How to Declare Global Variables in Rust
-
Declare Global Variables in Rust Using the
static
Keyword -
Use the
lazy_static
Crate -
Use the
std::sync::RwLock
for Read-Write Global Variables -
Use the
Once
Primitive for Initialization - Conclusion
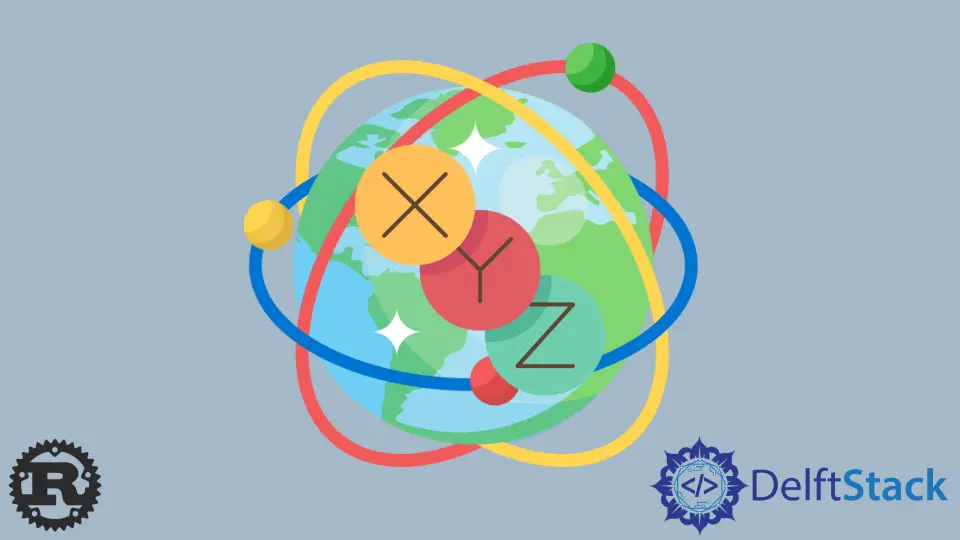
Rust, known for its emphasis on memory safety and performance, provides developers with two primary mechanisms for declaring global variables: const
and static
. The choice between these two keywords depends on the desired mutability of the global variable.
In this article, we will delve into the details of global variables in Rust and how to declare and use them effectively.
Global Variables in Rust
Global variables enable the storage of data with a wide scope, making it accessible across functions, modules, and even threads. In Rust, global variables offer a way to maintain values throughout the program’s execution.
However, they are stored in distinct memory locations depending on whether they are defined as const
or static
.
-
const
Variables: Declared with theconst
keyword, these global variables are immutable. Once assigned a value, they cannot be modified.The key advantage of
const
variables is their immutability, making them ideal for values that should not change during the program’s execution. -
static
Variables: On the other hand,static
global variables, declared using thestatic
keyword, are mutable. They allow developers to change their values throughout the program’s execution.This flexibility can be useful in scenarios where the global state needs to be altered dynamically.
It’s important to note that the let
keyword is not allowed in the global scope. Hence, for global variables in Rust, const
and static
are the only available options.
Global variables in Rust serve a critical role in memory management. They allow developers to maintain data on the stack during runtime while retaining a reference to data on the heap.
In the resulting machine code, there is a pointer to the heap saved on the stack.
These global variables are stored in the program’s data section and have fixed memory addresses that remain constant throughout program execution. This attribute allows the code segment to incorporate constant addresses and utilize them without consuming stack space.
In Rust, constants persist throughout the program’s entire lifetime. Unlike some other programming languages, Rust’s constants do not have a fixed memory location.
Instead, they are functionally inlined wherever they are used, which can optimize performance.
Static objects in Rust are akin to constants but with one significant distinction: they retain a single instance stored at a specific position in memory, making them ideal for scenarios where a shared, mutable global state is required.
Declare Global Variables in Rust Using the static
Keyword
The most straightforward way to declare global variables in Rust is by using the static
keyword. This creates a global, immutable variable that is accessible from any part of your program.
To declare a global variable in Rust, you use the static
keyword followed by the variable’s name, its type, and, optionally, its initial value. Here’s the basic syntax:
static GLOBAL_VARIABLE: Type = initial_value;
GLOBAL_VARIABLE
: The name of the global variable.Type
: The data type of the variable.initial_value
(optional): The initial value to assign to the variable.
Let’s look at a simple example:
static GLOBAL_COUNTER: i32 = 0;
fn main() {
println!("Global counter: {}", GLOBAL_COUNTER);
}
Output:
Global counter: 0
In this example, we declare a global variable named GLOBAL_COUNTER
of type i32
(32-bit signed integer) and initialize it to 0
. This variable is accessible from the main
function and can be used anywhere in the program.
By default, global variables declared with the static
keyword are immutable, meaning their values cannot be changed once initialized. Attempting to modify an immutable global variable will result in a compilation error.
static GLOBAL_VARIABLE: i32 = 42;
fn main() {
// Compilation error: cannot assign to an immutable static variable
GLOBAL_VARIABLE = 100;
}
If you need to declare a mutable global variable, you can use the mut
keyword to indicate that the variable’s value can be changed. Here’s an example:
static mut MUTABLE_GLOBAL_VARIABLE: i32 = 42;
fn main() {
unsafe {
MUTABLE_GLOBAL_VARIABLE = 100;
println!("Mutable global variable: {}", MUTABLE_GLOBAL_VARIABLE);
}
}
Output:
Mutable global variable: 100
In this case, we use static mut
to declare a mutable global variable, MUTABLE_GLOBAL_VARIABLE
, and we wrap any operations that modify or access it with unsafe
blocks.
Rust enforces strict safety rules, and using mutable global variables is considered unsafe because they can lead to data races in concurrent programs. The unsafe
block is required to indicate that you are aware of the potential issues and are handling them carefully.
Use the lazy_static
Crate
While the static
keyword in Rust is excellent for simple, immutable global variables, it might not be the best choice when dealing with complex or mutable global states. To handle such situations, you can leverage the lazy_static
crate, a powerful tool that allows you to create lazy-initialized global variables.
Lazy initialization is a technique where a variable is created and initialized only when it is first accessed. This approach is particularly valuable when working with mutable global variables.
By using lazy_static
, you can ensure that your mutable global state is set up efficiently and safely.
To begin using lazy_static
, you need to add it as a dependency in your Rust project’s Cargo.toml
file. Here’s how to do that:
[dependencies]
lazy_static = "1.4"
Now, let’s delve into a practical example of how to use lazy_static
to declare a mutable global variable.
We’ll declare a mutable global variable named MUTABLE_GLOBAL_VARIABLE
using lazy_static
. The variable will be of type Mutex<i32
, which provides safe concurrent access.
use lazy_static::lazy_static;
use std::sync::Mutex;
lazy_static! {
static ref MUTABLE_GLOBAL_VARIABLE: Mutex<i32> = Mutex::new(42);
}
fn main() {
let mut data = MUTABLE_GLOBAL_VARIABLE.lock().unwrap();
*data += 1;
println!("Mutable global variable: {}", *data);
}
Output:
Mutable global variable: 43
As you can see, we start by importing the necessary libraries: lazy_static
and std::sync::Mutex
.
The lazy_static!
macro is used to define a mutable global variable. This macro will ensure that the variable is only initialized when it is first accessed.
In our case, we name the variable MUTABLE_GLOBAL_VARIABLE
, give it the type Mutex<i32>
, and initialize it with the value 42
.
In the main
function, we access the global variable by calling MUTABLE_GLOBAL_VARIABLE.lock()
. This method acquires a lock on the Mutex
, ensuring that only one thread can access and modify the variable at a time.
We then increment the value within the lock, and finally, we print the updated value to the console.
By using lazy_static
, we can safely create and manage mutable global variables, ensuring they are correctly initialized and protected from data races when accessed by multiple threads. This approach simplifies working with the global state and contributes to the reliability and safety of your Rust programs.
Use the std::sync::RwLock
for Read-Write Global Variables
When you require a global variable that supports both read and write access, std::sync::RwLock
is a valuable alternative to Mutex
. This allows for concurrent read access while providing exclusive write access.
Let’s explore this concept through an example:
use lazy_static::lazy_static;
use std::sync::RwLock;
lazy_static! {
static ref RW_GLOBAL_VARIABLE: RwLock<i32> = RwLock::new(42);
}
fn main() {
let read_lock = RW_GLOBAL_VARIABLE.read().unwrap();
println!("Read global variable: {}", *read_lock);
// Release the read lock as soon as you're done with it
drop(read_lock);
let mut write_lock = RW_GLOBAL_VARIABLE.write().unwrap();
*write_lock += 1;
println!("Modified global variable: {}", *write_lock);
// Release the write lock explicitly by dropping it when you're done
drop(write_lock);
}
Output:
Read global variable: 42
Modified global variable: 43
Here, we begin by importing the required libraries: lazy_static
, which helps with lazy initialization, and std::sync::RwLock
, which provides a read-write lock.
The lazy_static!
macro is used to declare the global variable. In this example, it’s named RW_GLOBAL_VARIABLE
and is of type RwLock<i32>
. We initialize it with the initial value of 42
.
Inside the main
function, we demonstrate the capabilities of the read-write lock. First, we acquire a read lock using RW_GLOBAL_VARIABLE.read().unwrap()
, which allows multiple threads to access the variable simultaneously without any modification.
We print the value of the read-locked global variable, showing that multiple threads can read it concurrently without conflicts.
Next, we demonstrate the write lock. We acquire an exclusive write lock using RW_GLOBAL_VARIABLE.write().unwrap()
. This ensures that only one thread can modify the global variable at a time.
We increment the value within the write lock, and finally, we print the updated value. This showcases that the write lock prevents data races and ensures exclusive access during write operations.
By using RwLock
, you can design global variables that support both reading and writing operations in a thread-safe manner. This approach is particularly useful when you need concurrent access for reading while still maintaining data integrity during writes, all within the safety guarantees provided by Rust.
Use the Once
Primitive for Initialization
In certain situations, you may require a one-time initialization of global variables in Rust. To achieve this, Rust provides the std::sync::Once
primitive.
This primitive ensures that a specific function is executed only once, which is particularly useful for initializing global variables.
Let’s delve into this concept through a comprehensive example:
use std::sync::{Once, ONCE_INIT};
static mut GLOBAL_VARIABLE: i32 = 0;
static ONCE: Once = ONCE_INIT;
fn initialize_global() {
unsafe {
GLOBAL_VARIABLE = 42;
}
}
fn main() {
ONCE.call_once(|| initialize_global());
unsafe {
println!("Global variable: {}", GLOBAL_VARIABLE);
}
}
Output:
Global variable: 42
In this code, we start by importing the required libraries: std::sync::Once
for one-time initialization and ONCE_INIT
for a one-time initialization constant. We’ll be using these to set up the one-time initialization mechanism.
We declare a mutable global variable named GLOBAL_VARIABLE
. This variable is marked as unsafe
because we’ll be changing its value in a non-thread-safe manner, which is why we need to use unsafe
blocks.
We define the ONCE
static variable and initialize it with ONCE_INIT
. This Once
variable will manage the one-time initialization of the initialize_global
function.
The initialize_global
function is where we set the value of the GLOBAL_VARIABLE
. Since we are modifying a global variable in this function, it requires an unsafe
block.
In the main
function, we use ONCE.call_once(|| initialize_global())
to ensure that the initialize_global
function is called only once. The call_once
method takes a closure, and the closure’s code is executed on the first call. Subsequent calls to call_once
do nothing.
After the one-time initialization, we use an unsafe
block to safely print the value of the GLOBAL_VARIABLE
to the console.
This example illustrates how to use std::sync::Once
to guarantee that a particular function is executed only once, which is invaluable for initializing global variables in a thread-safe manner. By leveraging the Once
primitive, you can ensure that your global variables are properly initialized without risking data races or other synchronization issues.
Conclusion
Rust provides several ways to declare and use global variables, each with its use case.
The static
keyword is suitable for simple, immutable global variables. For a more complex or mutable global state, you can use the lazy_static
crate with Mutex
or RwLock
for synchronization.
Additionally, the std::sync::Once
primitive allows for one-time initialization of global variables, ensuring thread-safe behavior.
When working with global variables in Rust, it’s important to keep safety and concurrency in mind to prevent data races and maintain the language’s core principles of memory safety and thread safety.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook