How to Scroll to an Element in React
-
Scroll to an Element With the
Element.scrollIntoView()
Method in React - Scroll to an Element With React Refs (References)
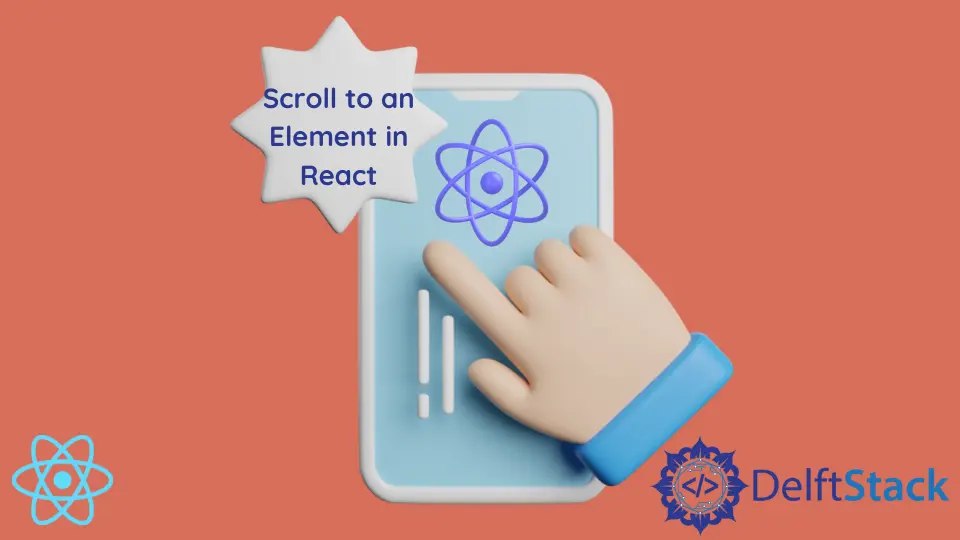
The modern internet is filled with text all types of content. Quite often, you have to scroll to find what you’re looking for. However, scrolling endless feed can be frustrating and a bad UX practice. One solution is to create a table of contents.
React frameworks offer a better way to achieve the same result. You can refer to an element and scroll to it, either automatically or by clicking a button. Detecting what users are looking for and instantly displaying it can increase the user-friendliness of your app or website.
There are multiple ways to implement this feature. Depending on what type of components you’re using (functional vs class), you have to choose the appropriate one.
Still, every implementation consists of two bits: the Element.scrollIntoView()
method provided by HTML element interface and React refs (short for references), which can be created either using useRef()
hook (for functional components), or createRef()
method (for class components).
Scroll to an Element With the Element.scrollIntoView()
Method in React
As previously mentioned, this method ensures that the scroll moves up or down to show whichever element it is called upon.
element.scrollIntoView()
can only accept one argument. It can be either a alignToTop
Boolean or options
Object.
alignToTop
Passing a true
Boolean value will result in the element being aligned to the top of the scrollable parent element.
Passing a false
value will align the element to the bottom of the scrollable parent element.
Options
This argument is represented as an object but allows you to customize and change the method’s default settings.
The object can have three optional properties. In the event of their absence, the settings revert to default.
- The
behavior
property allows you to customize the transition. By default, it is set toauto
. It can be set tosmooth
, which is a better-looking animation. - The
block
property allows you to position the element vertically. It can take the value ofstart
,center
,end
, ornearest
.start
is the default setting. - The
inline
property allows you to position the element horizontally. It can take the value ofstart
,center
,end
, ornearest
.nearest
is the default setting.
Browser Support
The element.scrollIntoView()
method itself is supported by all major browsers. However, some old browsers will ignore the options
argument passed into it. According to caniuse.com, only ~76% of users worldwide have browsers that support this argument. The website describes it as an experimental feature.
Scroll to an Element With React Refs (References)
Refs in React have many different applications. One of the most common uses is to reference elements in the DOM. By referencing the element, you also gain access element’s interface. This is essential to capture the element to which we want to scroll.
An element can be referenced by setting its ref attribute to the reference instance. Here’s the code example:
const testDivRef = useRef(null);
<div className = 'testDiv' ref = {testDivRef}>< /div>
Here, the <div>
element is the one we want to scroll into view. You can use react references on any other HTML element as well.
<h1 ref={testDivRef}>Title</h1>
The current
property of the reference object holds the actual element. Here’s what we get if we log the testDivRef.current
onto the console:
Below is the full solution.
In a functional component:
function TestComponent() {
const testRef = useRef(null);
const scrollToElement = () => testRef.current.scrollIntoView();
// Once the scrollToElement function is run, the scroll will show the element
return (
<>
<div ref={testRef}>Element you want to view</div>
<button onClick={scrollToElement}>Trigger the scroll</button>
</>
);
}
In a class component:
class TestComponent extends Component {
constructor(props) {
super(props);
this.testRef = React.createRef();
}
scrollToElement = () => this.testRef.current.scrollIntoView();
// Once the scrollToElement method is run, the scroll will show the element
render() {
return <div ref = {this.testRef}>Element you want in view < /div>;
}
}
Additional Tips:
The current
property will only become accessible once the component has mounted.
It’s best to reference the property in a useEffect()
callback or equivalent (which is componentDidMount()
lifecycle method for class components).
useEffect(() => console.log(testRef.current), [])
You can also conditionally check if ref.current
equates to true before proceeding.
if (testRef.current) {
testRef.current.scrollIntoView()
}
You can set up the scroll to be triggered by clicking a button. This way, you can ensure that the scroll will be executed once the button and the component that should be viewed are mounted.
<button onClick={scrollToElement}>Trigger the scroll</button>
a Bundled Solution
NPM package called react-scroll-to-component
was built specifically to provide this feature as a bundled solution. It is easy to install with npm or yarn.
To learn more about this package, read the documentation.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn