Applications of the forEach() Method in React
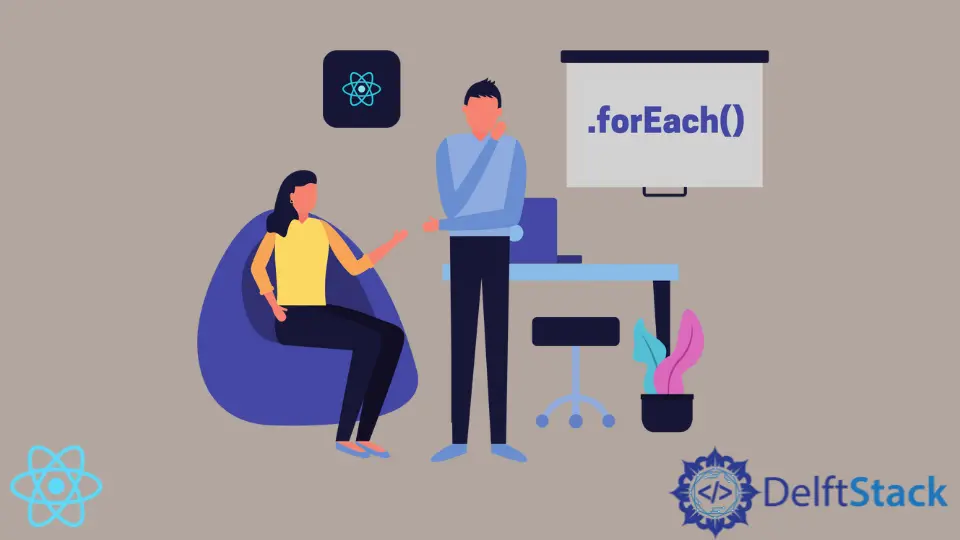
The introduction of the Modern ES6 version of JavaScript has brought us many useful features. The .forEach()
method is just one example.
Like you might’ve guessed from the name, the .forEach()
method executes a callback function on every item in the array. This is done to transform every item in the array in one way or another.
In React, we often render components based on a list. For instance, it is not uncommon to have a list of usernames and need to display every one of them as paragraphs.
In the process, you can also transform them in another way, such as capitalizing them.
Array Items to Elements in JSX
JSX is a familiar HTML-like syntax that reduces the effort of structuring our components. Ultimately, the code written in JSX compiles into a complex set of React.createElement()
method calls.
To use .forEach()
or other JavaScript methods within JSX, you must place the expressions between curly braces. This is necessary to let React know to treat everything between curly braces as regular JavaScript.
.forEach()
in React
In pure JavaScript, .forEach()
is very useful for changing every array item.
It is less useful if you’re trying to render components based on a list in React. There are multiple reasons why React developers don’t use .forEach()
to render components.
If you want to use curly braces to render multiple components based on a list, the expression between the curly braces should return a list of components.
If you try to use .forEach()
for this purpose, you’ll find out that nothing renders. This happens because .forEach()
returns undefined.
By nature, .forEach()
is not designed to return a value. Its primary purpose is to transform every array element, but nothing else. The method doesn’t return a new array; it returns undefined
.
Instead, React developers use an alternative method, .map()
.
.map()
as an Alternative
ES6 also introduced the .map()
method, which is better suited to render components based on a list.
Like .forEach()
, it takes one argument - a function that specifies how to transform every item in the array. However, unlike .forEach()
, the map method leaves the original array and returns a new one containing the transformed items.
This is just what you need to render multiple components based on an array.
Let’s look at an example:
class App extends Component {
render() {
return <div>{[1,2,3].map(number => <h1>{number}</h1>)}</div>;
}
}
In this example, we got an array of numbers that we .map()
to create a new array. The new array contains three <h1>
elements with the three number values from the original array.
When using the .map()
method, giving each new element a distinct key
prop is also a good idea. React uses the key
value to identify a unique element.
Here’s how the above code block would look like after following this pattern:
class App extends Component {
render() {
return <div>{[1,2,3].map(number => <h1 key={number}>{number}</h1>)}</div>;
}
}
Since the numbers in our array are unique, we can use their value as a key
.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn