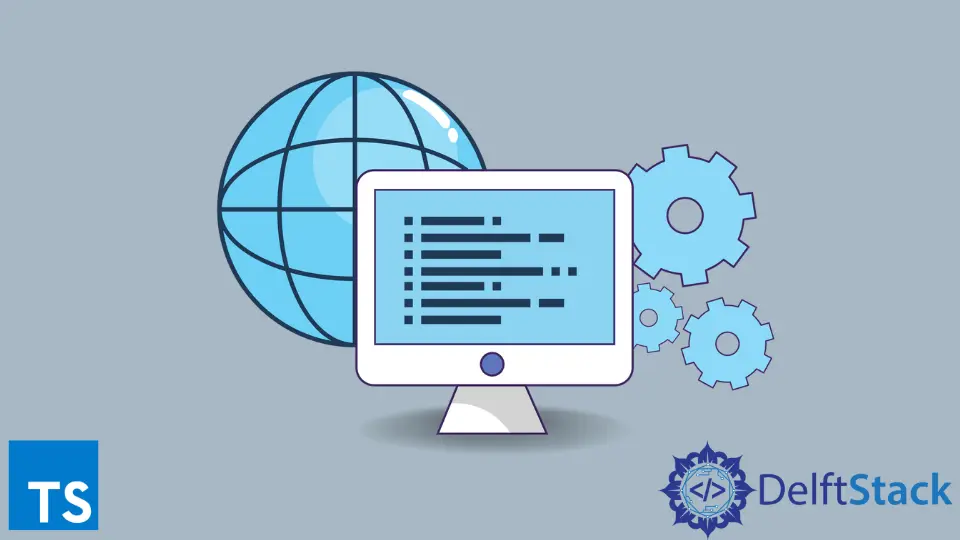
The TypeScript documentation defines a global library as modules that are accessed from the global scope, and we usually know we are using the global scope if we are not using the import
keyword to access its members.
The global libraries contain global variables
, interfaces
, namespaces
, and other declarations that other modules can access in the application. This tutorial will teach us how to create global variables in TypeScript and access them from other files.
Create a New Project in TypeScript
Open WebStorm IDEA
and select File
> New
> Project
. On the window that opens, select Node.js
on the left side and change the project name from untitled
to global-scope
on the Location
section or use any name preferred.
Ensure the node
runtime environment is installed before creating this project to ensure the Node interpreter
and Package manager
sections are automatically added from the file system.
Finally, press the Create
button to generate the project. Once the project has been generated, open a new terminal window using the keyboard shortcut Alt+F12 and use the following command to create a tsconfig.json
file.
~/WebstormProjects/global-scope$ tsc --init
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"noEmitOnError": true,
"strict": true,
"noFallthroughCasesInSwitch": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true
}
}
Most of the configurations in this file are auto-generated, and we have added the noEmitOnError: true
property to ensure that the JavaScript files are not generated if there is a transpile error.
What Is a Global Object
Before we create global variables, we need to understand what a global object is as it plays a key role in this context. In a JavaScript environment, when global variables are created using the var
keyword, they are added to the global object.
In a Node.js
environment, the variables are added to the global object depending on the execution context. The different execution contexts available include the browser, the code is executed in a worker, or the code being executed by Node.js.
The object for each of these contexts includes Window
, Worker
, and global
.
To access the global object without considering the execution context, we will use a property named globalThis
. The globalThis
property will help us access the properties added to the global scope.
Note that any variable declared using let
or const
is not added to the global scope, and any variable declared using the var
keyword is added to the global scope.
Declare Global Variables in TypeScript
Create a file named module.d.ts
under the package global-scope
and copy and paste the following code into the file.
declare var userName: string
declare var age: number
When we want to create a module for the global variables, we must do so inside a file with the extension .d.ts
as we have done in this example.
In this code, we have created two global variables named userName
and age
. Note that we have prefixed the variable declarations with the keyword declare
.
We must ensure that the global variables declared at the top level are prefixed with the declare
keyword since it is a convention for any variable declared in this file.
Access Global Variables in TypeScript
Create a file named main.ts
under the package global-scope
and copy and paste the following code into the file.
globalThis.userName = "john doe"
globalThis.age = 34
function main(){
console.log(userName);
console.log(age);
}
main();
In this code, we have used the globalThis
property to access the global variables userName
and age
from the global object and initialized each global variable with values for their type.
The main()
function logs the values for each global variable to the console. Run this code using the following command to verify our code is working as expected.
~/WebstormProjects/global-scope$ tsc && node main.js
The tsc
command transpile the TypeScript code to JavaScript code, and the node
command executes the file named main.js
. Ensure the output is as shown below.
john doe
34
Conclusion
This tutorial taught us how to create global variables in TypeScript. The topics covered in this tutorial include what a global object is, how to declare global variables, and how to access global variables.
Note that not only variables can be declared in the .d.ts
file. We can declare interface, class, function, and others.
If we want all of these details enclosed in a variable, we can use a namespace
which will also work as expected.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub