How to Use forwardRef in React With TypeScript
-
What Are
refs
in React -
Why Do We Need
forwardRef
in React -
the
forwardRef
in React and TypeScript -
Use
forwardRef
With TypeScript
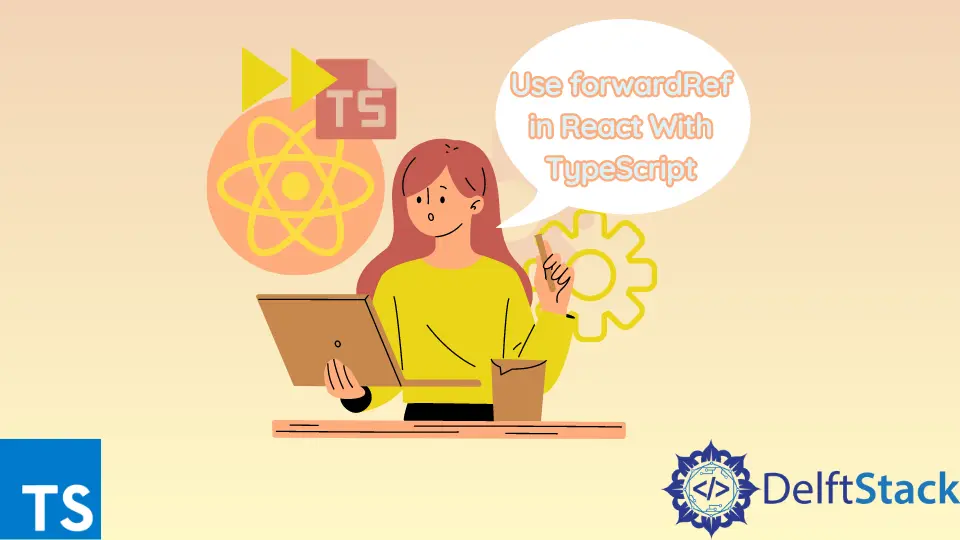
In recent years, more and more developers have been using React and TypeScript together. Job descriptions can further confirm that using TypeScript with React is not a fad but the present and the future.
Web developers prefer to write TypeScript to make code more organized, reduce room for errors and save time on debugging.
This article discusses forwarding a ref from a child component to the parent.
What Are refs
in React
Usually, DOM React element’s appearance changes in response to changes in state
or props
objects. However, React still allows you to capture specific DOM elements and imperatively modify them when necessary.
Refs in React (short for references) allow developers to access and manipulate DOM elements.
Since React 16.8, React developers can use the hook useRef
to create hooks. Here is an example.
function App() {
let inputRef = useRef();
return (
<div className>
<input ref={inputRef} type="text" />
</div>
);
}
Here, we create a variable inputRef
and initialize it as a ref
.
Then we find the <input>
element in JSX and set its ref
attribute to the variable we created earlier. Now the reference to this element is stored in the variable.
Refs are often used to directly animate DOM elements. They commonly highlight borders when the element is focused or selected or imperatively trigger animations.
Why Do We Need forwardRef
in React
Usually, we access references in the same component where we create them.
However, sometimes you need to define a ref
for one of the DOM elements in the parent component but pass it down to the child component. That’s why you need the forwardRef
feature.
the forwardRef
in React and TypeScript
Sometimes we need to access an element from a child component in the parent component. For example, let’s say we have a <Message>
component that contains a <div>
element, and we want to access it from the parent <Messenger>
component.
function Message({messageText}) {
return <div>{messageText}</div>;
}
Maybe we want to highlight the box when it is in focus. The only way to do that is to create a ref
in the parent component; then, we can check if the element is in focus and highlight it conditionally.
function App() {
const input = React.useRef<HTMLElement>(null);
React.useEffect(() => {
if (input.current) {
input.current.focus();
}
}, []);
return <Message messageText="hello world" ref={input} />;
}
However, this will throw an error because a parent component cannot directly access an HTML element contained in the child component. Instead, we use forwardRef
.
When defining the child component, we need to make the following changes.
const Message = React.forwardRef((props, ref) => {
return <div ref={ref}>{props.messageText}</div>;
});
The forwardRef
function from React will make our <div>
element available to the parent. We wrap our entire component in the forwardRef
function.
It returns a component, which we can store in the Message
variable.
This time, the parent component will access the child’s elements.
Use forwardRef
With TypeScript
In the previous example, when we create a ref
instance and store it in the input
variable, we specify its type - <HTMLElement>
.
function App() {
const input = React.useRef<HTMLElement>(null);
...
return <Message messageText="hello world" ref={input} />;
}
The <HTMLElement>
is the most generic type for DOM elements in React. If you were referencing a <div>
element, you could use a more specific type like <HTMLDivElement>
.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn