How to Decide Between Context API and Redux
-
Use the
Context API
in React -
Use the
React.createContext()
in React -
Use the
useContext()
Hook in React - Use the Redux Library in React
- When to Use the Context API vs. The Redux in React
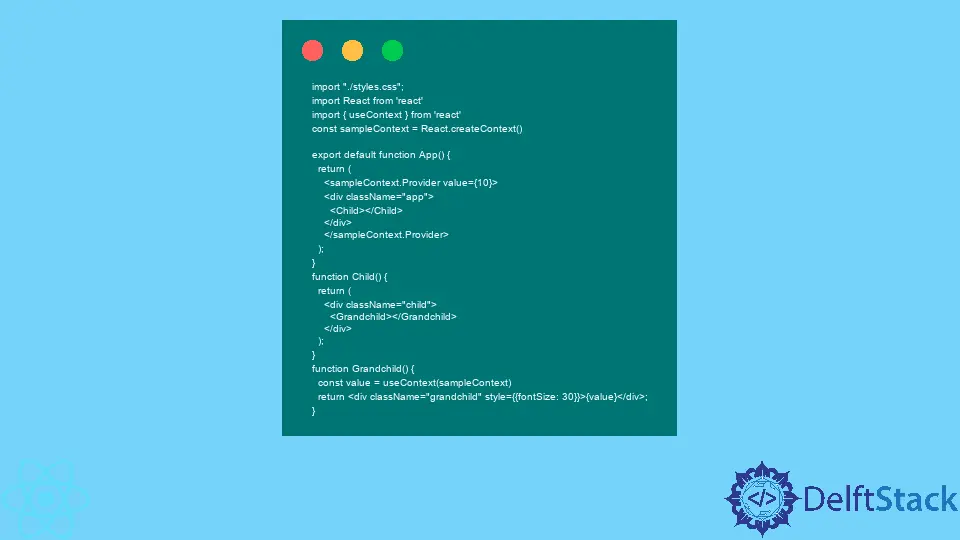
React
is one of the most popular JavaScript frameworks because it creates reusable UI components
for web applications. It is easy to integrate with other libraries and offers a lot of flexibility.
It is common for React applications to have one parent component and many children components. The default way to pass down data from a parent component to the children is called props
.
This feature is essential for the reusability of components, which are building blocks of React applications. Each component accepts data necessary to perform specific actions and can have child components and use props
to pass down the data.
If your React application has a simple component tree, you can use props
to manually pass down the data. However, the component tree
becomes more complex as the application grows.
At some point, the child components
will have child components
of their own. It’s not uncommon to find component trees with a complex component hierarchy
.
Use the Context API
in React
The Component trees
for complex applications can be challenging to traverse. In this case, it’s not practical to manually pass down the props
through every branch of the tree.
The Context API
is a solution for passing down props from a parent component
at the top of the tree to its children components
.
You can use Context API
, regular props
, and Redux
to pass down the props. The key is to know the advantages of each and make the best use of them.
Context API
helps pass down specific props
required in most components on different tree branches. For instance, values like locale preference are needed in most components of React applications.
You can technically pass down the data using props
, but navigating the component tree and its many branches would take too much time.
Instead, you can use Context API to pass down the data from a parent component
straight to the children on the lower levels of the component tree.
Let’s look at a simple example:
import "./styles.css";
import React from 'react'
import { useContext } from 'react'
const sampleContext = React.createContext()
export default function App() {
return (
<sampleContext.Provider value={10}>
<div className="app">
<Child></Child>
</div>
</sampleContext.Provider>
);
}
function Child() {
return (
<div className="child">
<Grandchild></Grandchild>
</div>
);
}
function Grandchild() {
const value = useContext(sampleContext)
return <div className="grandchild" style={{fontSize: 30}}>{value}</div>;
}
Here, we have three components: the App
component, which sits at the top of the tree, the Child
component, and the Grandchild
component.
The Grandchild
component, which sits on the bottom of the component tree, can directly access the value
attribute from the parent component. The prop
value does not have to be manually passed through every tree level.
The .createContext()
API comes with the core React library, so we must import it first. Then we create a sampleContext
variable, which will store the Context object
.
Lastly, we import the useContext()
hook from the core React library, which we will use to get the value from Context API. You can look at live codesandbox.
Use the React.createContext()
in React
The React.createContext()
is the main method for returning Context object
. The variable that stores the returned object should be created outside of component definitions.
The useEffect()
hook is only used to get the value from the Context object
, not to create it. React.createContext()
takes one argument: the default value for Context object
.
React will only read the default value
if the child component
doesn’t have a Provider in its tree. For this reason, React developers often use the default value to test components in isolation.
The Context object
holds a custom Provider
component. It allows child components to subscribe to the value in the context object.
If you set the contextType
property to the context object, you can access the value from the Provider
component by reading the component’s this.context
property.
Unfortunately, you can only subscribe to one Provider
component. You can create multiple custom Provider components for different Context objects
.
These can be nested at various levels in the component tree. In this case, the child component will read the value of the custom Provider
component of the nearest parent component.
The Provider
component takes one value
prop, which can assign a value you need to access in children components. It can be connected to multiple children simultaneously, as long as its descendants are in the component tree.
Every time the value
prop of the Provider
component changes, all children components subscribed to it will re-render. Normally, component re-rendering in React is handled by .shouldComponentUpdate()
method.
However, this method does not handle the updates for child components that consume the value from Provider
. Instead, the child components compare the previous value with the new one and update any changes.
Since the re-rendering isn’t handled by the .shouldComponentUpdate()
method, the re-render of the parent component does not automatically re-render the child.
Use the useContext()
Hook in React
There are multiple ways to consume the value from the Context object.
First off, you can set the contextType
property of a class component. It must be set to the context object.
The second way to access the value is to use the Context.Consumer
custom component. The child for this custom component must be a function suitable for functional components.
However, the easiest way to subscribe to the Provider
component is to use the useContext()
hook. As you can see in the practical example above, it accepts one argument, and it must be a context object.
This way, you can access the value
prop of the Provider
component and store it in a variable. Let’s look at an example again:
function Grandchild() {
const value = useContext(sampleContext)
return <div className="grandchild" style={{fontSize: 30}}>{value}</div>;
}
The value
variable stores the value from the custom Provider
component. For better demonstration, take a look at codesandbox.
Use the Redux Library in React
Redux is a library for managing the state of complex React applications. It is separate from React and developed by a different team.
Redux is the most popular library and a viable alternative to Context API
and a more advanced library with rich features. It can be a replacement for Context API
. However, Context API
does not have all the features of Redux.
Both Context API
and Redux library
can pass down the data to components on the lower part of the tree. Unlike Context API
, Redux comes with Redux DevTools
.
Redux DevTools
lets you track your state updates. Redux also allows you to use middleware
and many other advanced features not available with Context API
.
When to Use the Context API vs. The Redux in React
You don’t need Redux to share the data to children components without manually passing down the props
if that’s your primary motivation for using Redux.
It would be best if you considered using Context API
instead. It has a more straightforward interface, and it’s easier to set up.
If using middleware
is essential to making your React application work, Redux is better. Redux also comes with additional libraries, such as redux-persist
to store the data locally.
To get more information about advanced features of Redux, check out this blog.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn