How to Use Axios in TypeScript
- Use Axios in TypeScript
- Types in the Axios Library
- Use Axios to Make REST API Calls in TypeScript
-
Create an Axios
Config
File in TypeScript
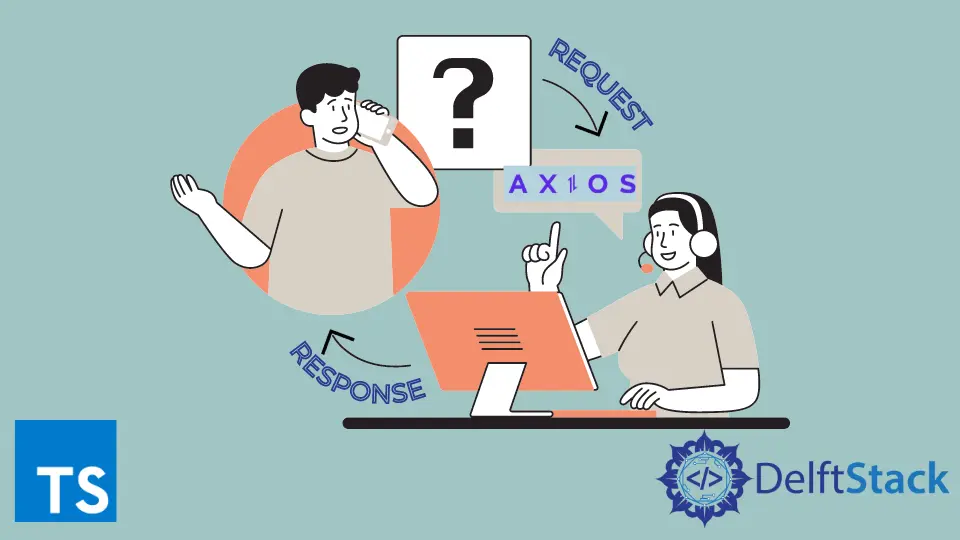
Axios is a prevalent JavaScript library for managing making requests to a backend resource. With the growing demand for TypeScript, types have been added to the Axios library.
This tutorial will use Axios to make REST API calls in TypeScript.
Use Axios in TypeScript
The first step is to install Axios in a project. Axios can be installed in a NodeJs or React project.
npm install axios
// or
yarn install axios
Now, Axios can be used in the project with other packages.
Types in the Axios Library
Several pre-built types are available in the Axios Github repository. This tutorial will focus on some of the important types in Axios.
export interface AxiosResponse<T = never> {
data: T;
status: number;
statusText: string;
headers: Record<string, string>;
config: AxiosRequestConfig<T>;
request?: any;
}
The AxiosResponse
is the response object returned as a Promise due to a REST API call such as GET
or POST
.
get<T = never, R = AxiosResponse<T>>(url: string, config?: AxiosRequestConfig<T>): Promise<R>;
post<T = never, R = AxiosResponse<T>>(url: string, data?: T, config?: AxiosRequestConfig<T>): Promise<R>;
The above code shows two REST API methods in Axios and their types. The AxiosRequestConfig
is a pretty big interface defined here.
Some of the important fields in the interface are:
export interface AxiosRequestConfig<T = any> {
url?: string;
method?: Method;
baseURL?: string;
data?: T;
headers?: Record<string, string>;
params?: any;
...
}
Thus, one can either put the baseURL or the full URL as part of the request. One of the critical things to notice here is the data
field in AxiosRequestConfig
and AxiosResponse
, which are generic types T
and can accept any type.
Use Axios to Make REST API Calls in TypeScript
The above types can make typed REST API calls in TypeScript. Suppose an e-commerce website makes a REST API call to its backend to show all the books available on its frontend.
interface Book {
isbn : string;
name : string;
price : number;
author : string;
}
axios.get<Book[]>('/books', {
baseURL : 'https://ecom-backend-example/api/v1',
}).then( response => {
console.log(response.data);
console.log(response.status);
})
Using React, one can even store the returned data as part of the AxiosResponse
in a state with something like:
const [books, setBooks] = React.useState<Book[]>([]);
axios.get<Book[]>('/books', {
baseURL : 'https://ecom-backend-example/api/v1',
}).then( response => {
setBooks(response.data);
})
Create an Axios Config
File in TypeScript
Axios provides many useful types and can be used to create a config
file which we can further use to make REST API calls throughout the application.
interface Book {
isbn : string;
name : string;
price : number;
author : string;
}
const instance = axios.create({
baseURL: 'https://ecom-backend-example/api/v1',
timeout: 15000,
});
const responseBody = (response: AxiosResponse) => response.data;
const bookRequests = {
get: (url: string) => instance.get<Book>(url).then(responseBody),
post: (url: string, body: Book) => instance.post<Book>(url, body).then(responseBody),
delete: (url: string) => instance.delete<Book>(url).then(responseBody),
};
export const Books {
getBooks : () : Promise<Book[]> => bookRequests.get('/books'),
getSingleBook : (isbn : string) : Promise<Book> => bookRequests.get(`/books/${isbn}`),
addBook : (book : Book) : Promise<Book> => bookRequests.post(`/books`, book),
deleteBook : (isbn : string) : Promise<Book> => bookRequests.delete(`/books/${isbn}`)
}
Thus, throughout the application, the config
can be used as:
import Books from './api' // config added in api.ts file
const [books, setBooks] = React.useState<Book[]>([]);
Books.getPosts()
.then((data) => {
setBooks(data);
})
.catch((err) => {
console.log(err);
});
Thus, Axios enables us to make clean and strongly typed implementations of REST API calls.