How to Implement Copy-To-Clipboard Feature in React
-
Copy to Clipboard in React Using
e.clipboardData.setData()
Method -
Copy to Clipboard in React Using
navigator.clipboard.writeText()
-
Copy to Clipboard Using
react-copy-to-clipboard
Package
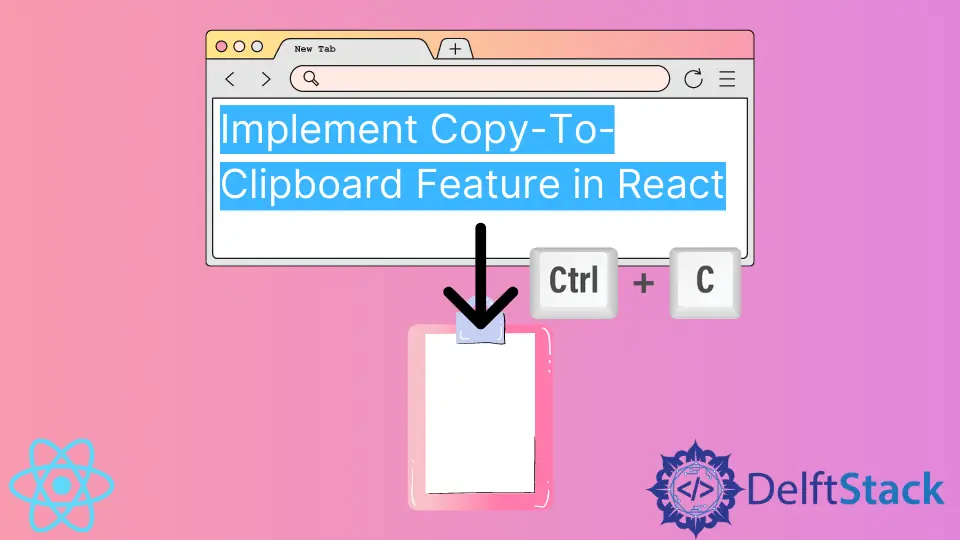
Copy and paste are universally popular features used across all kinds of devices and operating systems.
When developing an application, sometimes you can predict that a specific value, for instance, a piece of text, will need to be copied. In these situations, you can implement a button that automatically copies it. This is useful because people who use phones might sometimes struggle with text selection.
ReactJS is optimized to allow the development of user-friendly applications. The framework makes it easy to implement a clickable button (or link) that copies a piece of text.
Copy to Clipboard in React Using e.clipboardData.setData()
Method
This method provides an easy way to listen to events and copy a text once they occur. Your handler will need to receive a reference to the browser event.
In React, this will be an instance of SyntheticEvent
, referenced simply as e
. Let’s look at a simple example:
class App extends Component {
constructor(props){
super(props)
this.state = {
text: "This is a sample text"
}
}
render() {
const handleCopy = (e) => {
e.preventDefault()
e.clipboardData.setData("Text", this.state.text)
}
return (
<div>
<p>{this.state.text}</p>
<button onClick={(e) => handleCopy(e)}>Copy Text</button>
</div>)
}
}
In this example, we have a text value stored in the text
state property. Then, once the button is clicked, we execute the handler, which uses the e.clipboardData.setData()
method to push the text into the clipboard.
The method takes two arguments: the type of copied value and the value itself.
According to caniuse.com, 95% of the people browsing the internet worldwide use the browser that supports this feature. So it is safe to use.
Copy to Clipboard in React Using navigator.clipboard.writeText()
The Navigator
interface is a more modern API. It has almost the same support (92%) for users all around the world. It is compatible with both - Functional and Class components.
Let’s look at an example:
class App extends Component {
constructor(props){
super(props)
this.state = {
text: "This is a sample text"
}
}
render() {
return (
<div>
<p>{this.state.text}</p>
<button onClick={() => navigator.clipboard.writeText(this.state.text)}>Copy Text</button>
</div>)
}
}
As you can see, this is a simpler solution that requires fewer lines of code. It doesn’t require you to import any values or install external packages.
For this reason alone, for me, the Navigator
interface is the better way to copy text to a clipboard. You can try it out yourself on playcode.
Before using the Navigator
interface, you should remember that older versions of Internet Explorer do not support it. Also, in Chrome, it seems like the Navigator
interface only works as long as the page is marked as secure (HTTPS or localhost).
Besides these two points, there are no drawbacks to using this method.
Copy to Clipboard Using react-copy-to-clipboard
Package
If you’re not against installing an external package, react-copy-to-clipboard
is probably your best solution. To get in-depth information about different features, take a look at its official npm page.
Once installed, you’ll gain access to the custom <CopyToClipboard>
component. You can use its text
attribute to provide a value that needs to be copied. Then you can add a simple <button>
component, which will act as a trigger to copy the text value to the clipboard.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn