The Purpose of Strict Mode in React
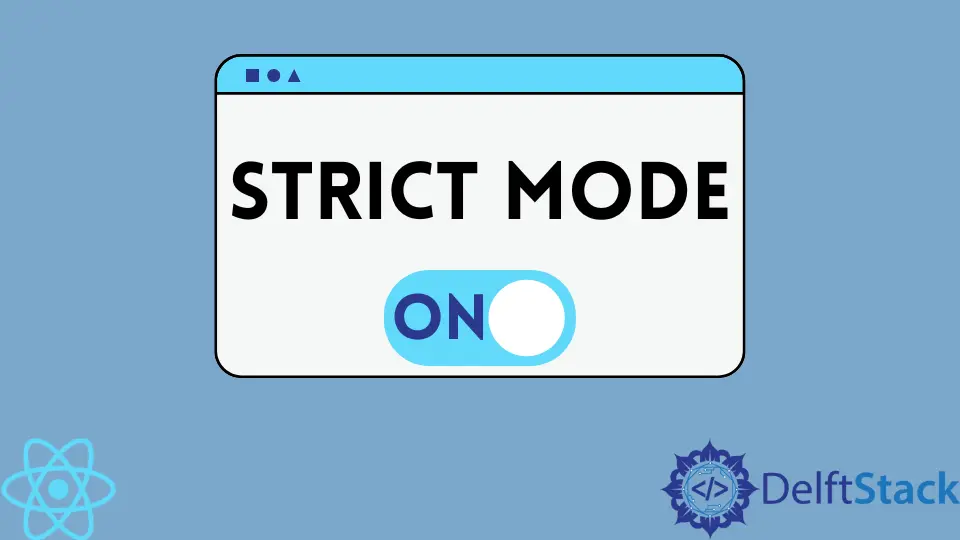
JavaScript is a great programming language for developing full-stack applications. Newbie developers often struggle with writing clean, organized JavaScript code. Fortunately, there are JavaScript frameworks that provide some structure for writing web applications. And React is one of, if not the most, popular framework for writing efficient web applications. It provides a helper component called StrictMode
to highlight code pieces that deviate from standard practices in React.
Strict Mode in React
React StrictMode
highlights potential problems in the application. By doing so, it helps you write more readable and safe applications. This feature is included in the React package, so you don’t have to import it separately. If you have any suspicious code, wrap it within the StrictMode
helper component, and that’s it. Here’s how to use it in functional React components:
import React, { Component } from 'react';
function SuspiciousComponent() {
return (
<div>Let's say this is suspicious</div>
)
}
function App() {
return (
<div>
<React.StrictMode>
<SuspiciousComponent></SuspiciousComponent>
</React.StrictMode>
</div>
)
}
In this case, we just wrapped <SuspiciousComponent>
with our helper component. If we changed the child component’s code to something that goes against the recommended practices in React, StrictMode
would raise the alarm. This is necessary because some bad practices might not crash the app. Just because the app works doesn’t mean there are no problems with it. If you’re suspicious about a piece of code, wrap it with the <React.StrictMode>
helper component to ensure that nothing’s wrong with it. You can wrap a single component, multiple components, or an entire tree.
If you want to look for potential errors in the entire component tree, look at the parent component’s return
statement and wrap the <div>
(or some other element) that contains all other components. If you’ve built a complex application, StrictMode
will inevitably detect some errors and report them to the console.
Just like the Fragment
helper component, StrictMode
does not render any actual UI. Nor does it affect the styles of your application.
React Strict Mode is used for detecting bugs in development mode. Once you’ve cleaned up all errors and the app is ready for production, you can remove it.
Advantages of React Strict Mode
Besides the obvious benefits of using StrictMode
in React, it can also be a helpful tool for learning to write better quality code.
Protecting From Side Effects
Some lifecycle methods may be called multiple times during the render phase, so React recommends that they shouldn’t contain any side effects. This recommendation is often overlooked, leading to incorrect state and memory leaks that expose your application to outside attacks. Fortunately, the StrictMode
helper component can help you locate harmful side effects and fix them. And to achieve this, the methods of components wrapped with StrictMode
are purposefully called twice.
Detect Outdated Practices
Features like findDOMNode
were once allowed in ReactJS, but they’re now deprecated. The same goes for context
API, which has been widely used in the past but is about to be removed. StrictMode
can be a useful tool for raising the alarm about outdated methods or React-specific features.
Best Uses for React Strict Mode
If you’re working with a codebase that you didn’t write, StrictMode
can be a helpful tool for ensuring the proper organization of the code, especially if an inexperienced developer wrote the React application.
Finding a bug in JavaScript (laced with React) code can be quite difficult. But wrapping the code with StrictMode
might give you some ideas about what might be wrong.
Overall, StrictMode
is one of the most useful tools in React. Newbie developers can use it to get into the habit of writing code that adheres to recommended practices in React. The more you play around with Strict Mode, the more comfortable you become using it.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn