SQLite Equivalent to ISNULL(), NVL(), IFNULL(), or COALESCE()
-
Fetch Records With
NULL
Values in the SQLite Database -
Use
THEN
andELSE
to Check forNULL
Values in the SQLite Database -
Use the
COALESCE()
Function in the SQLite Database -
Use the
NVL()
Function in the SQLite Database -
Difference Between the
COALESCE()
and theifnull()
Functions -
Difference Between the
COALESCE()
and theisnull()
Functions
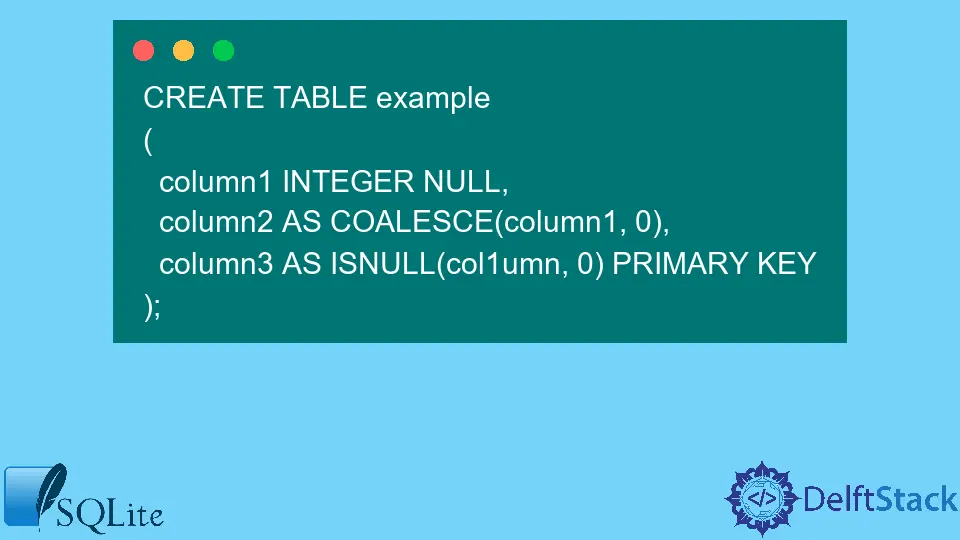
SQLite is used to create, read, update and delete records from the database. The database contains different tables for maintaining the data.
Each table consists of rows and columns, which carry the record. These records often have empty or null values, which cause issues or errors while retrieving the data.
Fetch Records With NULL
Values in the SQLite Database
To fetch the records from the SQLite database, we use the SELECT
clause in the SQLite statement. The SELECT
statement also fetches and ignores the NULL
values and fetches the record with value only.
SELECT field
FROM table
WHERE field = aCondition
A problem arises when there are empty values inside the table. The SELECT
statements need the NULL
check to record or fill the empty values with a specified text to accommodate the records with empty values.
SELECT field, [isnull](field, '')
FROM table
WHERE field = aCondition
In the above statement, the NULL
values may not be able to recognize the isnull()
function. There is a similar function to the isnull()
function, the ifnull()
function, to address this issue.
The full syntax of the ifnull()
function is as follows.
Syntax of the ifnull()
function:
ifnull(column, alternateValue)
Parameters:
column |
The target column in the SQLite database. |
alternateValue |
The selected value if the return value is null or empty. |
In the below statements, we use the ifnull()
function with the SELECT
statement. The first statement removes all the NULL
values from the record and replaces them with an empty string.
You can add any value as a replacement for the NULL
values in the database records. This can be shown in the second statement, where a NULL
value is replaced by a This is a NULL
string value in the SQLite database.
The ifnull()
function takes exactly the two arguments and returns the first non-empty string or text or NULL
if both the provided arguments are NULL
.
SELECT ifnull(NameofColumn,'')
SELECT ifnull(NULL,'This is a NULL');
Use THEN
and ELSE
to Check for NULL
Values in the SQLite Database
Alternatively, if the first scenario is unsuitable for your condition, we can use the conditional statement IS NULL
to check the empty values inside the table.
The SQLite statement below is equivalent to the ISNULL(fieldName, 0)
command. The IS NULL
clause will return all the empty values inside the database.
If any value is found, THEN
replaces the empty with a 0
value, ELSE
if something is found (not empty), then the value remains unchanged.
SELECT fieldName FROM NameOfTable WHERE fieldName IS NULL THEN 0 ELSE fieldName END
Use the COALESCE()
Function in the SQLite Database
Similar to the ifnull()
function, the COALESCE()
function provides an alternate value to the column value where the given value is NULL
. To write the SQLite statement to check the null value with the COALESCE()
function, we write the statement for the given table.
The function COALESCE()
works in SQLite version 3.8.6 and above.
id | Quantity |
---|---|
1 | 200 |
2 | 13 |
3 | NULL |
4 | 30 |
5 | 512 |
SELECT COALESCE(Quantity, 0) AS Inventory
FROM Product;
Updated Table Output:
id | Quantity |
---|---|
1 | 200 |
2 | 13 |
3 | 0 |
4 | 30 |
5 | 512 |
Use the NVL()
Function in the SQLite Database
Similar to the COALESCE()
function, the NVL()
function does the same. It checks for the empty values inside the database and replaces them with an alternate value as specified in the NVL()
function.
The difference is that the isnull()
function has been replaced with the NVL()
function, an Oracle function in the SQL server.
Difference Between the COALESCE()
and the ifnull()
Functions
The main difference between the COALESCE()
and ifnull()
functions is that the ifnull()
function takes only two arguments. It checks whether the first argument is NULL
or not, and if it is NULL
, it replaces it with the second argument.
Conversely, the function COALESCE()
takes two or more parameters and checks whether the first argument is NULL
. If the first argument is NULL
, it checks for the second.
If the second argument is NULL
, it keeps checking the next argument until it finds a non-empty value and replaces it with the first NULL
argument.
SELECT IFNULL('any value', 'extra value');
SELECT IFNULL(NULL,'extra value');
SELECT COALESCE(NULL, 'extra value');
SELECT COALESCE(NULL, 'any value', 'extra value');
SELECT COALESCE(NULL, NULL, NULL, NULL, 'the non-null value');
Output:
any value
extra value
extra value
some value
the non-null value
Difference Between the COALESCE()
and the isnull()
Functions
Mainly, both functions do the same functionality, checking for the NULL
values and replacing them with the specified non-empty value. However, they have a difference in their behavior.
- The difference is that the
isnull()
function evaluates only once, but theCOALESCE()
function evaluates multiple times. - Another difference is that in determining the data type, the function
isnull()
uses the first argument data type, whereas theCOALESCE()
function uses theCASE
expression rule and takes the data type of the highest precedence. - The last difference is that the function
isnull()
never returns aNULL
value, and we always assume that the result of this function is non-Nullable. While theCOALESCE()
function can return theNULL
expression.
Example 1:
CREATE TABLE example
(
column1 INTEGER NULL,
column2 AS COALESCE(column1, 0) PRIMARY KEY,
column3 AS ISNULL(column1, 0)
);
Output:
# the statement fails as the nullability of the COALESCE function evaluates to NULL
Error: PRIMARY KEY cannot accept NULL values
Example 2:
CREATE TABLE example
(
column1 INTEGER NULL,
column2 AS COALESCE(column1, 0),
column3 AS ISNULL(col1umn, 0) PRIMARY KEY
);
Output:
# the above statement works as the nullability of the ISNULL function evaluates as NOT NULL.
No error
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn