Multiple Approaches to Formatting Date in React
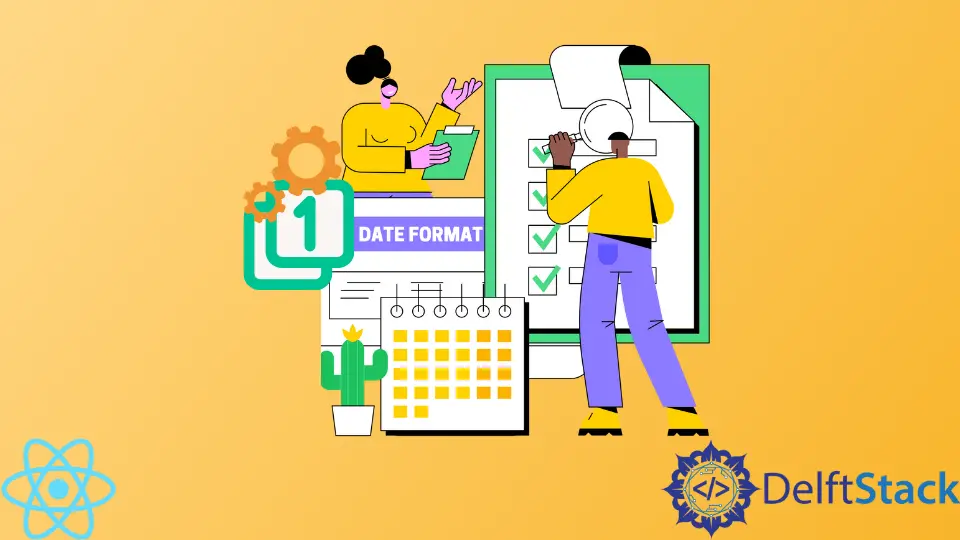
When building React applications, developers have to find a way to work with dates.
This involves formatting, parsing, and manipulating the date values. It’s hard to think of any React app that doesn’t involve dates in one way or another.
The problem with dates in programming is that values aren’t always formatted or classified the way you want them. Sometimes date values are available as strings, arrays, or even objects.
Other times they only include year, while other date values include everything from years to milliseconds. JavaScript includes the Date
object to solve this problem, which provides a few good methods for managing data.
However, it is still insufficient for more complex needs, such as internationalization and adding/subtracting time values.
Developers solved this problem by creating numerous libraries for working with dates in JavaScript. Even the most experienced web developers choose to use external libraries to interact with Date
API directly.
These external packages provide a simpler API for formatting, parsing, and manipulating the date. They conserve JavaScript developers’ energy and time spent on managing dates.
The most popular libraries are moment, date-fns
, and luxor
, to name a few.
Date Object in JavaScript
The Date
object in JavaScript is not completely useless. It provides a few useful methods for simple operations, such as .getDay()
, which returns the day of the week of a specific date value.
The Date
object can be useful if you know all the caveats and intricate details of every method. Compared to external libraries, the biggest disadvantage of the Date
object is that it is mutable.
This feature can cause inconsistencies on your website. For example, it’s easy to accidentally overwrite mutable date values and end up displaying the wrong date without knowing.
Here are the most useful methods available for Date
objects:
.toDateString()
: converts the date value to a verbal string, which is much more readable. For example, today’s date will be formatted as the'Fr Dec 17, 2021'
string..getDay()
: returns the day in a week. If called today, it would return'Friday'
.
Read the official documentation on MDN to gain more in-depth knowledge of the Date
object and all its associated methods.
Formatting in React Using the date-fns
Library
Date libraries, such as moment
or date-fns
, are the simplest solutions for managing dates in React.
There is also the react-moment
library, and it provides a React component with all the features of its sister library. These libraries are great solutions for handling date and time in React applications.
First, you must install the date-fns
package by entering the following command in the shell:
npm install date-fns
Once installed, you can import the library.
One of the great things about the date-fns
package is modular. You don’t have to import the entire package, just the functions you need.
This is an extremely lightweight approach and ensures the high performance of your app. Here’s an example of how to format a date using date-fns
:
import "./styles.css";
import {format} from 'date-fns'
export default function App() {
return (
<div className="App">
{format(new Date(), "'We are currently in' MMMM")}
</div>
);
}
As you can see on codesandbox, the final output reads: We are currently in December
.
This is a simple demonstration of the utility of the date-fns
library and its formatting power. In this case, we use a modular approach and only import the format
function.
Then, we call it inside our JSX, between curly braces, so that the call to the function is interpreted as normal JavaScript. Curly braces also allow us to get an instance of the Date
object, which is the first argument for the format()
function.
In the second argument, we specify the text we want to output and how we want to format it. In this case, we chose MMMM
formatting, which returns the month’s full name.
We could’ve chosen MMM
, and the output would be We are currently in Dec
. This is just a simple demonstration of this package’s formatting abilities.
To get a fuller picture, check out the documentation for their format()
function.
the Advantages of Using date-fns
for Formatting in React
The library has a number of advantages over its alternatives. We already mentioned its modular approach, which further increases its speed.
The library provides a layer of abstraction to work with Date
objects, so it is familiar and doesn’t reinvent the wheel. It supports many time zones and languages.
date-fns
has a straightforward API. Most importantly, it comes with informative documentation, which explains use-cases in detail.
We’ve already demonstrated that it works very well with React. The library also works wonderfully with TypeScript and Flow.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn