How to Return Type for React Components in TypeScript
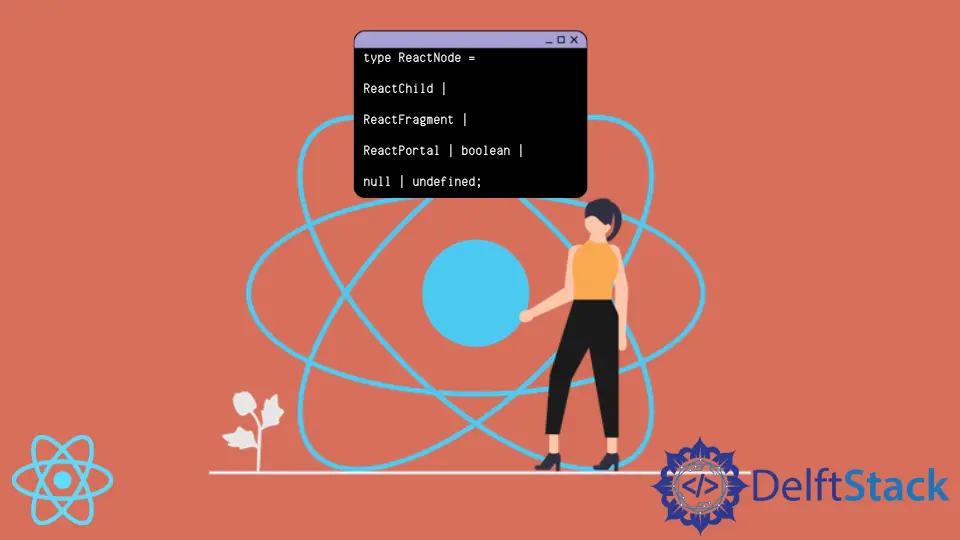
React is a modern JavaScript library that allows you to create good-looking UIs for your web apps. A growing number of developers are embracing a subset of JavaScript called TypeScript.
Static type-checking can bring structure and order to your code and help you detect bugs faster.
Normally, when writing a React application in TypeScript, assigning return type to components is unnecessary. However, sometimes it’s necessary to do so.
For instance, a company-wide linting rule might require developers to specify the return type for components.
This article will discuss the three types that can apply to components. These are JSX.Element
, ReactNode
, and ReactElement
.
We’ll discuss the similarities and the differences between them.
Return Types for React Components in TypeScript
If you need to assign a type to a component, you can choose from one of the three types listed above. The differences between JSX.Element
, ReactNode
, and ReactElement
are subtle but important.
Understanding each type will help you write error-free and more accurate TypeScript code.
ReactElement
Type in TypeScript
It’s an interface from the @types/react
package. The render()
functions of functional components return the ReactElement
type.
If you must assign a type to functional components in React, it should be this one.
If there’s a chance that the component will return null
, you should use the or ||
operator to assign it the type ReactElement || null
.
The actual interface definition from the @types/react
package looks like this.
interface ReactElement<P = any, T extends string | JSXElementConstructor<any> = string | JSXElementConstructor<any>> {
type: T;
props: P;
key: Key | null;
}
You can think of ReactElement
as a primitive type of component. Other types listed below are built on top of this basic interface.
JSX.Element
Type in TypeScript
The type
and props
of this type are set to any
, so the definition is even broader than ReactElement
.
It exists because React is not the only library that relies on JSX.
JSX.Element
allows other frameworks to define their own, more specific types on top of this one. In the case of React, ReactElement
is a more specific type based on JSX.Element
.
If you want to specify the return type of a functional component, you can use either ReactElement
or JSX.Element
. Let’s look at the example that uses the latter.
type sampleProps = {
someText: string;
};
const App = ({ someText }: sampleProps): JSX.Element => <p>{someText}</p>;
ReactNode
Type in TypeScript
The type is returned by React class components’ render()
function. It can be a ReactElement
, ReactFragment
, ReactText
, a string, a Boolean, and other falsy values in JavaScript, such as undefined
or null
.
Below is the official definition of the ReactNode
type from the @types/react
package.
type ReactNode = ReactChild | ReactFragment | ReactPortal | boolean | null | undefined;
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn