How to Create Enums in Rust
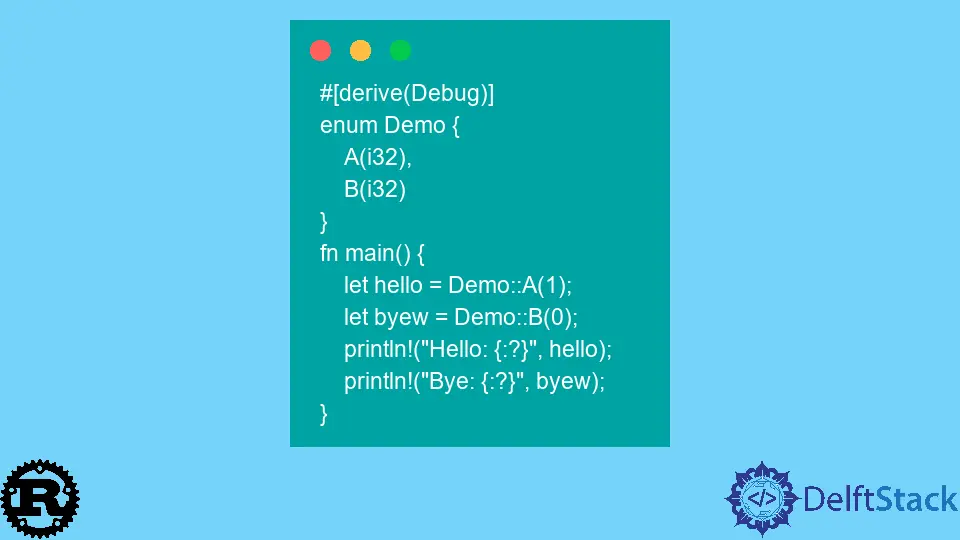
Enums, short for enumerations, are a powerful feature in Rust that allows developers to define types that can take on a limited set of values. The enum
keyword is used to create an enum type, while the variant
keyword is used to declare its variants. Each variant can hold any number of constants, known as enumerators. Understanding how to create and use enums in Rust is essential for effective programming in this language.
In this article, we will explore the process of creating enums, provide practical examples, and explain how they can enhance your Rust applications.
Understanding Enums in Rust
Enums in Rust are a way to define a type that can be one of several different variants. This is particularly useful when you want to represent a value that can have multiple forms. For example, you might want to represent a traffic light with three possible states: Red, Yellow, and Green. By using enums, you can create a type that clearly expresses these variations, making your code more readable and maintainable.
To define an enum in Rust, you use the enum
keyword followed by the name of the enum. Inside the curly braces, you define the variants. Each variant can be a simple name or can hold data. Here’s a basic example of defining an enum:
enum TrafficLight {
Red,
Yellow,
Green,
}
In this example, TrafficLight
is the enum type, and Red
, Yellow
, and Green
are its variants. This structure allows you to use TrafficLight
in your code to represent the state of a traffic light.
In the following sections, we will delve deeper into creating enums with data, pattern matching, and how to use them effectively in your Rust applications.
Creating Enums with Data
Enums can also hold data, which makes them even more powerful. Each variant can have different types and amounts of associated data. This feature allows you to create complex data structures that still maintain the clarity and safety that Rust is known for.
Here’s how you can define an enum with data:
enum Shape {
Circle(f64),
Rectangle(f64, f64),
Triangle(f64, f64, f64),
}
In this example, the Shape
enum has three variants: Circle
, Rectangle
, and Triangle
. The Circle
variant holds one f64
value representing the radius, while Rectangle
holds two f64
values for width and height, and Triangle
holds three f64
values for the lengths of its sides.
To use this enum, you can create instances of each variant like this:
let my_circle = Shape::Circle(2.5);
let my_rectangle = Shape::Rectangle(3.0, 4.0);
let my_triangle = Shape::Triangle(3.0, 4.0, 5.0);
By using enums with data, you can create a more structured and type-safe way to represent different shapes in your application. This not only improves code organization but also leverages Rust’s strong type system to catch errors at compile time.
Pattern Matching with Enums
One of the most powerful features of enums in Rust is pattern matching. This allows you to easily handle different variants of an enum in a clean and readable way. Pattern matching is done using the match
statement, which allows you to execute specific code based on the variant being matched.
Here’s an example of how you can use pattern matching with the Shape
enum we defined earlier:
fn area(shape: Shape) -> f64 {
match shape {
Shape::Circle(radius) => std::f64::consts::PI * radius * radius,
Shape::Rectangle(width, height) => width * height,
Shape::Triangle(a, b, c) => {
let s = (a + b + c) / 2.0;
(s * (s - a) * (s - b) * (s - c)).sqrt()
}
}
}
In this function, we calculate the area of different shapes based on the variant passed to the function. For the Circle
, we use the formula for the area of a circle. For the Rectangle
, we multiply the width by the height. For the Triangle
, we use Heron’s formula to calculate the area.
Using pattern matching not only makes your code cleaner but also ensures that you handle all possible variants of the enum, which can help prevent bugs and improve code reliability.
Conclusion
Enums in Rust are a fundamental feature that enhances the language’s expressiveness and safety. By defining types that can take on a limited set of values, you can create clear and maintainable code. Whether you are defining simple enums or more complex variants with associated data, understanding how to create and use enums effectively is crucial for any Rust programmer. With the power of pattern matching, you can handle different cases elegantly, ensuring your code is both efficient and robust.
FAQ
-
What is an enum in Rust?
An enum in Rust is a type that can represent one of several different values, known as variants. It allows for more structured and type-safe code. -
How do I define an enum in Rust?
You can define an enum using theenum
keyword followed by the name of the enum and its variants enclosed in curly braces. -
Can enums hold data in Rust?
Yes, enums in Rust can hold data, allowing each variant to have different types and amounts of associated data. -
What is pattern matching in Rust?
Pattern matching is a feature that allows you to execute specific code based on the variant of an enum. It is done using thematch
statement. -
Why should I use enums in my Rust code?
Enums improve code clarity, maintainability, and safety by allowing you to represent a value that can take on a limited set of forms, reducing the chance of errors.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook