One-Way Data Binding in React
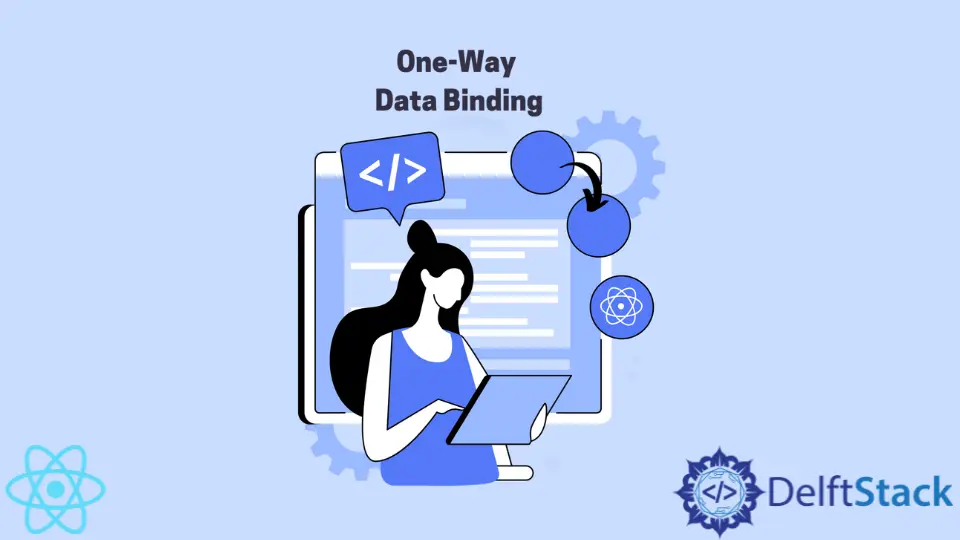
Currently, front-end developers’ have three choices for building web applications - React, Vue, and Angular JavaScript frameworks. These frameworks share many similarities but are also different in important ways.
In this article, we will discuss one-way data binding in React. This is one of the distinguishing features of React library, which influences the structure and functionality of React web applications.
One-Way Data Binding in React
Unlike other JavaScript frameworks, React does not have a feature to tie the value of the DOM input element with a state variable. Entering new characters into the element does not automatically update the state variable.
The structure of React components is usually written in JSX, which has HTML-like syntax with added dynamic features.
To update the state in response to the user’s actions, you need to define an event handler and set the attribute to execute the event handler in response to the user’s actions.
export default function App() {
const [value, setValue] = useState("");
return (
<div className="App">
<input type="text" onChange={(e) => setValue(e.target.value)} />
<h2>{value}</h2>
</div>
);
}
In this example, the user’s actions trigger the onChange
event handler, which updates the state variable. Then you can display this value as an <h2>
tag.
One-Way Data Binding vs. Two-Way Data Binding in React
Comparison of React, Angular, and Vue, which both have two-way data binding.
You can think of two-way binding as setting up a two-way connection between an input (DOM element) and data so that whenever one updates, another also updates.
For example, in Vue, you can tie a value of the input element to a state value. Changing it will change the value in the input element as well.
Also, entering more characters into the input element will change the state value.
In React, there is no way to set up a direct relationship between the value of the input DOM element and the state. You can set up event handlers to update the state whenever it detects new input from the user.
Typically, changing the state does not change the value in the <Input>
element.
Let’s look at this example.
import "./styles.css";
import { useState } from "react";
export default function App() {
const [value, setValue] = useState("");
return (
<div className="App">
<input type="text" onChange={(e) => setValue(e.target.value)} />
<h2>{value}</h2>
<button onClick={() => setValue(value.concat(value))}>
Double the Text
</button>
</div>
);
}
Here, we have an <input>
element whose internal value is not tied to a state variable. However, every time user enters a character, React executes the event handlers and sets the state variable value
equal to the user input.
This is demonstrated when we output the state variable as the <h2>
header.
We also have a button labeled Double the Text
. Clicking this button executes another function, which updates the state variable by adding it.
Go to this CodeSandbox demo to better understand the code. When you use the button to change the state value, it does not affect the input element.
The reason is that React does not have two-way data binding between the input element and state variable.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn