How to Get Current Time in UTC in Ruby
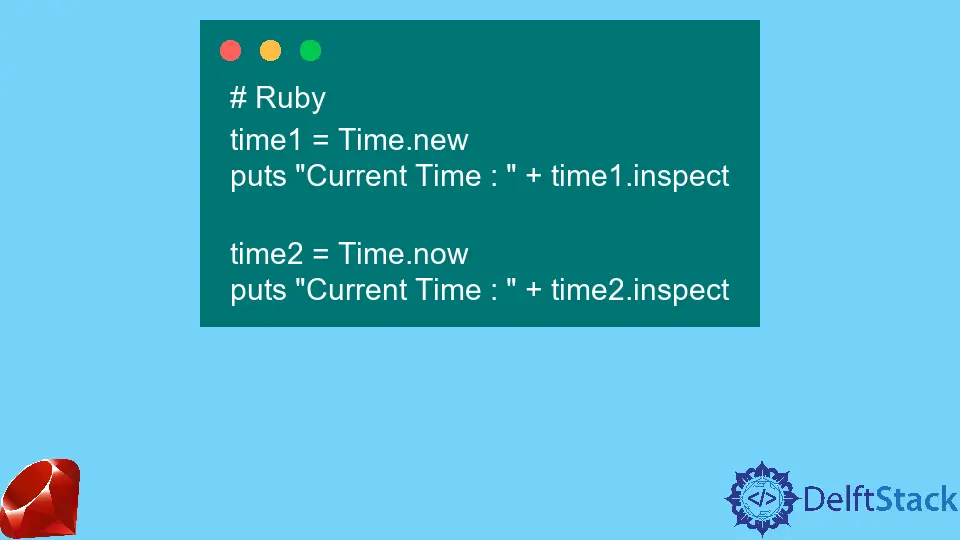
This article will tackle the time class act for dates and times in Ruby.
Use Time.now
in Ruby
The operating system provides date and time functionality. Before 1970 or after 2038, this class may be unable on our system to represent dates.
You can use the Time.now
in Ruby to represent a time and date. The three components of a date are Day
, Month
, and Year
.
Talking about time
also has three components: Hours
, Minutes
, and Seconds
.
We can create a Time object using a Unix timestamp at the method, and you can give the starting date in numbers to Time.new
(Format: year/month/day). We can get an object representing the current time using Time.now
.
Example Code:
# Ruby
time1 = Time.new
puts 'Current Time : ' + time1.inspect
time2 = Time.now
puts 'Current Time : ' + time2.inspect
Output:
Time objects are used to get various components of date and time.
Example Code:
# Ruby
time = Time.new
Output:
The following list is other methods used for different functions.
time.inspect
- get the current time of the day.time.year
- get the year of the date.time.month
- get the month of the date.time.wday
- get the day of the week. The value can range from1
to7
.time.yday
- get the day of the year. The value can range from11
to365
.time.hour
- get the hour with the format of a 24-hour clock.time.min
- get the value of the minutes.time.sec
- get the value of the seconds.time.usec
- get the microseconds.time.zone
- get the zone of the time.
The UTCTime.utc
, Time.gm
, and Time.local
functions are used in a standard format to format data. In the syntax below, we get all the components in an array.
# ruby
[seconds, minutes, hour, day, month, year, wday, yday, isdst, zone]
Example Code:
# Ruby
time = Time.new
values = time.to_a
puts values
Output:
Time objects are used to get various components of date and time.
Example Code:
# Ruby
time = Time.new
values = time.to_a
puts Time.utc(*values)
Output:
We can use time objects to get all the time zones and daylight savings information.
# ruby
time = Time.new
Methods | Description |
---|---|
time.utc_offset |
Get the difference between our time zone and UTC zone. |
time.isdst |
To check if UTC has DST. |
time.utc? |
Checks whether we are in UTC or not. |
time.locatime |
Get the time in our local time zone. |
time.gmtime |
Converts back to the UTC. |
time.getlocal |
To get a new time object in the local time zone. |
time.getutc |
To get a new time object in UTC. |
If we want to format times and dates, There are many ways to format dates and times.
Example Code:
# Ruby
time = Time.new
puts time
puts time.ctime
puts time.localtime
puts time.strftime('%Y-%m-%d %H:%M:%S')
Output:
Time Formatting Directives
The following directives are used with the method time.
Abbreviation | Description |
---|---|
%a and %A |
%a is used for weekday names (Sun ) and %A for the full weekday name (Sunday ). |
%b and %B |
%b is used for the month name (Jan ) and %B for the full month name (January ). |
%c |
Preferred local date and time representation. |
%d |
Used a day of the month ranging from 01 to 31 . |
%H |
Used for an hour of the day ranging from 00 to 23 . |
%I |
Used for an hour of the day (01 to 12 ). |
%j |
Used for a day of the year (001 to 336 ). |
%m and %M |
%m is used for the month of the year from 01 to 12 , and %M is used for a minute of the hour from 00 to 59 . |
%p |
Used for the meridian indicator, AM or PM . |
%S |
Used for the second of the minute from 00 to 60 . |
%U |
Used for the first Sunday as the first day of the first week, ranging from 00 to 53 . |
%w and %W |
%w is used for a day of the week range from 0 to 6 (Sunday is 0 ), and %W is used for the first Monday as the first day of the first week from 00 to 53 . |
%x and %X |
%x used only for a date, and %X used only for a time. |
%y and %Y |
%y is used for a year without a century, ranging from 00 to 99 and %Y used for the year of the century. |
%z |
Used for time zone. |
%% |
Used for literal % character. |
Time Arithmetic in Ruby
We can do simple arithmetic with time, such as follows.
Example Code:
# Ruby
now = Time.now
puts now
past = now - 10
puts past
future = now + 10
puts future
diff = future - past
puts diff
Output: