How to Validate React Form
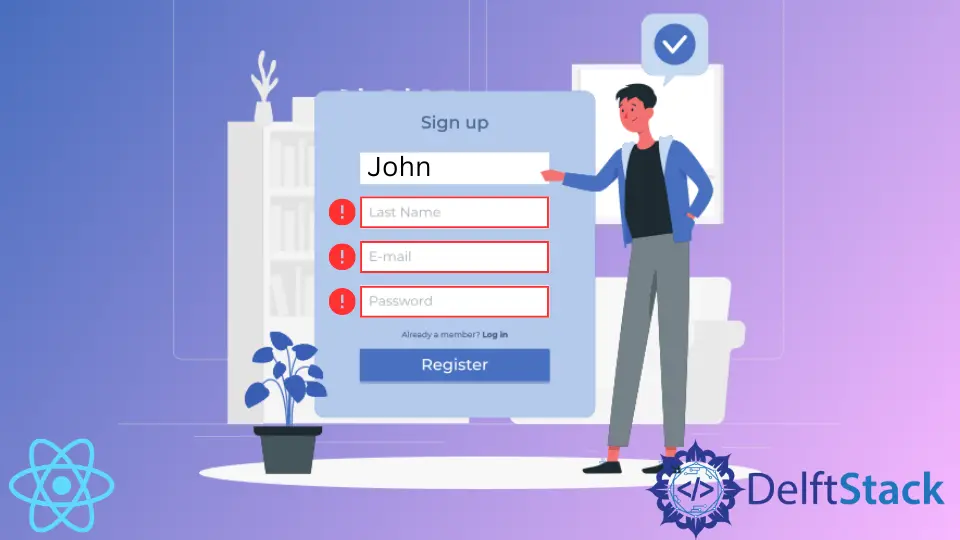
In our time, the companies store, maintain and analyze the data for many different purposes.
Whether it is for maintaining a user database, generating reports, or anything else, the role of data can not be overstated. Thus, data need to be structured and well-organized.
Web applications powered with React must use form validation to ensure that the user inputs follow standard conventions. This way, you can facilitate the user journey and collect the data in an organized manner.
Poor data validation can also lead to malicious attacks, so implementing proper solutions can also increase the safety of your applications.
React Form Validation
For proper React applications, form validation is an essential feature. For instance, an email must contain the @
sign, a valid suffix, and meet other criteria.
onChange
Event
The most effecient way to implement form validation in React is by listening for onChange
events and maintaining user inputs in the state
of your component. First, you must have controlled inputs, so if you have two fields to validate (let’s say, name and email) you need to initialize the state with the following empty values:
constructor(props){
super(props)
this.state = {
inputFields: {"name": "", "email": ""},
errorMessages: {}
}
}
The state must be initialized in the constructor()
function when writing class components. It is also good to have an errorMessages
property, where you can store the messages to tell users what’s wrong with the entered values.
The first step in the form validation should be to set up <input>
elements with onChange
event listeners. The changes in the input fields will be automatically reflected in the state as well.
Our render()
method should look like this:
render() {
return (
<form>
<input type="text" onChange={(e) => this.setState({inputFields: {"name": e.target.value, "email": this.state.inputFields.email}})} />
<br />
<input type="text" onChange={(e) => this.setState({inputFields: {"name": this.state.inputFields.name,"email": e.target.value, }})}/>
</form>
)
}
This is a great start; still, we need to set up conditions for form validation and generate error messages. For that, we create a custom validate()
function.
For the sake of simplicity, we’ll set up two basic rules: The name should be longer than 2 letters and the email value should contain @
. Now, our entire application should look something like this:
class App extends Component {
constructor(props){
super(props)
this.state = {
inputFields: {"name": "", "email": ""},
errorMessages: {}
}
}
render() {
console.log(this.state)
const validate = () => {
let inputFields = this.state.inputFields
let errors = {}
let validityStatus = true
if (inputFields["name"].length <= 2){
validityStatus = false
errors["name"] = "The name should contain more than two symbols"
}
if (inputFields["email"].indexOf("@") === -1 ){
validityStatus = false
errors["email"] = "The email should contain @"
}
this.setState({errorMessages: errors})
return validityStatus
}
const handleSubmit = (e) => {
e.preventDefault();
if (validate()){
alert("form complete")
}
else {
alert("errors")
}
}
return (
<form onSubmit={(e) => handleSubmit(e)}>
<input type="text" onChange={(e) => this.setState({inputFields: {"name": e.target.value, "email": this.state.inputFields.email}})} />
<br />
<input type="text" onChange={(e) => this.setState({inputFields: {"name": this.state.inputFields.name,"email": e.target.value, }})}/>
<br />
<button type="submit">Submit</button>
</form>
)
}
}
Once the form is submitted, our validate()
function checks the values entered into the fields and returns true
if all the conditions are met and false
if they’re not. Once the check is over, the handleSubmit()
function alerts either form complete
or errors
.
The function also includes local the errors
variable, storing the warning messages for each text field. Once the check is done, it references the variable to update the errorMessages
state property.
For more convenience, under each field, you can add a text which will display the error message for that field. Here’s an example:
return (
<form onSubmit={(e) => handleSubmit(e)}>
<input type="text" onChange={(e) => this.setState({inputFields: {"name": e.target.value, "email": this.state.inputFields.email}})} />
<p style={{color: "red"}}>{this.state.errorMessages.name}</p>
<br />
<input type="text" onChange={(e) => this.setState({inputFields: {"name": this.state.inputFields.name,"email": e.target.value, }})}/>
<p style={{color: "red"}}>{this.state.errorMessages.email}</p>
<br />
<button type="submit">Submit</button>
</form>
)
This way, you can use the warning messages in your errorMessages
state property to give users helpful minor warnings to help them fix the errors.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn