How to Cast a JSON Object to a Class in TypeScript
-
Use
Object.assign
to Cast From JSON to Class in TypeScript - Use Custom Methods to Cast a JSON String to Class in TypeScript
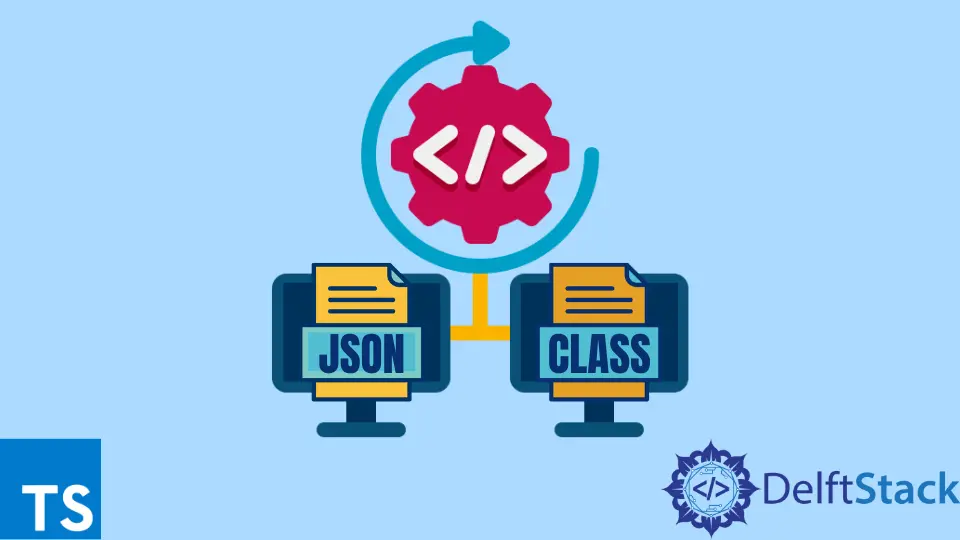
The data on the internet flows in the form of strings and can be converted to a very popular format called JSON. This JSON representation of the data often represents an object or even a class in TypeScript.
TypeScript provides the functionality to cast an object to a class. This article will discuss how to transform the received object into a TypeScript class to make type support, IDE completions, and other features accessible.
Use Object.assign
to Cast From JSON to Class in TypeScript
The Object.assign
method gives an easy way of converting JSON objects to a TypeScript class so that the methods associated with the class also become accessible.
The following code segment shows how a JSON object can be cast to a TypeScript class using the Object.assign
method.
Code:
class Animal{
name : string;
legs : number;
eyes : number;
constructor(name? : string, legs? : number, eyes? : number){
this.name = name ?? 'Default name';
this.legs = legs ?? 0;
this.eyes = eyes ?? 0;
}
getName(){
return this.name;
}
getEyes(){
return this.eyes;
}
}
var jsonString : string = `{
"name" : "Tiger",
"legs" : 4,
"eyes" : 2
}`
var animalObj = new Animal();
console.log(animalObj.getEyes());
Object.assign(animalObj, JSON.parse(jsonString));
console.log(animalObj.getEyes());
Output:
0
2
In the above example, the getEyes()
method returned 0
, which was the default value, and when the parsed JSON object was assigned to the animalObj
object, it returned 2
, the value in the JSON string.
Use Custom Methods to Cast a JSON String to Class in TypeScript
One can have custom methods such as fromJSON
to cast a JSON object to the respective class in TypeScript. This method is more useful as it gives more power to the user.
Code:
class Animal{
name : string;
legs : number;
eyes: number;
constructor(name : string, legs : number, eyes: number){
this.name = name;
this.legs = legs;
this.eyes = eyes;
}
getName(){
return this.name;
}
toObject(){
return {
name : this.name,
legs : this.legs.toString(),
eyes : this.eyes.toString()
}
}
serialize() {
return JSON.stringify(this.toObject());
}
static fromJSON(serialized : string) : Animal {
const animal : ReturnType<Animal["toObject"]> = JSON.parse(serialized);
return new Animal(
animal.name,
animal.legs,
animal.eyes
)
}
}
var jsonString : string = `{
"name" : "Tiger",
"legs" : 4,
"eyes" : 2
}`
var animalObj : Animal = Animal.fromJSON(jsonString);
console.log(animalObj)
// this will work now
console.log(animalObj.getName());
Output:
Animal: {
"name": "Tiger",
"legs": 4,
"eyes": 2
}
"Tiger"