How to Set Media Queries in React Without CSS
- Media Queries in React
- Create Class Components to Set Media Queries in React Without CSS
- Create Functional Components to Set Media Queries in React Without CSS
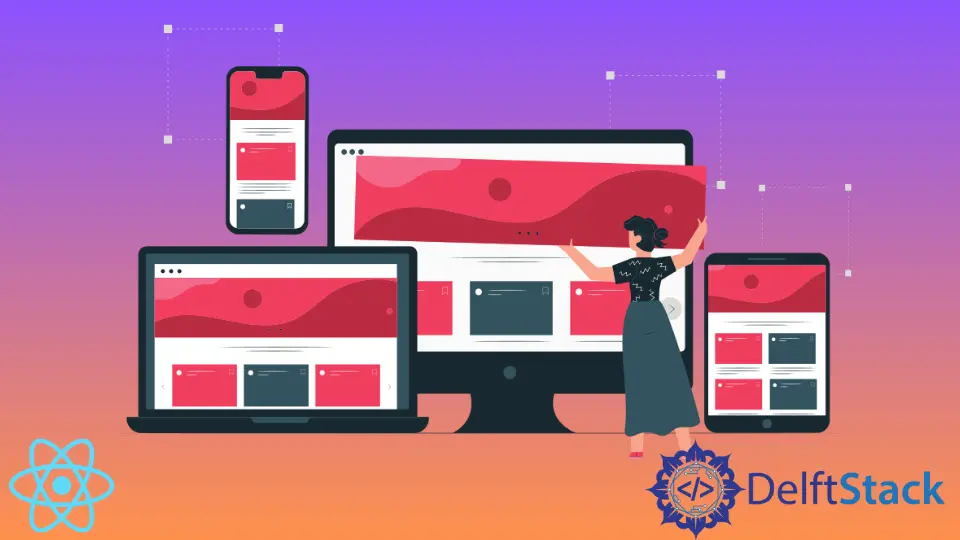
In 2022, users who browse the internet are much less predictable than 20 or even 10 years ago. Now, most people browse the internet on their smartphones and expect a much better user experience than they used to before.
Customers are also flooded with options, so businesses have to try harder to design the best possible websites to get their attention.
Media Queries in React
Since users visit websites from devices with different screen sizes, web developers need to find a way to adjust website design accordingly. Using media queries is the primary way to ensure the responsiveness of your application.
React is the most popular JavaScript-based library for building fast single-page applications. If you’ve worked with DOM and built applications using HTML, CSS, and JavaScript, you shouldn’t have a hard time transitioning to working in React.
React uses JSX, a templating language similar to HTML. In many ways, it works like HTML, but there are significant differences; for instance, setting inline styles works differently in JSX.
As you may know, media queries are relatively easy to implement in CSS. However, for some reason, if you want to implement media queries without CSS, it can be achieved using plain JavaScript in React.
Create Class Components to Set Media Queries in React Without CSS
Class components were the primary way to have state
functionality in React for a long time. The state
must make your React component respond to the user’s actions.
Let’s see how we can set the media queries in React class components without further ado.
Example:
import React, { Component } from "react";
class App extends Component {
constructor(props) {
super(props);
this.state = {
screenSizeFits: window.matchMedia("(min-width: 768px)").matches
};
}
componentDidMount() {
const adjustScreenSize = (e) =>
this.setState({ screenSizeFits: e.matches });
window
.matchMedia("(min-width: 768px)")
.addEventListener("change", adjustScreenSize);
}
render() {
return (
<div>
{this.state.screenSizeFits && <h1>Laptop</h1>}
{!this.state.screenSizeFits && <h3>Smartphone or Tablet</h3>}
</div>
);
}
}
export default App;
We have an App
component with one state
property called screenSizeFits
. It is initialized to a boolean value, which we get by using the window.matchMedia()
method.
We use the componentDidMount()
lifecycle method to add an event listener and update the state. We add an event listener to the window
object so that every time the screen resolution changes, we update the state
variable accordingly.
If the screen resolution is larger than 768 pixels, the screenSizeFits
property will be set to true
. If it’s smaller, the property will be set to false
.
Finally, we use the simple &&
logical connector to display the relevant text based on the state
property value.
You can check out this live demo to see how it works. Try making the screen size larger or smaller, and see how the text changes accordingly.
Every time you change the screen resolution, the component will hire an event listener and update the state
accordingly.
Create Functional Components to Set Media Queries in React Without CSS
Since React v16.8, you can use hooks to add interactive features to functional components. The React community came up with the react-media-hook
library to help developers easily manage media queries in functional components.
You can install the package by entering this command:
npm i react-media-hook --save
Then, you can import the useMediaPredicate
hook directly into your application and start conditionally styling your application based on the screen size of the user’s device.
Example:
import React from "react";
import { useMediaPredicate } from "react-media-hook";
const App = () => {
const moreThan720 = useMediaPredicate("(min-width: 720px)");
return <div style={{border: moreThan720 ? "2px solid red" : ""}}>
<button>SomeButton</button>
</div>
};
In this example, the variable moreThan720
will store a boolean value, depending on whether or not the argument we pass to the useMediaPredicate
hook is true
.
Then, we use the ternary operator to set inline styles conditionally. If the window width exceeds 720px, we will set a border property to the following value: 2px solid red
; if not, there will be no border.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn