How to Do Case-Insensitive String Comparison in Sqlite3
- Implement Case-Insensitive String Comparison in Sqlite3
-
Use the
COLLATE NOCASE
in theCREATE
Query for Case-Insensitive String Comparison in Sqlite3 -
Use the
COLLATE NOCASE
in theSELECT
Query for Case-Insensitive String Comparison in Sqlite3 -
Use of
LIKE
Operator for Case-Insensitive String Comparison in Sqlite3 -
Use the
LOWER()
orUPPER()
Functions for Case-Insensitive String Comparison in Sqlite3
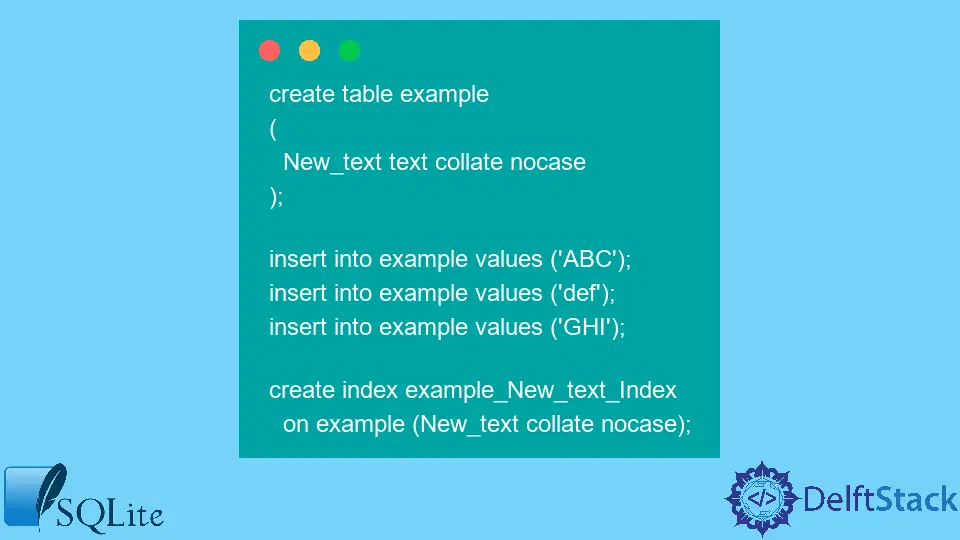
SQLite database engine is developed in C language. It is not an application that can stand alone; rather, it’s a library that the application developers can use to implement their applications.
Like MySQL, SQLite is also a relational database management system (RDBMS) and is implemented on SQL (structured query language). In version 3 of SQLite, the database is case-sensitive when string matching, so when a user selects a record from a database using the =
(single equal) operator in the where
clause, the SQLite database shows that it is case-sensitive.
Implement Case-Insensitive String Comparison in Sqlite3
We have different ways to work with the SQLite database to tackle such a situation:
- We often use the
collate nocase
clause inCREATE
orSELECT
query statements. - Another option is to use the
LIKE
operator, which is case-insensitive when comparing the string operands. - Lastly, we use the
UPPER()
andLOWER()
functions on both sides of the operands when comparing a string with the=
operator.
Use the COLLATE NOCASE
in the CREATE
Query for Case-Insensitive String Comparison in Sqlite3
Suppose we have a database with different records added with the text (string) as a data type. Now, we want to select a few records from the table using the =
operator, and then the database shows that the records are case-sensitive.
So, generally, in SQLite, you can mention that a column should be case insensitive when you create a table with a collate nocase
query. We can also specify collate nocase
with an id or index value in the table.
In the below example, the example.New_text
is not case-sensitive.
create table example
(
New_text text collate nocase
);
insert into example values ('ABC');
insert into example values ('def');
insert into example values ('GHI');
create index example_New_text_Index
on example (New_text collate nocase);
Once we create and insert a few records in the table example
, we can test its case sensitivity by retrieving the records in a select
query. The below results show us that the collate nocase
works fine whenever we need case insensitivity for comparing the string data types in the where
clause.
Example 1:
SELECT New_Text FROM example WHERE New_Text = 'DEF';
Output:
def
Example 1 results in a def
value when we use the =
operator in the where
clause without taking care of the case-sensitive values inside the table. The reason is that the use of collate nocase
at the time of table creation makes the values of column New_text
case insensitive.
Example 2:
SELECT New_Text FROM example ORDER BY New_Text;
Output:
ABC
def
GHI
Example 3:
SELECT New_Text FROM example ORDER BY New_Text DESC;
Output:
GHI
def
ABC
Use the EXPLAIN
Command in the SQLite Database
We can also check for case-sensitive matching and searching using an index on the column. We use the EXPLAIN
command for this purpose.
EXPLAIN SELECT New_Text FROM example WHERE New_Text = 'def';
Output:
addr opcode p1 p2
1 Integer 0 0
2 OpenRead 1 3
3 SetNumColumns 1 2
4 String8 0 0
5 IsNull -1 14
6 MakeRecord 1 0
7 MemStore 0 0
8 MoveGe 1 14
9 MemLoad 0 0
10 IdxGE 1 14
11 Column 1 0
12 Callback 1 0
13 Next 1 9
14 Close 1 0
15 Halt 0 0
16 Transaction 0 0
17 VerifyCookie 0 4
18 Goto 0 1
19 Noop 0 0
Use the COLLATE NOCASE
in the SELECT
Query for Case-Insensitive String Comparison in Sqlite3
Suppose we create a table without considering the case sensitivity issue and not using the collate nocase
clause. Then, we can still use the collate nocase
clause while retrieving the records with the SELECT
query.
In this example, we can see that the collate nocase
clause can be used with the SELECT
statement in SQLite.
SELECT * FROM NameOfTheTable WHERE value = 'MatchingValue' COLLATE NOCASE
Use of LIKE
Operator for Case-Insensitive String Comparison in Sqlite3
Similar to the collate nocase
clause, we can use the LIKE
operator for the case-insensitive comparison of the string type in the SQLite database.
The LIKE
operator is a pattern-matching operator. As a usual operator, there are left and right-hand side operands for comparison.
The left-hand side operands contain the matching string, while the right-hand side operand contains the pattern to match with the matching string. This operator also contains the percent symbol %
for any number of sequences for a given character.
The LIKE
operator is also case insensitive, so it does not matter if lower or upper case letters are used for matching; it only has case sensitivity with the Unicode characters, not for ASCII code characters.
For example, in the LIKE
operator, the ASCII characters A
and a
are the same, but the Unicode characters Æ
and æ
are different. We use the LIKE
operator to compare the case-insensitive records in the SQLite database.
SELECT * FROM NameOFTheTable WHERE Value LIKE 'something'
Use the LOWER()
or UPPER()
Functions for Case-Insensitive String Comparison in Sqlite3
Suppose we have records in a particular column with lower and upper case values. However, when we compare the values using the =
operator in the SELECT
statement, we have a case-sensitive issue in the result.
To address this issue, we can implement SQLite functions LOWER()
and UPPER()
, where the LOWER()
function is lowercase for all the characters and the UPPER()
function is uppercase for all the characters of the provided string. We use these functions in the SELECT
statement to make them effective.
The example below demonstrates that the matching string first converts into the lower case characters using the LOWER()
function and the upper case character if we use the UPPER()
function.
Using the UPPER()
function in SQLite:
SELECT * FROM NameOFTheTable WHERE UPPER(value) = UPPER('something')
Using the LOWER()
function in SQLite:
SELECT * FROM NameOFTheTable WHERE LOWER(value) = LOWER('something')
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn