Fibonacci Sequence in Ruby
- Use a Loop to Get the Fibonacci Sequence in Ruby
- Create a Function to Get the Fibonacci Sequence in Ruby
- Use a Recursive Approach to Get the Fibonacci Sequence in Ruby
- Conclusion
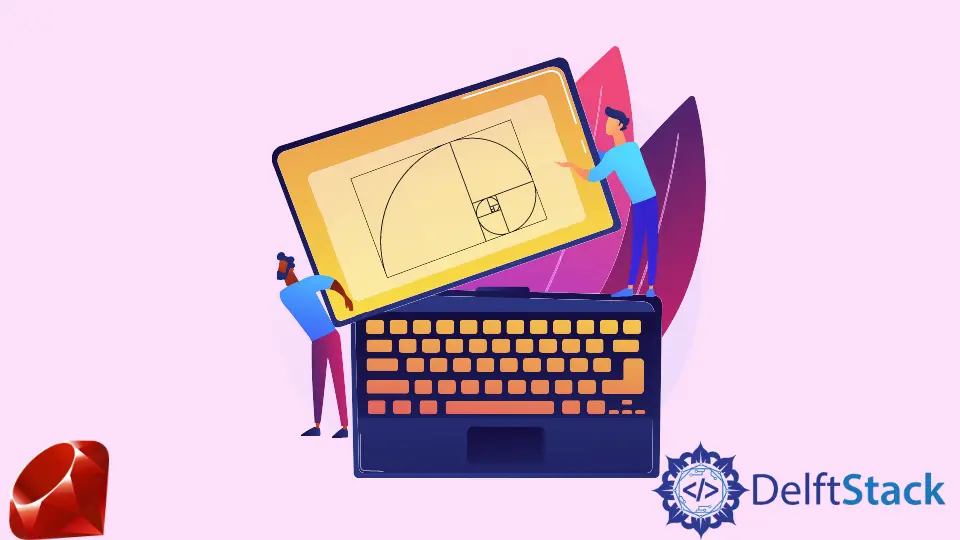
The Fibonacci sequence is a series of numbers, with each current number being the sum of the previous two numbers. This sequence plays a significant role in various mathematical and programming contexts.
For instance, the Fibonacci sequence up to 10
terms is: 1, 1, 2, 3, 5, 8, 13, 21, 34, 55
. Here, each number, except the first two, is the sum of the two preceding numbers.
We will introduce the Fibonacci sequence in Ruby with examples.
Use a Loop to Get the Fibonacci Sequence in Ruby
We may need to get a range of Fibonacci sequences, or we may want to get the Fibonacci sequence up to a specific number. We can use Ruby to get the Fibonacci sequence of any number of terms.
We can use a loop to generate the Fibonacci sequence. We’ll start with an example where we initialize the first two numbers and use a loop to calculate the subsequent ones.
The loop used in the following code is a times
loop. The times
loop is a way to iterate over a block of code a specific number of times, and it is often used when you know exactly how many iterations you want to perform.
Basic Syntax - times
loop:
n.times do
# code to be repeated
end
In the syntax, the n
is the number of times you want to execute the code inside the block. The block of code will be executed n
times.
Code:
firstNum = 0
secondNum = 2
5.times do
firstNum, secondNum = secondNum, firstNum + secondNum
puts firstNum
end
In the code, we initialize two variables, firstNum
and secondNum
, with values 0
and 2
, respectively. Using a loop executed 5 times (5.times do
), we iteratively update these variables to represent the next numbers in the Fibonacci sequence.
The line firstNum, secondNum = secondNum, firstNum + secondNum
utilizes parallel assignment to swap values efficiently, and puts firstNum
prints the current value of firstNum
in each iteration.
Output:
2
2
4
6
10
The output displays the first 5 numbers in the Fibonacci sequence, starting with 2 and continuing with the sum of the previous two numbers in each step. The above example shows that we can get the Fibonacci sequences for as long as possible using the loop.
Create a Function to Get the Fibonacci Sequence in Ruby
Now, let’s create a function in Ruby that will give us the Fibonacci sequence up to a certain number. First of all, we will define the function.
Inside our function, we will define the first two steps of the Fibonacci sequence.
Now, we will use the loop to get the Fibonacci sequence of a number that a user will pass. As shown below, we will run the loop one less time than the passed number.
Code:
def getFib(x)
firstNum = 0
secondNum = 1
(x - 1).times do
firstNum, secondNum = secondNum, firstNum + secondNum
puts firstNum
end
end
getFib(10)
In the code, we define a function named getFib
that takes a parameter x
. Inside the function, we initialize two variables, firstNum
and secondNum
, to 0
and 1
, respectively.
Using a loop executed (x - 1)
times, we iteratively calculate and print the next numbers in the Fibonacci sequence. The line firstNum, secondNum = secondNum, firstNum + secondNum
efficiently updates the variables, and puts firstNum
prints the current value of firstNum
in each iteration.
Finally, when we call getFib(10)
, the function generates and outputs the first 10 numbers in the Fibonacci sequence.
Output:
1
1
2
3
5
8
13
21
34
As you can see from the above example, we can easily get the Fibonacci sequence of any number by creating a function and defining the Fibonacci sequence inside that function. We can adjust the argument passed to getFib
to generate the sequence with a different number of terms.
Use a Recursive Approach to Get the Fibonacci Sequence in Ruby
Another method to generate the Fibonacci sequence in Ruby is by using a recursive approach. A recursive approach to generating the Fibonacci sequence in Ruby involves defining a method that calls itself to calculate the Fibonacci numbers.
The recursive function stops when it reaches the base case, which is often when the input is 0
or 1
.
Code:
def fibonacci_recursive(n)
return n if (0..1).include? n
fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2)
end
11.times { |n| puts fibonacci_recursive(n) }
In the code, we define a recursive function named fibonacci_recursive
that takes a parameter n
. The function serves to calculate the Fibonacci sequence recursively.
The base case checks if n
is 0 or 1, in which case it returns n
. Otherwise, the function calls itself twice with the arguments n - 1
and n - 2
, summing their results.
We then use a range from 0 to 10 in a loop, calling the function for each value of n
and printing the result.
Output:
0
1
1
2
3
5
8
13
21
34
55
The output displays the Fibonacci sequence from the 0th to the 10th term and showcases the Fibonacci sequence calculated by the recursive function, where each number is the sum of the two preceding ones.
However, keep in mind that recursive solutions like this can become inefficient for larger values of n
due to repeated calculations.
Conclusion
In conclusion, we explored various methods to generate the Fibonacci sequence in Ruby. First, we used a loop to efficiently obtain the sequence by iteratively updating variables and printing the results.
Next, we created a function that allowed us to generate the Fibonacci sequence up to a specified number by employing a loop within the function. This modular approach enhances code reusability and readability.
Finally, we utilized a recursive approach, defining a function that calculates the Fibonacci numbers by calling itself. Each method provided a unique perspective on generating the Fibonacci sequence in Ruby, showcasing flexibility in implementation.