React.memo and Its Uses
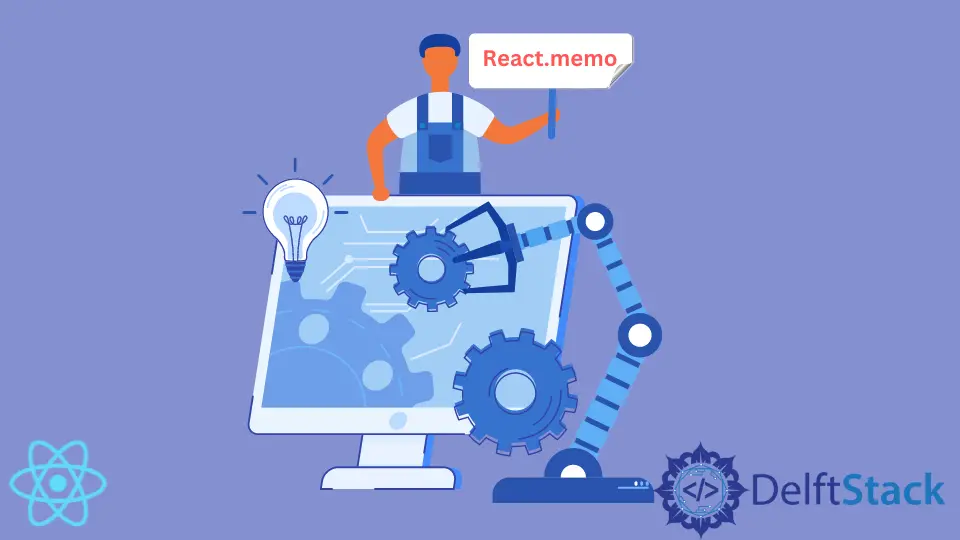
React is popular because it allows developers to build dynamic applications.
However, the default behavior of React components is inefficient. The library includes PureComponent
for Class Components and React.memo
HOC for functional components to address this issue.
These solutions can reduce the number of times components are updated and make React applications much more efficient. This article will explore what React.memo
does and how to use it.
What Is React.memo
React.memo
is a higher-order component, which wraps around normal function components in React.
If the component does not have any side effects and renders the same output with the same props, React.memo
can improve the speed and performance of your component.
The app wrapped in the React.memo
higher-order component, and all its children, will only re-render if the props change. The child components in the tree will also re-render if their local state or context changes.
Here’s an example of a component wrapped in React.memo
HOC:
const App = React.memo(() => {
return <p>Hello World</p>;
});
Any component tree under the App
component will also be influenced. Here’s a link to the playcode.
What React.memo
Does
All components in React have a default shouldComponentUpdate()
method, which triggers re-renders every time their own or parents’ props change.
Wrapping a component in React.memo
HOC can change this default behavior. Once wrapped, the shouldComponentUpdate()
method will change, and the component and all of its children will only re-render if the local state or props change.
The method will perform a shallow comparison of the state and props to determine when to re-render. If you look at the order in which lifecycle methods are called, shouldComponentUpdate()
always comes before the render method, as it should.
So the shouldComponentUpdate()
will be called every time the props change. But the component and the children will only re-render if the shallow comparison determines that their props have changed.
Performance Gains
By reducing the unnecessary updates, memorizing (wrapping a React component in React.memo
HOC) can significantly improve your app’s performance.
You can wrap your component in React.memo
, or you can selectively wrap some branches of the component tree. You can use profiling tools to find if there are significant enough inefficiencies in your app to warrant the use of React.memo
.
As a rule of thumb, making the shallow comparison on props
objects is always cheaper than calling React.createElement()
and comparing entire component trees.
However, if your component has deep comparing props, the shallow comparison of React.memo
will be insufficient. Also, this higher-order component does not work if your component receives and displays children
prop values.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn