How to Use jQuery in React Properly
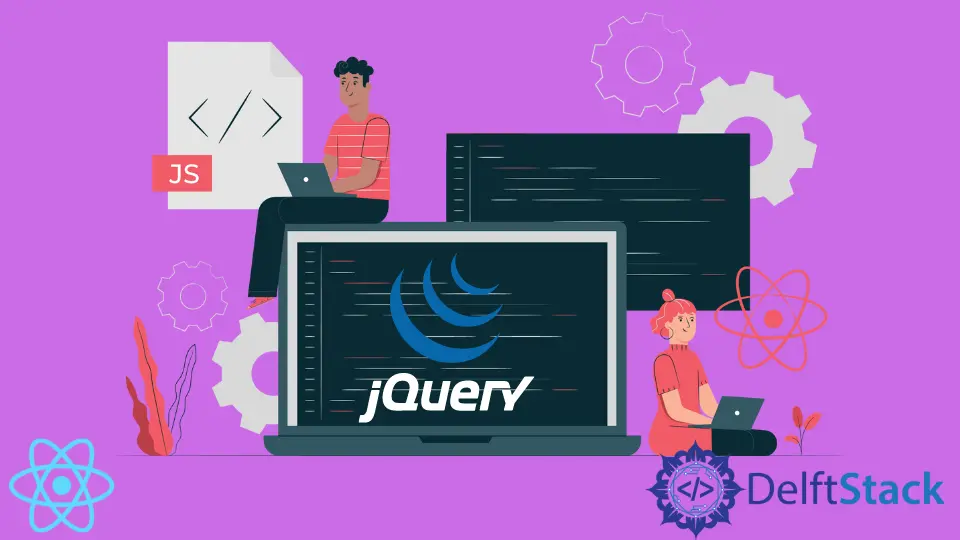
jQuery is one of the most known libraries used by JavaScript developers.
The library provides an easy way to perform DOM manipulation and animation, to name a few. Because of its popularity, many web developers are familiar with it and prefer to use jQuery when they can.
React is another popular library developed by Facebook. In the past few years, it earned the title of the most widely used JavaScript framework.
It is arguably more modern and has more features than jQuery. While you can use both libraries in a single application, there are rules for using jQuery within React applications.
Can You Use jQuery in React
It is possible to use jQuery in a React app, as long as you use it properly and choose its placement carefully. Similarly, you can embed React into jQuery.
What Are the Limitations of jQuery in React
The main limitation of using jQuery in React is that jQuery manually updates the DOM. On the other hand, React has its system for making changes to the DOM.
It internally determines when a React app or component should re-render. It also figures out which parts of the UI need to be updated.
Therefore, when you use jQuery to manipulate the DOM, React gets confused. That’s why, under normal circumstances, it is considered a bad practice to use jQuery in React, but there are exceptions.
the Right Way of Using jQuery in React
First, you need to install the jQuery
library and then import it, like this:
import $ from 'jquery';
As we already mentioned, jQuery and React are two libraries concerned with DOM manipulation. To make sure that jQuery doesn’t interfere with React, we need to create a component that never gets updated by React.
How can we do this? As you may know, React components re-render when their state
or props
change. So we have to build a component that doesn’t have any state
or props
.
Let’s look at an example:
class App extends Component {
componentDidMount(){
$("p").hide()
}
render() {
return <div>
<p>Hi! Try edit me</p>
</div>;
}
}
To ensure that React doesn’t ever update the DOM for the App
component, we do not initiate any state or use any prop
values. Our return
statement consists of the static wrapper and paragraph elements.
This way, we can clear the way for the jQuery plugin to manipulate the DOM and hide all the paragraph elements directly.
Another good way to select DOM elements directly is to use a ref
.
Should You Use jQuery in React
React has built-in features that allow you to manipulate the DOM in most cases. Working within the framework of React components enables you to control the behavior and appearance of your application.
If you are sure you need to use jQuery and stay within the limitations stated above, you are free to do so. However, jQuery has a limited set of applications within React.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn