Difference Between Mod and Use in Rust
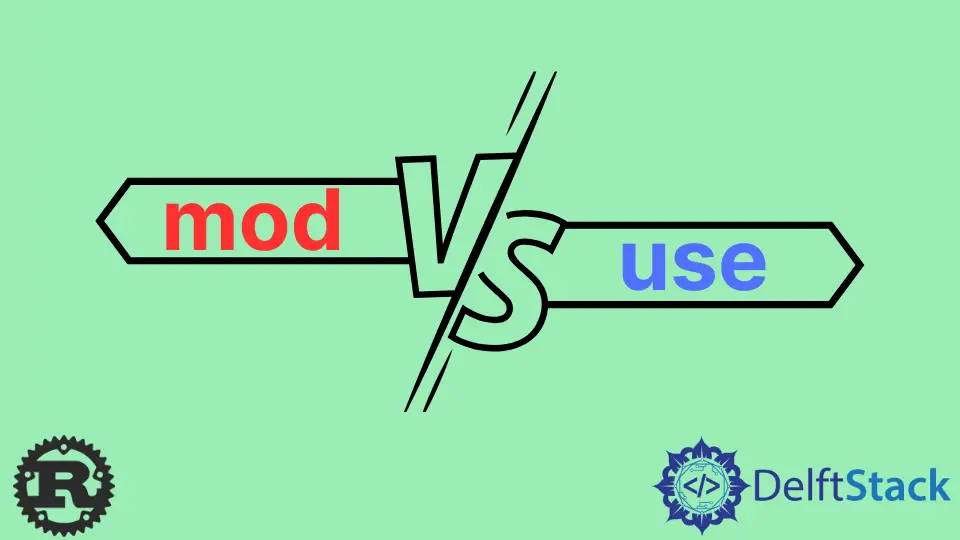
Rust is a modern and fast programming language that is designed to be a safe, concurrent, and functional system programming language. It was created by Mozilla in 2006.
Rust has three main concepts: ownership, borrowing, and lifetimes. Rust uses the two keywords: use
and mod
.
The keyword use
is used to import the module’s contents into the current scope. This means that it will make all of the functions within the module available to call from this point onwards.
mod
, on the other hand, imports only a single item from another module into your current scope so it can be called or referenced as needed without worrying about anything else in that module being accessible from now onwards.
Concept of use
in Rust
By pursuing a different path, use
adds another item to the current namespace. An item is a generic object you need to access, such as a function, struct, or trait.
A route is a module hierarchy you must follow to reach it. The current namespace refers to bringing an object into the current file so you may access it as if it were local.
Features of use
in Rust
Rust features a fairly dynamic mechanism for users that makes it simple to introduce many objects into the existing namespace with minimal effort.
- You may use the
self
keyword to introduce the universal parent module and any other things you want to avail into the namespace. - To avoid identity issues, use the
as
keyword to change things. - You may use the glob-like syntax to bring several objects into the current namespace:
use std::path::{self, Path, PathBuf};
Concept of mod
in Rust
Modules allow you to organize your code into separate files. They divide your code into logical pieces that can be imported and used in other modules or programs.
In short, mod
is used to specify modules and submodules so that you may use them in your current .rs
file, which might be complicated. Why not just use it?
A module in Rust is nothing more than a container for zero or more things. It’s a method of logically organizing items so your module can easily traverse.
Modules may be used to create a crate’s tree structure, enabling you to split your work across several files arbitrarily deep if necessary. A single .rs
file can include several modules, or a single file can contain multiple modules.
Lastly, you may use mod
to group objects logically. You may construct mod
blocks in a single .rs
file, or you can divide your source code across multiple .rs
files, use mod
, and let Cargo generate the tree structure of your crate.
Basic Difference Between mod
and use
in Rust
The primary difference between them is that use
imports a module from an external library, while mod
creates an internal module that can only be used within the current file.
Let’s discuss an example using the use
keyword.
use hello::rota::function as my_function;
fn function() {
println!("demo `function()`");
}
mod hello {
pub mod rota {
pub fn function() {
println!("demo `hello::rota`");
}
}
}
fn main() {
my_function();
println!("Coming");
{
use crate::hello::rota::function;
function();
println!("Returning");
}
function();
}
Output:
demo `hello::rota`
Coming
demo `hello::rota`
Returning
demo `function()`
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook