How to Replace Strings in Ruby
- Different Methods for Ruby Replace
-
Use the
replace()
Method to Replace Strings in Ruby -
Use the
gsub()
Method to Replace Strings in Ruby -
Use the
sub()
Method to Replace String in Ruby - Conclusion
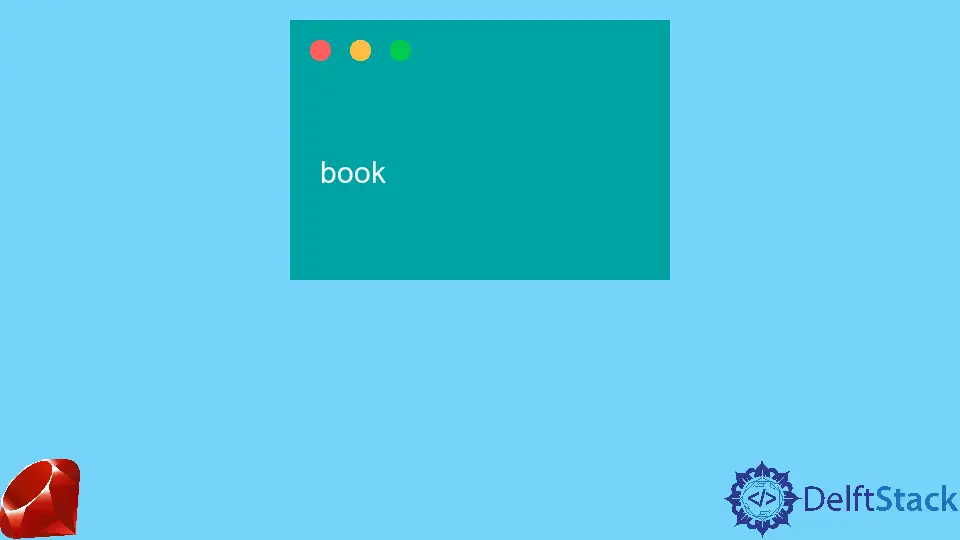
This article will explain the different methods used to replace strings in Ruby.
Different Methods for Ruby Replace
Ruby provides the best code if you want to replace the strings in your coding. In Ruby, the String class provides several methods for creating, manipulating, and searching strings.
replace()
methodgsub()
methodsub()
method
In the first method, as the name said, you can replace the string easily by the replace
method.
One of these is the gsub
method, which stands for global substitution. As its name suggests, gsub
allows you to replace all occurrences of a given substring with another string.
Another method is the sub
method, which is also beneficial for replacing the strings.
Use the replace()
Method to Replace Strings in Ruby
The replace()
method takes two arguments: the text to be replaced and the text to replace it.
puts 'pen'.replace('book')
Output:
book
Use the gsub()
Method to Replace Strings in Ruby
The most standard method is the gsub()
method. The gsub()
method in Ruby replaces all occurrences of a fixed string with another string.
The specified string can be a regular expression, and the replacement string can be either a block or a string. If a block is specified, it will be called for each occurrence of the specified string, and the return value will be used as the replacement string.
This method takes two arguments, the first is the word or phrase you like to replace, and the second is the alternate word or phrase.
Code Example:
puts 'I have a pen.'.gsub('pen', 'lion')
Output:
I have a lion
For example, let’s say you have a string that contains the word “ruby” multiple times, and you want to replace it with the word “python”. You could do so like the following.
Code Example:
str = 'I love ruby, but I also love python'
puts str.gsub('ruby', 'python')
Output:
I love python, but I also love python
As you can see, all occurrences of “ruby” in the original string have been replaced with “python”.
gsub()
also allows you to use a regular expression as the first argument, which can be helpful for more complex replacement tasks.
For example, let’s say you want to replace all occurrences of “lion” or “cat” with the word “fruits”.
Code Example:
str = 'I love lion, but I also love cat'
puts str.gsub(/lion|cat/, 'fruits')
Output:
I love fruits, but I also love fruits
In this case, the regular expression /lion|cat/
matches both “lion” and “cat”, so both are replaced with “fruits”.
Finally, it’s worth noting that gsub
returns a new string containing the modified text. It doesn’t modify the original string.
Use the sub()
Method to Replace String in Ruby
The sub method in Ruby is a powerful tool that allows you to replace substrings in a string with another string.
For example, if you have a string “I am a human”, and you want to replace the word “human” with the word “object”, you could use the sub method like this.
Code Example:
puts 'I am a human'.sub('human', 'object')
Output:
I am a object
You can also use regular expressions with the sub method, which can be very useful for complex replacements.
If you only like to replace the earliest word or phrase, you can use the sub()
method. This works the same as gsub()
, except it only replaces the first occurrence.
A few other methods can replace words in a string, but these are the most common.
Conclusion
In conclusion, the Ruby replace method is a powerful tool for manipulating strings. It can replace substrings, change cases, and strip whitespace.
With creativity, it can even be used to perform complex tasks such as converting HTML to plain text. Ruby is a very concise and readable language, making it easy to write code that is both effective and easy to read.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn