Assertions in Ruby
- What Are Assertions?
- Using Assertions in RSpec
- Using Assertions in Minitest
- Best Practices for Using Assertions
- Conclusion
- FAQ
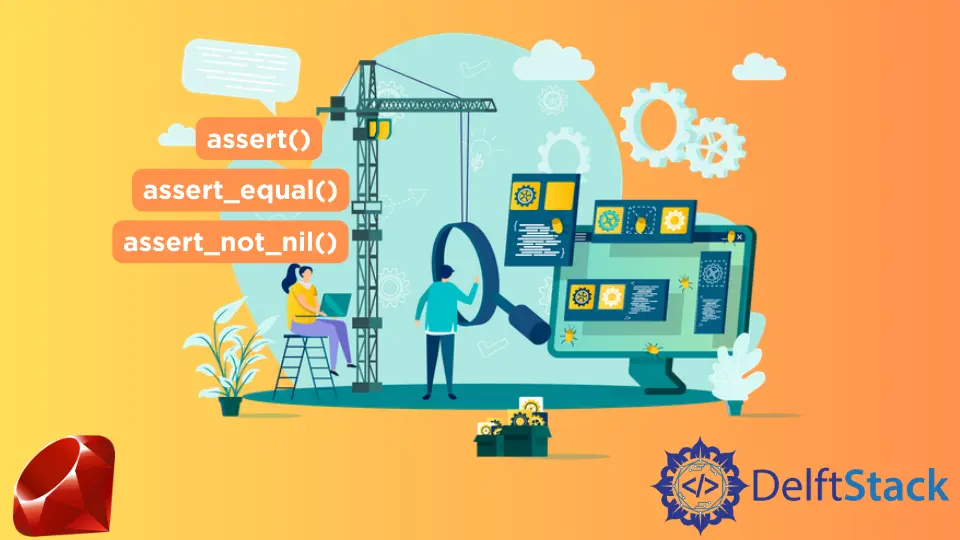
Assertions are a fundamental concept in programming, often used in testing to verify that a condition holds true. In Ruby, assertions can help developers ensure that their code behaves as expected.
This tutorial will explore what assertions are in Ruby, how to implement them, and the best practices for using them effectively. Whether you’re a beginner looking to understand the basics or an experienced developer wanting to refine your testing skills, this guide will provide valuable insights. By the end, you’ll have a solid grasp of assertions and how they can enhance your Ruby programming experience.
What Are Assertions?
Assertions are statements that check whether a condition is true. If the condition evaluates to false, the assertion will typically raise an error, signaling that something is wrong. In Ruby, assertions are commonly used in testing frameworks like RSpec and Minitest. They help ensure that your code behaves as expected by checking the results of operations against expected outcomes.
For example, if you have a method that adds two numbers, you can use an assertion to verify that the output is indeed the sum of those numbers. This practice not only helps catch bugs early but also serves as documentation for your code, making it clearer what the expected behavior is.
Using Assertions in RSpec
RSpec is one of the most popular testing frameworks in Ruby, and it provides a robust way to implement assertions. To get started, you’ll first need to install RSpec if you haven’t already. You can do this by adding it to your Gemfile:
gem 'rspec'
After installing, you can create a simple test file. Here’s an example of how to use assertions in RSpec:
require 'rspec'
def add(a, b)
a + b
end
RSpec.describe 'Addition' do
it 'adds two numbers' do
expect(add(2, 3)).to eq(5)
end
end
Output:
.
Finished in 0.002 seconds (files took 0.080 seconds to load)
1 example, 0 failures
In this example, we define a method add
that takes two parameters and returns their sum. The RSpec block describes the behavior we expect from the add
method. The expect
method is where the assertion happens. If the result of add(2, 3)
does not equal 5
, RSpec will raise an error, indicating that the test has failed.
Using RSpec’s assertions allows you to write clear and expressive tests. This clarity makes it easier to understand what your tests are checking for, which is especially helpful when working in teams or revisiting code after some time.
Using Assertions in Minitest
Minitest is another popular testing framework in Ruby, known for its simplicity and speed. If you prefer a more minimalistic approach, Minitest is a great choice. Here’s how to implement assertions using Minitest:
First, ensure Minitest is included in your Gemfile:
gem 'minitest'
Now, you can create a test file similar to the one used with RSpec. Here’s an example:
require 'minitest/autorun'
def multiply(a, b)
a * b
end
class TestMath < Minitest::Test
def test_multiply
assert_equal 15, multiply(3, 5)
end
end
Output:
.
Finished in 0.001234 seconds
1 tests, 1 assertions, 0 failures, 0 errors, 0 skips
In this Minitest example, we define a method multiply
that returns the product of two numbers. The assert_equal
method is used to assert that the output of multiply(3, 5)
equals 15
. If the assertion fails, Minitest will report the failure, making it easy to identify issues in your code.
Minitest provides a straightforward way to write tests without the need for additional configuration. Its simplicity allows you to focus on writing effective tests that enhance your code’s reliability.
Best Practices for Using Assertions
When using assertions in Ruby, it’s essential to follow best practices to maximize their effectiveness. Here are some tips to consider:
-
Be Clear and Concise: Write assertions that are easy to understand. A clear assertion helps both you and others quickly grasp what the test is verifying.
-
Test One Thing at a Time: Each test should focus on a single aspect of your code. This approach makes it easier to identify the source of a failure.
-
Use Descriptive Names: Name your test methods descriptively to indicate what they are testing. This practice improves readability and helps during debugging.
-
Run Tests Frequently: Regularly running your tests can catch issues early in the development process. Make it a habit to run your test suite after making significant changes.
-
Refactor Tests as Needed: As your code evolves, your tests may need to change too. Don’t hesitate to refactor your tests to keep them relevant and effective.
By following these best practices, you can create a robust suite of tests that will help ensure your Ruby applications are reliable and maintainable.
Conclusion
Assertions in Ruby are a powerful tool for ensuring that your code behaves as expected. Whether you choose to use RSpec or Minitest, implementing assertions can significantly enhance the reliability of your applications. By writing clear and concise tests, you not only catch bugs early but also create documentation that helps others (and your future self) understand the intended behavior of your code. Embrace assertions in your Ruby development journey, and you’ll find that your coding experience becomes more efficient and enjoyable.
FAQ
-
What are assertions in Ruby?
Assertions are statements that check whether a condition is true, helping to ensure that your code behaves as expected. -
How do I use assertions in RSpec?
You can use theexpect
method in RSpec to create assertions, checking that the output of your code matches expected values. -
What is the difference between RSpec and Minitest?
RSpec is more expressive and provides a behavior-driven development approach, while Minitest is simpler and faster, focusing on minimalism. -
Why are assertions important in testing?
Assertions help catch bugs early, ensure code reliability, and serve as documentation for expected behavior. -
Can I use assertions in any Ruby project?
Yes, assertions can be used in any Ruby project, especially those that involve testing frameworks like RSpec or Minitest.