How to Map an Array of Objects in React
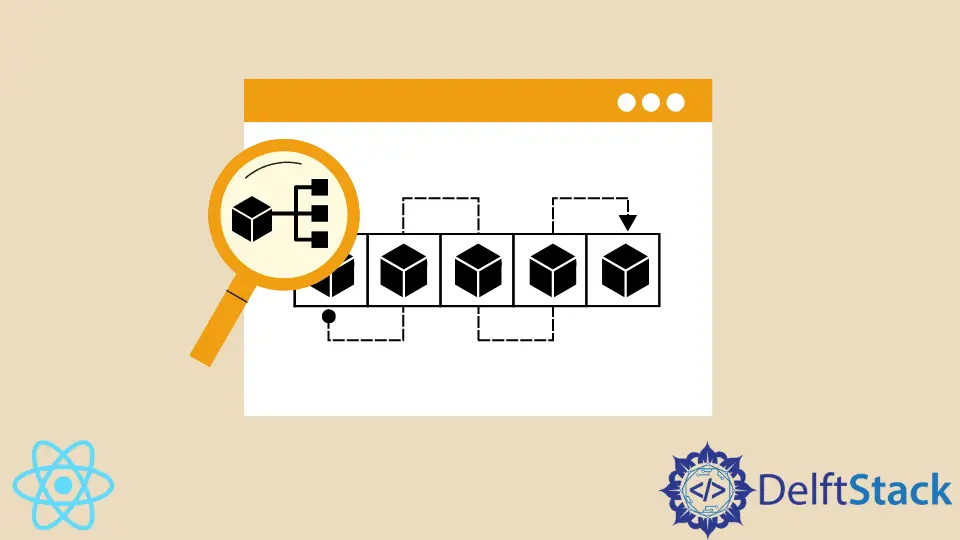
One of many great features of the React library is that it is highly dynamic and can dynamically render and not render certain elements.
Often, web developers have large volumes of data and want to display it on the screen, and usually, it is formatted as an array of objects. This article will show how to map an array of objects in React and discuss possible use cases for this operation.
Use the map()
Method to Map an Array of Objects in React
React library is founded on JavaScript so that you can use methods like map()
to go over an array of objects. External data is often stored as an array of objects; hence, in developing web applications in React, you’ll often have to map an array of objects.
There are several ways to loop through an array in JavaScript. You can use the for
loop, the forEach()
method, or even the while
loop.
Every web developer prefers to use map()
because you can go over an array of objects and generate new React elements or components within JSX.
JSX is a special HTML-like syntax for building the layout of React components. It allows you to embed JavaScript expressions within what looks like your HTML code.
This makes it easy to dynamically generate React elements, components, and values. Other ways of iterating through an array are not supported within JSX, map()
is the only supported method.
Let’s see an example of how to map an array of objects in React:
export default function App() {
const arr = [
{ name: "Irakli", height: 180 },
{ name: "Chris", height: 190 },
{ name: "Michael", height: 176 },
{ name: "John", height: 182 },
{ name: "Todd", height: 198 },
{ name: "Jack", height: 178 }
];
return (
<div className="App">
{arr.map((person) => (
<div
style={{
border: "2px solid black",
margin: "30px auto",
width: "30%"
}}
>
<p>name: {person.name}</p>
<p>height: {person.height}</p>
</div>
))}
</div>
);
}
In this example code, we have a simple array of objects. Each item in the array represents one person.
You can check out the live demo on CodeSandbox and see what the map()
method renders.
Within JSX (in the return
statement), we used the .map()
method to go over every item in the array.
The map()
method takes one argument - a callback function that will be executed on every item in the array. The callback function takes one argument for every item in the array.
In this case, the method will go through the entire array of objects, and each item in the array will be used as the person
argument. As you can see, we take the person
argument’s name
and height
properties and use these to generate values for two <p>
elements in the output.
Don’t forget that to write JavaScript expressions (like executing a map()
method) in JSX, you must wrap them in curly braces.
Ultimately, the map()
method will output a new array, which transforms an array of objects into an array of React elements (or components).
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn