How to Use the Rust MPSC
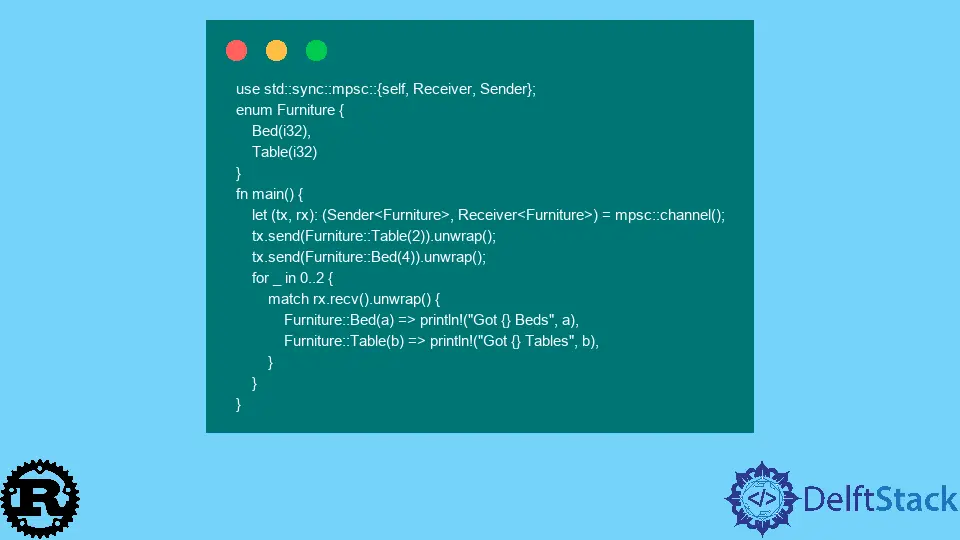
The Rust programming language is renowned for its performance and safety, especially in concurrent programming. One of the standout features in Rust’s concurrency toolkit is the MPSC (multiple producers, single consumer) channel. This powerful construct allows multiple threads to send messages to a single receiver, making it ideal for scenarios where you want to decouple producers from consumers.
In this article, we’ll explore how to use the Rust MPSC effectively, providing code examples and detailed explanations to help you understand its application. Whether you’re building a multi-threaded application or just want to learn more about Rust’s capabilities, this guide will equip you with the knowledge you need to leverage MPSC channels in your projects.
Understanding MPSC Channels
MPSC channels in Rust are designed to facilitate communication between multiple threads. The basic idea is that you have multiple producers (threads that send messages) and a single consumer (the thread that receives messages). This setup is beneficial in various scenarios, such as handling tasks or distributing workload among threads.
In Rust, MPSC channels are implemented using the std::sync::mpsc
module. The module provides the necessary tools to create channels, send messages, and receive them. The channel consists of a sender and a receiver. The sender is used by the producers to send data, while the receiver is used by the consumer to receive data.
Here’s a simple example to illustrate how to create and use an MPSC channel in Rust:
use std::sync::mpsc;
use std::thread;
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
let message = String::from("Hello from producer!");
tx.send(message).unwrap();
});
let received = rx.recv().unwrap();
println!("Received: {}", received);
}
In this code, we first create a channel using mpsc::channel()
, which gives us a transmitter (tx
) and a receiver (rx
). A new thread is spawned, which sends a message through the transmitter. The main thread then waits to receive this message and prints it out.
Output:
Received: Hello from producer!
This example demonstrates the basic mechanics of the MPSC channel, showcasing how easy it is to send and receive messages between threads in Rust.
Creating Multiple Producers
One of the key advantages of MPSC channels is the ability to have multiple producers sending messages to the same consumer. This feature is particularly useful in scenarios where you want to gather results from several sources or distribute work across threads.
To implement multiple producers, you can spawn several threads, each sending messages to the same channel. Here’s an example that illustrates this concept:
use std::sync::mpsc;
use std::thread;
use std::time::Duration;
fn main() {
let (tx, rx) = mpsc::channel();
for i in 0..5 {
let tx_clone = tx.clone();
thread::spawn(move || {
thread::sleep(Duration::from_secs(1));
let message = format!("Message from producer {}", i);
tx_clone.send(message).unwrap();
});
}
for _ in 0..5 {
let received = rx.recv().unwrap();
println!("Received: {}", received);
}
}
In this example, we create a loop that spawns five threads, each of which sends a message to the channel after a short delay. The tx.clone()
method is used to create a new sender for each thread. The consumer then receives messages in the order they arrive.
Output:
Received: Message from producer 0
Received: Message from producer 1
Received: Message from producer 2
Received: Message from producer 3
Received: Message from producer 4
This setup allows you to efficiently gather messages from multiple threads, demonstrating the flexibility of the MPSC channel in handling concurrent tasks.
Handling Message Types
Rust’s type system ensures that the messages sent through an MPSC channel are type-safe. You can send any type that implements the Send
trait, which includes most primitive types and user-defined types. However, it’s essential to ensure that both the sender and receiver agree on the message type.
Let’s create a custom message type and see how it can be sent through an MPSC channel:
use std::sync::mpsc;
use std::thread;
#[derive(Debug)]
struct CustomMessage {
id: u32,
content: String,
}
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
let message = CustomMessage {
id: 1,
content: String::from("Hello from custom producer!"),
};
tx.send(message).unwrap();
});
let received = rx.recv().unwrap();
println!("Received: {:?}", received);
}
In this code, we define a CustomMessage
struct with an id
and content
. The producer thread sends an instance of this struct through the channel. The consumer then receives it and prints its debug representation.
Output:
Received: CustomMessage { id: 1, content: "Hello from custom producer!" }
This example highlights the versatility of MPSC channels, allowing you to send complex data types while maintaining type safety.
Conclusion
The Rust MPSC channel is a powerful tool for managing concurrency in your applications. By enabling multiple producers to send messages to a single consumer, it simplifies the process of thread communication. Throughout this article, we’ve explored the basics of MPSC channels, how to implement multiple producers, and how to send custom message types. As you continue your journey with Rust, mastering MPSC channels will enhance your ability to build efficient and robust multi-threaded applications.
FAQ
-
What is an MPSC channel in Rust?
An MPSC channel allows multiple threads (producers) to send messages to a single thread (consumer) efficiently. -
How do I create an MPSC channel in Rust?
You can create an MPSC channel usingstd::sync::mpsc::channel()
which returns a sender and a receiver. -
Can I send custom types through an MPSC channel?
Yes, as long as the type implements theSend
trait, you can send custom types through an MPSC channel. -
What happens if the receiver is not ready to receive messages?
If the receiver is not ready, the sender will block until the receiver is ready to receive the message. -
Is it possible to have multiple consumers with MPSC channels?
No, MPSC channels are designed for multiple producers and a single consumer. For multiple consumers, you would need to use a different approach.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook