How to Use the Ampersand in Rust
- Understanding the Ampersand in Rust
- Creating Immutable References
- Creating Mutable References
- Borrowing and Ownership
- Mutable and Immutable References Together
- Conclusion
- FAQ
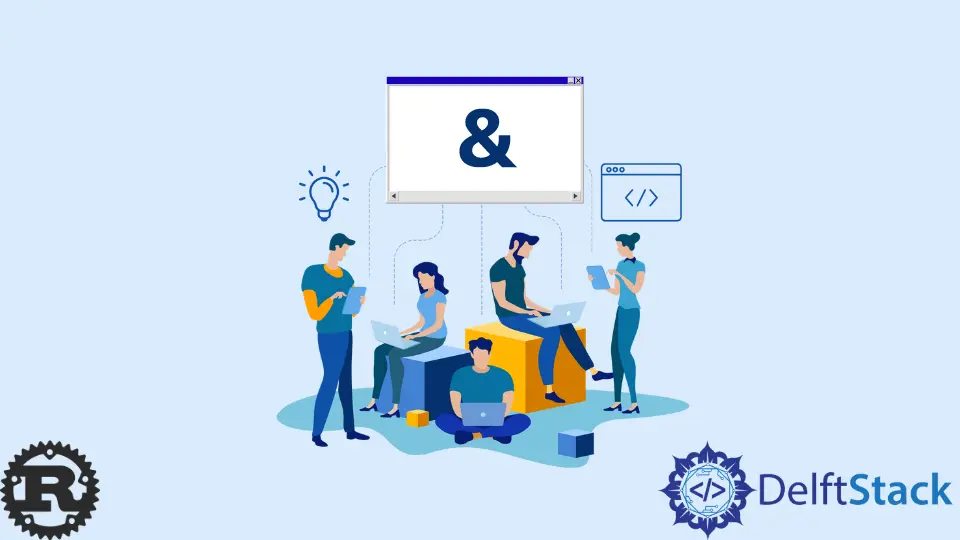
When diving into the Rust programming language, one feature that often puzzles newcomers is the ampersand symbol (&). This seemingly simple character plays a crucial role in managing variable mutability and immutability. Understanding how to use the ampersand effectively can significantly impact your coding experience in Rust. Whether you’re working with references or borrowing values, mastering the ampersand will enhance your ability to write efficient and safe code.
In this article, we will explore the various ways to utilize the ampersand in Rust, providing clear examples and explanations to help you grasp its functionality. Let’s get started!
Understanding the Ampersand in Rust
In Rust, the ampersand serves two primary purposes: it denotes references and helps manage mutability. References allow you to access data without taking ownership, while mutability determines whether you can modify the data. By default, variables in Rust are immutable, meaning they cannot be changed after they are initialized. However, you can create mutable references using the ampersand, allowing you to modify the underlying data.
To declare a mutable reference, you use the syntax &mut
. This tells the Rust compiler that you want to create a mutable reference to a variable. Conversely, if you simply use &
, you create an immutable reference. This distinction is crucial for ensuring memory safety and preventing data races in concurrent programming.
Creating Immutable References
To create an immutable reference in Rust, you simply use the ampersand before the variable name. This allows you to read the value of the variable without taking ownership. Here’s how you can do it:
fn main() {
let x = 5;
let y = &x;
println!("The value of y is: {}", y);
}
Output:
The value of y is: 5
In this example, x
is a variable initialized with the value of 5
. By using let y = &x;
, we create an immutable reference to x
. The value of y
can be accessed, but since it is an immutable reference, you cannot modify x
through y
. This ensures that the original value of x
remains unchanged, preserving the integrity of your data.
Creating Mutable References
If you need to modify a variable, you can create a mutable reference using &mut
. This allows you to change the value of the variable through the reference. Here’s an example:
fn main() {
let mut x = 5;
let y = &mut x;
*y += 1;
println!("The value of x is: {}", x);
}
Output:
The value of x is: 6
In this code, x
is declared as mutable with let mut x = 5;
. The line let y = &mut x;
creates a mutable reference to x
. The expression *y += 1;
dereferences y
to modify the value of x
. After this operation, the value of x
becomes 6
. This demonstrates how mutable references allow you to change the original variable, providing flexibility in your code.
Borrowing and Ownership
Understanding borrowing is essential when working with the ampersand in Rust. Borrowing allows you to temporarily use a variable without taking ownership. When you borrow a variable, you can create either an immutable or mutable reference. Here’s a simple example of borrowing:
fn main() {
let s = String::from("Hello");
let r = &s;
println!("Borrowed value: {}", r);
}
Output:
Borrowed value: Hello
In this example, we create a String
variable s
and then create an immutable reference r
to it. The original variable s
remains intact, and we can access its value through r
. This illustrates how borrowing works in Rust, allowing you to use data without transferring ownership.
Mutable and Immutable References Together
Rust enforces strict rules regarding mutable and immutable references. You cannot have mutable and immutable references to the same variable at the same time. This ensures that data races do not occur. Here’s an example that illustrates this concept:
fn main() {
let mut x = 5;
let y = &x; // Immutable reference
let z = &mut x; // Mutable reference (error)
println!("y: {}, z: {}", y, z);
}
This code will not compile because you cannot have an immutable reference y
and a mutable reference z
to x
simultaneously. Rust’s borrow checker prevents this situation to maintain memory safety. To fix this, you must ensure that the immutable reference is no longer in use before creating a mutable reference.
Conclusion
The ampersand in Rust is a powerful tool that allows you to manage variable mutability and immutability effectively. By understanding how to create immutable and mutable references, as well as the concepts of borrowing and ownership, you can write safer and more efficient code. Rust’s strict rules around references help prevent common programming errors, making it a robust choice for developers. As you continue to explore Rust, mastering the ampersand will undoubtedly enhance your coding skills and deepen your understanding of this unique language.
FAQ
-
What is the purpose of the ampersand in Rust?
The ampersand is used to create references, which allow you to borrow variables without taking ownership. -
How do I create a mutable reference in Rust?
You create a mutable reference using&mut
before the variable name. -
Can I have both mutable and immutable references to the same variable?
No, Rust does not allow mutable and immutable references to coexist for the same variable to ensure memory safety. -
What happens if I try to create a mutable reference while an immutable reference is still in use?
Rust will throw a compile-time error, preventing potential data races. -
Why is borrowing important in Rust?
Borrowing allows you to use data without taking ownership, which helps manage memory safely and efficiently.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook