How to Save Seaborn Figure in Python
- Method 1: Saving a Seaborn Figure as a PNG File
- Method 2: Saving a Seaborn Figure as a JPEG File
- Method 3: Saving a Seaborn Figure as a PDF File
- Conclusion
- FAQ
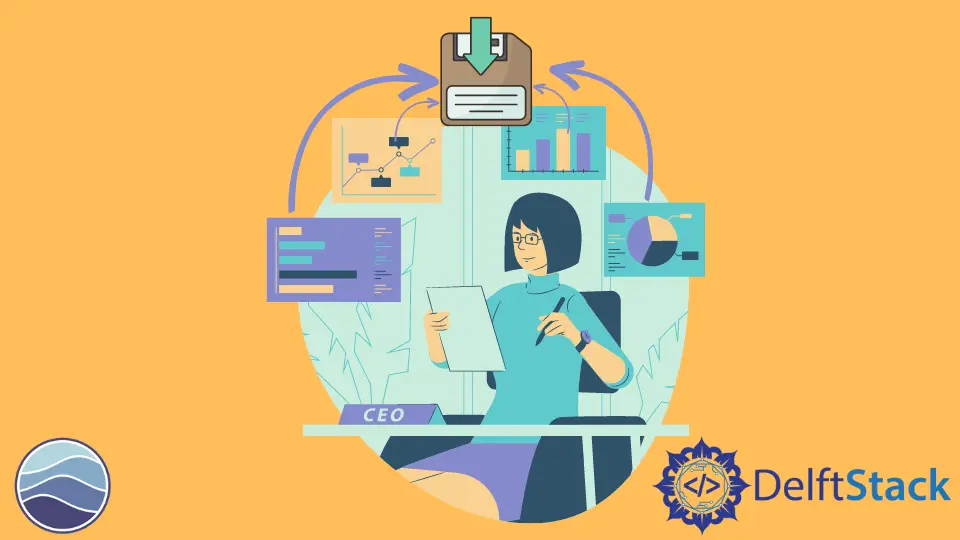
Creating stunning visualizations with Seaborn is just one part of the data visualization journey. Once you’ve crafted the perfect figure, the next logical step is to save it for future use or sharing.
In this tutorial, we’ll explore how to save a Seaborn figure in various formats using Python. Whether you’re looking to save your plot as a PNG, JPEG, or PDF, we’ve got you covered. By the end of this guide, you’ll be able to save your visualizations effortlessly, ensuring that your hard work doesn’t go to waste.
Method 1: Saving a Seaborn Figure as a PNG File
Saving a Seaborn figure as a PNG file is a straightforward process. The PNG format is widely used due to its lossless compression and support for transparency. To save your Seaborn figure in this format, you can use the savefig()
function provided by Matplotlib, which is the underlying library used by Seaborn.
Here’s how you can do it:
import seaborn as sns
import matplotlib.pyplot as plt
# Create a simple Seaborn plot
tips = sns.load_dataset("tips")
sns.scatterplot(data=tips, x="total_bill", y="tip")
# Save the figure as a PNG file
plt.savefig("seaborn_plot.png")
In this example, we start by importing the necessary libraries: Seaborn and Matplotlib. We then load the “tips” dataset and create a scatter plot. The crucial part comes with the plt.savefig()
function, which saves the figure to a file named “seaborn_plot.png”. This method is highly effective for preserving the quality of your visualizations, and PNG files are great for web use due to their smaller size.
Method 2: Saving a Seaborn Figure as a JPEG File
If you’re looking to save your Seaborn figure in a JPEG format, the process is quite similar to saving it as a PNG file. JPEG is a popular format for photographs and complex images, making it a good choice for visuals that contain gradients and colors.
Here’s how to save a Seaborn figure as a JPEG file:
import seaborn as sns
import matplotlib.pyplot as plt
# Create a simple Seaborn plot
tips = sns.load_dataset("tips")
sns.boxplot(data=tips, x="day", y="total_bill")
# Save the figure as a JPEG file
plt.savefig("seaborn_plot.jpg", quality=95)
In this code snippet, we again import Seaborn and Matplotlib, load the “tips” dataset, and create a box plot. The plt.savefig()
function is used here as well, but with an additional parameter: quality=95
. This parameter adjusts the quality of the saved JPEG file, with 100 being the highest quality. Saving figures as JPEG files is particularly useful when dealing with colorful plots, as it often results in smaller file sizes compared to PNG.
Method 3: Saving a Seaborn Figure as a PDF File
Saving your Seaborn figure as a PDF file is an excellent option if you need high-quality prints or want to preserve vector graphics. PDF files maintain the quality of your visualizations regardless of how much you zoom in, making them ideal for presentations and publications.
Here’s how to save a Seaborn figure as a PDF file:
import seaborn as sns
import matplotlib.pyplot as plt
# Create a simple Seaborn plot
tips = sns.load_dataset("tips")
sns.histplot(data=tips, x="total_bill", bins=30)
# Save the figure as a PDF file
plt.savefig("seaborn_plot.pdf")
In this example, we create a histogram using the “tips” dataset. The plt.savefig()
function is called with the filename “seaborn_plot.pdf”. The PDF format is particularly advantageous for academic purposes, as it retains the vector quality of the plot, ensuring that your figures look sharp and professional in any printed format.
Conclusion
In this tutorial, we’ve explored how to save Seaborn figures in Python using various formats, including PNG, JPEG, and PDF. Each format has its unique advantages, whether you’re sharing your visualizations online or preparing them for print. By utilizing the savefig()
function from Matplotlib, you can ensure that your hard work is preserved and easily accessible. Now that you know how to save your Seaborn figures, you can focus more on creating stunning visualizations and less on the logistics of storing them.
FAQ
-
What is the best format for saving Seaborn figures?
The best format depends on your needs. PNG is great for web use, JPEG is suitable for photographs, and PDF is ideal for high-quality prints. -
Can I specify the size of the saved figure?
Yes, you can specify the figure size using thefigsize
parameter in theplt.figure()
function before creating your plot. -
How can I adjust the resolution of the saved figure?
You can adjust the resolution by using thedpi
parameter in thesavefig()
function. Higher DPI values result in better quality. -
Is it possible to save multiple figures at once?
Yes, you can save multiple figures by callingplt.savefig()
for each figure you create, ensuring to clear the current figure after each save. -
Can I save Seaborn figures without displaying them?
Yes, you can save figures without displaying them by not callingplt.show()
after creating your plot.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn