How to Refresh a Page in React
-
Use
reload()
Method to Refresh a Page in React Manually - Update the State of the Component to Refresh a Page in React
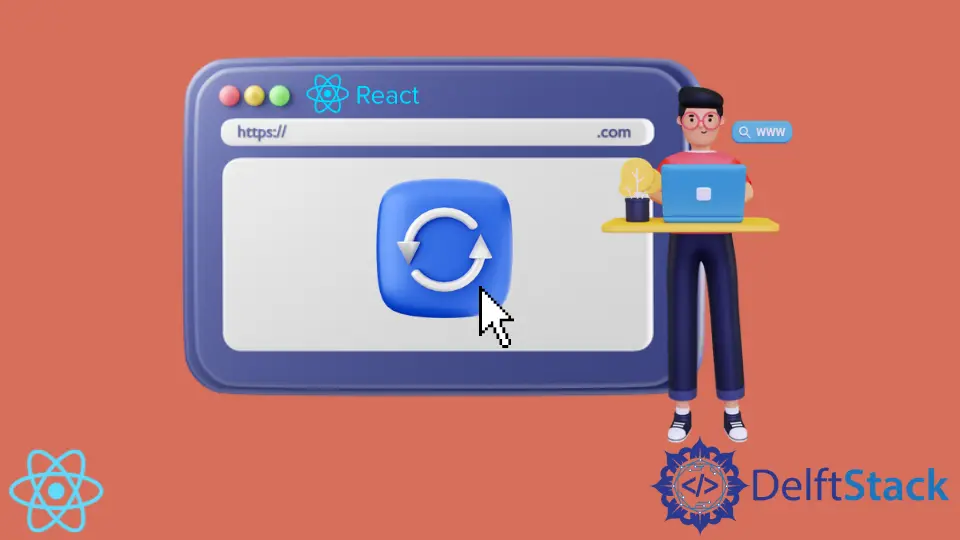
One of the significant advantages of React is that you don’t need to refresh the page to see the most recent updates. React automatically updates the view whenever the state changes.
Still, sometimes it’s necessary to refresh the page manually. For instance, a simple reload can fix inconsistencies in the state.
Other times, you might want to undo some unnecessary actions. Let’s see how you can manually refresh the page in React, whatever the reason may be.
Use reload()
Method to Refresh a Page in React Manually
Most of the time, we want to trigger a manual refresh in React after the user clicks on a button, a text, or some other element. To do that, we’ll need to create an event handler. Browsers natively provide a window
interface with a location
object, including the reload()
method.
It’s a fairly simple method, as you can see in the example below. It takes just one argument, which is used to specify whether or not the browser should completely reload the contents of the page from the server or just the cache.
We’ll need to use this method within our event handler to successfully reload a page. Let’s look at an example:
class App extends Component {
render() {
return <button onClick={() => window.location.reload()}>Refresh</button>;
}
}
It’s a basic example of refreshing the page manually in React. Remember, the onClick
event handler should be set equal to a function definition, not the call. This way, it is only executed when the user clicks the button. You can experiment with the code on playcode yourself.
Alternatively, you could use the lifecycle methods to reload the page once mounted. Functional components don’t have lifecycle methods, but you can accomplish the same result using the useEffect()
hook.
Update the State of the Component to Refresh a Page in React
Changes to the local state of the component automatically trigger the update. It is the natural way to handle page updates in React, so it’s more aligned with official good practice guidelines from the creators of React.
If built properly, React apps don’t need to be manually refreshed to load the most recent changes in the state. This process is automatically triggered.
The entire purpose of having a local state is that any changes to the state will be automatically reflected. Let’s look at an example:
class App extends Component {
constructor(props){
super(props)
this.state = {
textInput: ""
}
}
render() {
return <div>
<input type="text" onChange={(e) => this.setState({textInput: e.target.value})} />
<h1>{this.state.textInput}</h1>
</div>
}
}
It’s a fairly simple demonstration of how state changes automatically trigger the page refresh (also called re-render) in React. We initiate the state with one textInput
property and update the property every time the value in the input
field changes.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn