Array.shift() in Ruby
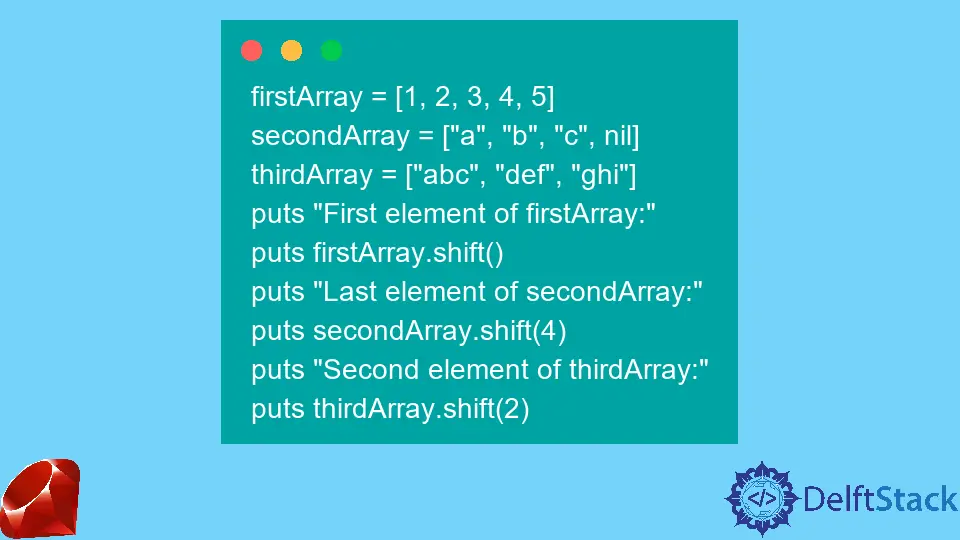
When working with arrays in Ruby, one of the most useful methods at your disposal is Array.shift()
. This method allows you to manipulate arrays by removing the first element. Whether you are building a game, processing data, or simply managing lists, understanding how to use Array.shift()
can significantly enhance your Ruby programming skills.
In this article, we will explore what Array.shift()
does, how to implement it in your code, and provide practical examples to illustrate its functionality. By the end of this guide, you will have a solid grasp of this essential method and be able to incorporate it into your Ruby projects with confidence.
What is Array.shift()?
Array.shift()
is a built-in method in Ruby that removes the first element from an array and returns that element. This method not only modifies the original array by shortening its length but also provides a way to access the removed element. If the array is empty, Array.shift()
will return nil
. This method is particularly useful when you need to process elements in a first-in-first-out (FIFO) manner, such as in queue implementations or when managing lists of tasks.
Here’s a simple example to illustrate how Array.shift()
works:
fruits = ["apple", "banana", "cherry"]
first_fruit = fruits.shift
puts first_fruit
puts fruits.inspect
Output:
apple
["banana", "cherry"]
In this example, Array.shift()
removes “apple” from the fruits
array and returns it. The original array is then modified to contain only “banana” and “cherry”. This demonstrates how Array.shift()
can be used to manage collections effectively.
How to Use Array.shift() in Ruby
Using Array.shift()
is straightforward. You simply call the method on an array object, and it will handle the rest. Here’s a deeper dive into its usage with more examples.
Basic Usage of Array.shift()
Let’s look at a more detailed example to see how Array.shift()
can be applied in various scenarios.
numbers = [1, 2, 3, 4, 5]
first_number = numbers.shift
puts first_number
puts numbers.inspect
Output:
1
[2, 3, 4, 5]
In this case, the first element, 1
, is removed from the numbers
array. The output shows that the original array is now [2, 3, 4, 5]
. This simple operation can be incredibly powerful when dealing with larger datasets or dynamic lists.
Using Array.shift() in Loops
Array.shift()
can also be effectively used within loops. This allows for more complex data manipulation. Here’s an example where we use Array.shift()
in a loop to process all elements of an array.
tasks = ["task1", "task2", "task3"]
while task = tasks.shift
puts "Processing #{task}"
end
Output:
Processing task1
Processing task2
Processing task3
In this example, we process each task in the tasks
array until it is empty. The loop continues to call Array.shift()
to remove and return the first element until there are no more tasks left. This pattern is particularly useful in scenarios such as task scheduling or event handling.
Handling Empty Arrays with Array.shift()
It’s important to know how Array.shift()
behaves with empty arrays. When you call Array.shift()
on an empty array, it simply returns nil
. Here’s how that looks in practice:
empty_array = []
result = empty_array.shift
puts result.inspect
Output:
nil
This behavior is crucial to handle gracefully in your code. If you are processing an array that could potentially be empty, always check the return value of Array.shift()
to avoid unexpected errors.
Conclusion
In summary, Array.shift()
is a powerful method in Ruby that allows you to manipulate arrays by removing the first element. Whether you are managing a list of items, processing tasks, or implementing data structures, understanding how to use Array.shift()
can greatly enhance your coding capabilities. With its simple syntax and versatile applications, this method is a must-know for any Ruby developer. Start experimenting with Array.shift()
in your projects today, and see how it can streamline your array management tasks.
FAQ
-
What does Array.shift() do in Ruby?
Array.shift() removes the first element from an array and returns that element. -
Can Array.shift() return nil?
Yes, if the array is empty, Array.shift() will return nil. -
How does Array.shift() affect the original array?
Array.shift() modifies the original array by removing the first element, thereby shortening its length. -
Is Array.shift() suitable for processing large arrays?
Yes, Array.shift() can be used to process large arrays, especially in scenarios requiring FIFO (first-in-first-out) operations. -
Can I use Array.shift() in a loop?
Absolutely! Array.shift() is often used in loops to process all elements of an array until it is empty.