React Proptype Array With Shape
- What are PropTypes?
- Using PropTypes.arrayOf() with PropTypes.shape()
- Benefits of Using PropTypes.shape()
- Conclusion
- FAQ
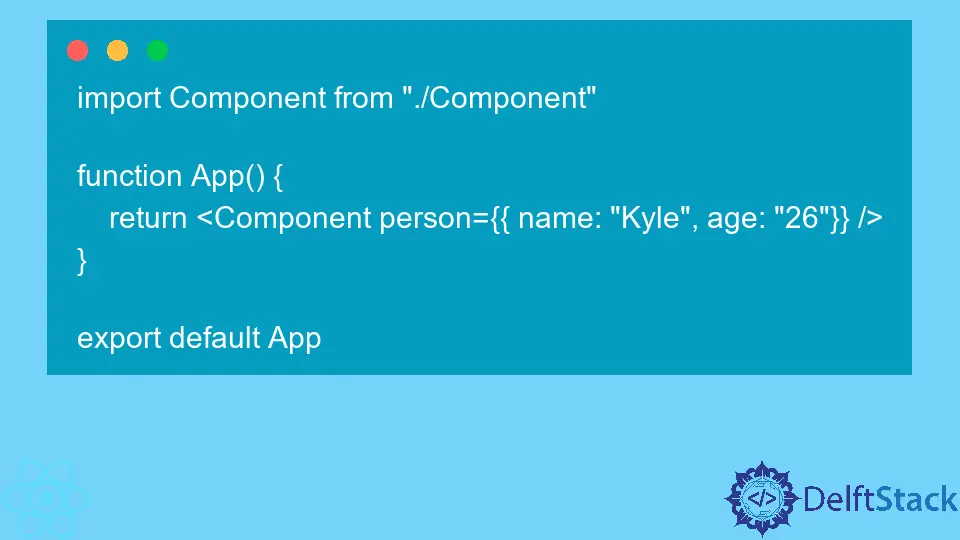
In the world of React, ensuring that your components receive the correct data types is crucial for maintaining robust applications. One of the powerful tools at your disposal is PropTypes, a mechanism that allows you to define the expected types for your props. Among these, PropTypes.shape()
is particularly useful when dealing with complex data structures, such as arrays of objects.
This tutorial will walk you through how to effectively implement PropTypes.arrayOf(PropTypes.shape())
in your React components, enabling you to catch bugs early in the development process. By the end, you’ll have a solid understanding of how to leverage this feature to enhance your code quality.
What are PropTypes?
PropTypes is a type-checking feature in React that helps you validate the types of props being passed to your components. It serves as a form of documentation, making your components easier to understand and maintain. By using PropTypes, you can catch bugs that arise from incorrect data types, leading to a more stable and reliable application.
When you define a prop type using PropTypes.shape()
, you can specify the exact structure of an object that your component should expect. This is especially useful when dealing with arrays of objects, where each object may have its own set of properties. Let’s explore how to implement this in your React applications.
Using PropTypes.arrayOf() with PropTypes.shape()
To define an array of objects with specific shapes, you can use PropTypes.arrayOf()
in combination with PropTypes.shape()
. This combination allows you to specify the expected properties and their types for each object within the array. Here’s how you can implement this in your React component:
import PropTypes from 'prop-types';
const MyComponent = ({ items }) => {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item.name}: {item.value}</li>
))}
</ul>
);
};
MyComponent.propTypes = {
items: PropTypes.arrayOf(
PropTypes.shape({
name: PropTypes.string.isRequired,
value: PropTypes.number.isRequired
})
).isRequired
};
export default MyComponent;
In this example, we define a component called MyComponent
that takes an items
prop. The items
prop is expected to be an array of objects, where each object must have a name
property (a required string) and a value
property (a required number). If the data structure passed to MyComponent
does not match this shape, React will issue a warning in the console.
This implementation not only ensures that the data is structured correctly but also enhances the readability of your code. By explicitly defining the expected shape of the data, you make it easier for other developers (or yourself in the future) to understand what MyComponent
requires to function properly.
Benefits of Using PropTypes.shape()
Using PropTypes.shape()
comes with several advantages that can significantly improve your development workflow. Firstly, it provides a clear contract for your components. When you define the expected shape of the data, it helps other developers understand how to use your component correctly. This clarity reduces the chances of bugs caused by incorrect data types.
Secondly, PropTypes act as a form of documentation. They serve as a reference for the expected props, making it easier to onboard new team members or revisit your code after a long time. This is particularly valuable in larger projects where components can become complex.
Finally, using PropTypes can help catch errors early in the development process. If someone tries to pass an incorrect data type to your component, React will provide warnings in the console. This immediate feedback allows you to address potential issues before they make it into production, saving you time and headaches later on.
Conclusion
Incorporating PropTypes.arrayOf(PropTypes.shape())
into your React components is an excellent way to enhance data validation and improve the overall quality of your applications. By defining the expected structure of your props, you can catch errors early, improve code readability, and provide better documentation for your components. As you continue to build more complex applications, leveraging PropTypes will become an invaluable part of your development toolkit. Start implementing this practice today to ensure a more robust and maintainable codebase.
FAQ
-
What is PropTypes in React?
PropTypes is a type-checking feature in React that validates the types of props passed to components, helping to catch bugs early. -
How do you use PropTypes.shape()?
You use PropTypes.shape() to define the structure of an object that a component expects as a prop, specifying the required properties and their types. -
What are the benefits of using PropTypes?
The benefits include improved code readability, better documentation, and early detection of bugs caused by incorrect data types. -
Can PropTypes validate arrays?
Yes, you can use PropTypes.arrayOf() in combination with PropTypes.shape() to validate arrays of objects with specific structures. -
Is using PropTypes mandatory in React?
No, using PropTypes is not mandatory, but it is highly recommended as it helps maintain code quality and reduces errors.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn