How to Implement Radio Button in React
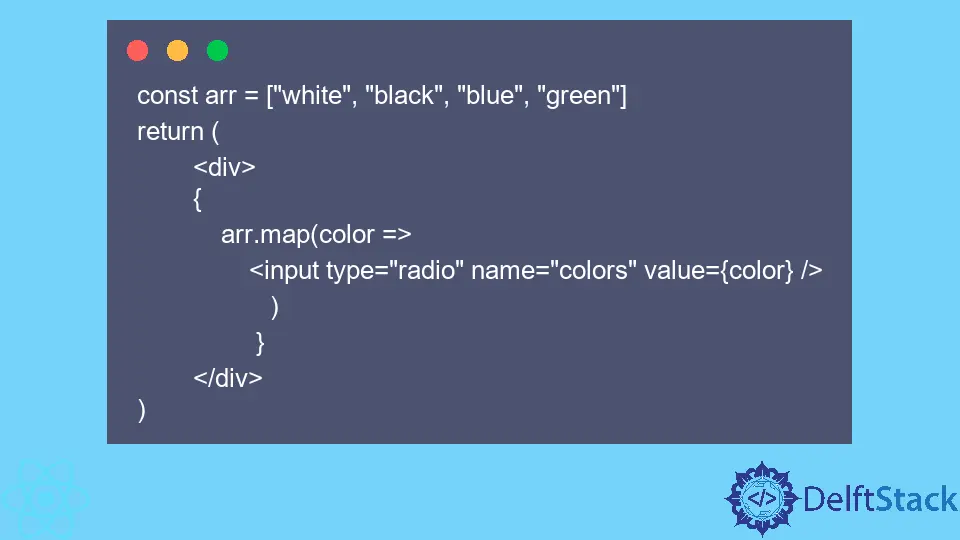
Any developer who’s ever created a simple form in any JavaScript framework should be familiar with radio buttons. They are the default <input/>
type to use when letting users choose between multiple options. Let’s look at two approaches to implementing radio buttons in React.
Radio Buttons in React
The basic implementation of radio buttons in JSX is very similar to HTML. Let’s look at an example:
<div>
<input type="radio" name="theme" value="DARK"/>Dark
<input type="radio" name="theme" value="LIGHT"/>Light
</div>
This JSX code could be easily mistaken for regular HTML. As we introduce more complex features, like updating the state after an onChange
event, the differences between HTML and JSX become more noticeable. Let’s look at this example:
class App extends Component {
constructor(props){
super(props)
this.state = {
theme: "light"
}
}
render() {
return <div onChange={(e) => this.setState({theme: e.target.value})}>
<input type="radio" name="theme" value="DARK"/>Dark
<input type="radio" name="theme" value="LIGHT"/>Light
</div>;
}
}
In this code, the users have two options: Dark
and Light
. Whenever they choose either one of them, we capture the value in the input element’s value
attribute and set the state’s theme
property equal to it. For better demonstration, look at the sample playcode app.
Notice that the event handler is placed between a pair of curly braces. It is necessary to convey that the expression between braces should be executed as JavaScript code.
As a developer, you are not limited by your choice of an event handler. You can use onChange
, onSubmit
if your radio buttons are placed within a form onClick
if you’re using buttons to submit your choice.
.map()
If you have an array of options, you can use .map()
method to render every one of them. For instance, if you have a list of colors that you’d like to allow your users to choose from, you can:
const arr = ["white", "black", "blue", "green"]
return (
<div>
{
arr.map(color =>
<input type="radio" name="colors" value={color} />
)
}
</div>
)
Curly braces within the <div>
element tell React that the expressions inside it should be executed as JavaScript code.
Once the code is executed, you’ll end up with four <input>
elements that each have a value
attribute from the array.
defaultChecked
To check a Radio button by default, you can use the defaultChecked
attribute. In the above example, if we wanted to set a default Dark theme, here’s what our element would look like:
<input type="radio" name="theme" defaultChecked value="DARK"/>Dark
Custom Radio Component
When developing React applications, you should always try to keep them simple. Also, if you’re going to use radio button functionality a lot, you can avoid code repetition by creating a custom radio component.
const Radio = ({handler, theme}) => {
return <div style={{height: "500px", width: "500px", backgroundColor: theme.toLowerCase() === "light" ? "white" : "black"}}
onChange={handler}>
<input type="radio" name="theme" value="DARK"/>Dark
<input type="radio" name="theme" value="LIGHT"/>Light
</div>;
}
class App extends Component {
constructor(props){
super(props)
this.state = {
theme: "light"
}
}
render() {
const handleChange = (e) => this.setState({theme: e.target.value})
return <div><Radio handler={handleChange} theme={this.state.theme}></Radio></div>
}
}
At the top, we created a stateless function component called Radio
. We can pass down event handlers as a prop, so there’s no real reason for this child component to initiate the state. Dumb components (those without state) are also easier to test and maintain.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn