How to Convert Tensor Into NumPy Array in TensorFlow
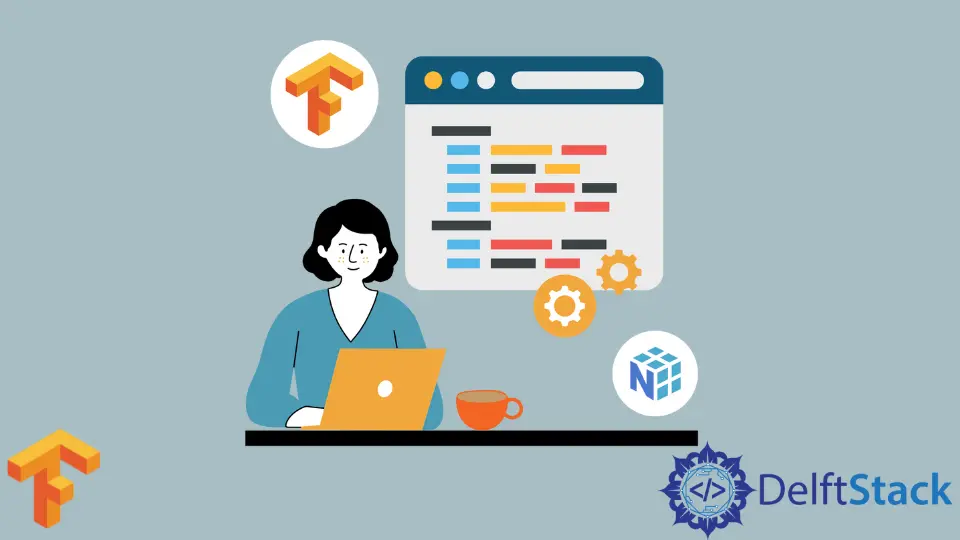
This article demonstrates the ways to convert tensors to NumPy array in TensorFlow. The compatibility of NumPy with TensorFlow is excellent, and we can easily convert a tensor to a NumPy array.
This article will show two methods to convert tensors to NumPy array.
TensorFlow Tensors vs. NumPy Arrays
An Array is a data structure used to store a collection of elements. To support the faster numerical operation associated with arrays, NumPy and TensorFlow are handy libraries, and one can easily use them in Python.
The NumPy library is mainly used for classical machine learning tasks, while TensorFlow supports deep learning computations very well. One significant benefit of a tensor over an array is that the GPUs are better at processing tensors than the primitive arrays.
Convert Tensor Into NumPy Array in TensorFlow
TensorFlow supports automatic conversion of the NumPy array during implementation. You can see the below code for automatic conversion.
!pip install tensorflow==2.9.1
import numpy as np
import tensorflow as tf
ndarray = np.ones([3, 3])
print("TensorFlow operations convert numpy arrays to Tensors automatically")
tensor = tf.math.multiply(ndarray, 42)
print(tensor)
In the above code, ndarray
is a NumPy array, and tf.math.multiply(tensor1, tensor2)
takes it as an argument and converts it to a tensor automatically before multiplication with the other tensor (i.e., 42). Note that tensor1
and tensor
must be of the same type.
Now, we will see an explicit conversion method. To be called on a tensor, you must use the .numpy()
function.
# Convert tensor to numpy array explicitly.
a = tf.constant([[1, 2], [3, 4]])
b = a.numpy()
print(type(b))
In the above code, a
is a constant tensor upon which we call the built-in .numpy()
function, which converts tensor a
into a NumPy array.
The above gives the output as:
numpy.ndarray
In TensorFlow, eager execution is an environment that supports fast execution of the different operations of tensors without building visual graphs behind the scene.
By default, eager execution in TensorFlow is enabled. But if you make it disabled during implementation, you can not convert the tensor to a NumPy array.
In that case, you see the below code;
tf.compat.v1.disable_eager_execution() # need to disable eager in TF2.9.1
# Multiplication
a = tf.constant([1, 2])
b = tf.constant(6)
c = a * b
sess = tf.compat.v1.Session()
# Evaluate the tensor c.
print(type(sess.run(c)))
You can visit this page to check more inter-related operations between TensorFlow and NumPy.