File Extensions for SQLite Database Files
- SQLite Database File Extensions
- Tools for Accessing or Modifying the SQLite Database Extension
- View SQLite Database Using Android
- Create an SQLite Database File With an Extension
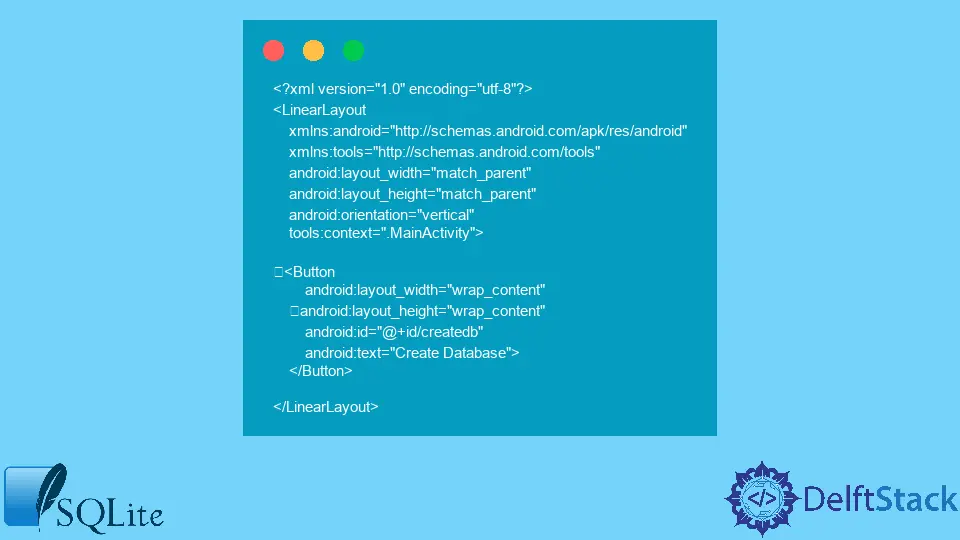
In an android operating system, we use the SQLite database to store the data locally. SQLite is an open-source database in which we can create, read, update and delete user data locally on the phone. This database is available any time you want to use the database. In SQLite, the data is stored in the form of tables. These tables look similar to the excel sheet style. On a local device, the files are stored in different file extensions on the phone.
SQLite Database File Extensions
There is no requirement for using a specific file extension for SQLite databases. We can use whatever file extension we want; it is the developer’s personal choice to use whatever extension they want.
Mostly, the developers use file extensions similar to the database schema; for example, we use database schema as a file extension or format. So, if we store the logs of files, we store them with a .logs
extension or .index
for storing the index information.
Similarly, if a developer wants to use a general file extension, then the most common name, .sqlite3
, is used. It shows the version of SQLite used with the database.
Scenario Discussion for SQLite File Extension
Suppose a company develops distributable software that does not allow customers to interact or do anything with the database. Then, there is a reason to name the database file extension with .db
instead of anything because the user may want to interact with the database to make a backup copy of the database.
In this case, if the file extension is .sqlite
or .anything
, the user might be able to change or mess up the database, which may cause the database to be corrupt, so it depends on the scenario in which type of file extension is used for the SQLite database.
Tools for Accessing or Modifying the SQLite Database Extension
For standard SQLite file extensions, SQLiteStudio
uses different file formats to access or modify the data. This file format includes db|sdb|sqlite|db3|s3db|sqlite3|sl3|db2|s2db|sqlite2|sl2
) to be used by default for development and deployment purposes.
So, a specific format for SQLite database is needed if we use a tool. Whatever tool you use, let the tool decide the file extension for the SQLite database.
If we open the SQLite database file on an android phone, it does not matter which file extension is used. The android phone will locate the database, and the user can easily view it without the hassle of the correct file extension.
The difference shows when we copy or move the database file on any drive to view the SQLite database on the operating system, then the tool will need to know the proper file extension. If the database has no file extension or even an incorrect file extension, then the tool won’t be able to open the database file.
So, if you are using a tool like SQLite studio, then the tool won’t be able to identify the database file without the .db
extension.
When we create an SQLite database from an android application, it creates the database file on the phone without the worry of file extension as the database header format contains information like SQLite format 3\000
to show that it is an SQLite database file. We only need the SQLite database format for humans or some operating system to recognize the file correctly.
View SQLite Database Using Android
To start, we need to create a project using an android studio or open a project in android studio, which already has an SQLite database connection. The SQLite database should have some records inside it.
Next is to connect an android device to the android studio by turning on the debugging mode in the developer settings on the phone. Once the device is successfully connected, the android studio will show the phone’s name on the editor.
Then on the bottom right-hand side of the android studio, click on the Device File Explorer
. Inside the explorer, search for your application package name.
Inside the package name, you can see the database file with the .sqlite
extension. To view the SQLite database file, you need to download the file and view it on the SQLite browser.
Create an SQLite Database File With an Extension
Create .xml
and .java
source files to create layout and functionality files, respectively.
main_activity.xml
File:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/createdb"
android:text="Create Database">
</Button>
</LinearLayout>
main_Activity.java
File:
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private Button createDB;
private DBHandler dbHandler;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_activity);
createDB = findViewById(R.id.createdb);
dbHandler = new DBHandler(MainActivity.this);
createDB.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dbHandler.addNewEntry("Hello World");
Toast
.makeText(MainActivity.this, "A new text has been added to the SQLite database",
Toast.LENGTH_SHORT)
.show();
}
});
}
}
DBHandler.java
File:
import android.content.ContentValues;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DBHandler extends SQLiteOpenHelper {
private static final String DB_NAME = "newdb";
private static final int DB_VERSION = 1;
private static final String TABLE_NAME = "mytable";
private static final String ID_COL = "id";
private static final String WORD = "word";
public DBHandler(Context context) {
super(context, DB_NAME, null, DB_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String query = "CREATE TABLE " + TABLE_NAME + " (" + ID_COL
+ " INTEGER PRIMARY KEY AUTOINCREMENT, " + WORD + " TEXT)";
db.execSQL(query);
}
// this method is used to add a new course to our sqlite database.
public void addNewCourse(String myword) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(WORD, myword);
db.insert(TABLE_NAME, null, values);
db.close();
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn