Equivalent of the Try-Catch Statement in Rust
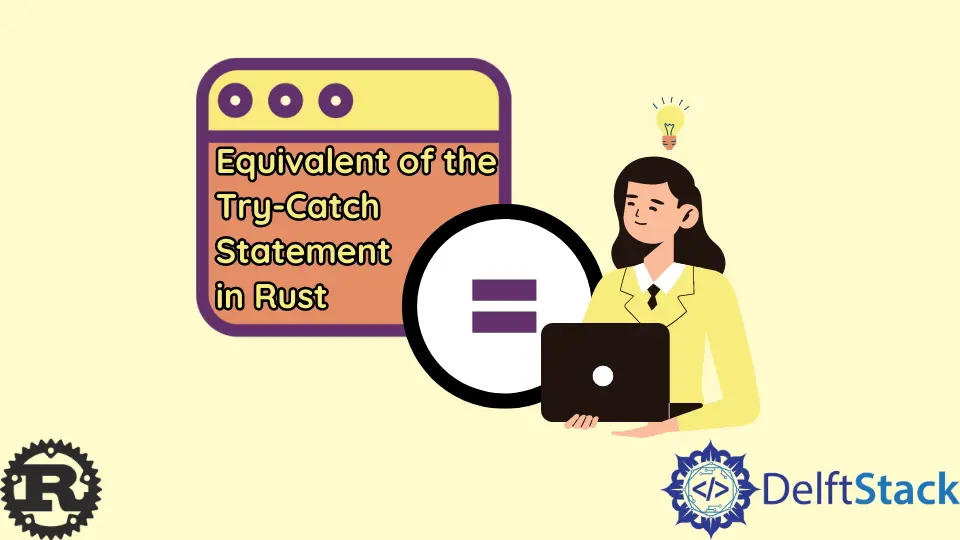
Rust is a programming language that provides a safer and more reliable way of developing software. It is designed to prevent common errors that lead to security vulnerabilities in other languages.
The Rust language does not support exception handling, which means it doesn’t have a try-catch
statement in Rust.
The lack of exception handling in Rust eliminates the possibility of runtime errors due to exceptions being thrown from a function. This is because the program will stop at the point where an error occurs instead of continuing and possibly causing other problems in the codebase.
This article will discuss the Rust equivalent to a try-catch
statement.
Equivalent of the try-catch
Statement in Rust
There are many equivalents available in Rust for try-catch
statements. But, here in this article, we will discuss only a few closest equivalents.
Use the ?
Operator in Rust
In Rust, we can use the ?
operator to return or not return the values.
The ?
operator is one of the essential features of Rust, and it can be used in many different ways. It can check if a value is present, if a value has been assigned to a variable, or if an expression evaluates to true or false.
The ?
operator can also be used as an alternative for Rust’s try-catch
statement. The ?
operator takes a closure as its parameter.
The closure can then be called without worries about what will happen if an error occurs during execution.
The ?
operator will catch the error and return the value of the closure if there was no error, or it will return an error type with a description of what went wrong if there was one.
Example:
fn main() {
let hello_tasks = || -> Result<(), HelloError> {
hello_task_1()?;
hello_task_2()?;
hello_task_3()?;
Ok(())
};
if let Err(_err) = hello_tasks() {
println!("There's an error in your code, please correct it");
}
}
enum HelloError {
HelloTask1Error,
HelloTask2Error,
HelloTask3Error,
}
fn hello_task_1() -> Result<(), HelloError> {
println!("Task No 1");
Ok(())
}
fn hello_task_2() -> Result<(), HelloError> {
println!("Task No 2");
Err(HelloError::HelloTask2Error)
}
fn hello_task_3() -> Result<(), HelloError> {
println!("task3");
Ok(())
}
Output:
Task No 1
Task No 2
There's an error in your code, please correct it
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook