The alert Method in React
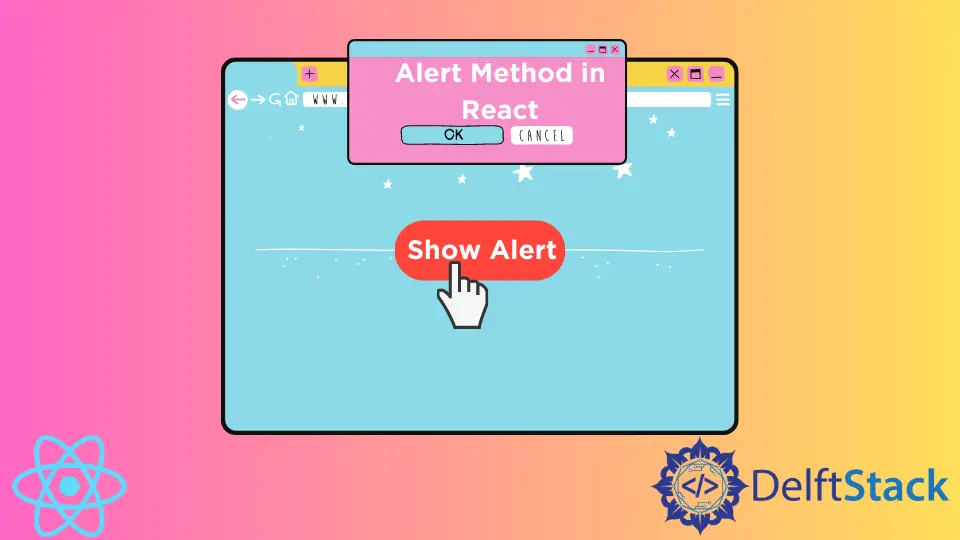
Customers like to use web applications with interactive features. Maintaining high user experience standards will ensure that your users stay longer and return to your website.
Communicating with customers is one of the essential elements of maintaining a good UX. For example, you can use red text to tell users something is wrong.
Web developers can use many other methods to communicate with users. In this article, we will explore the alert()
method and how it can be used to communicate with users.
Use window.alert()
in React
The alert()
method is often used to display a message to the user. This method displays a dialog box, which can be used by clicking a button.
The popup message appears in the center of the page and limits users’ ability to interact with your app.
It highlights the message in the alert, so the method should be used for communicating something important. However, users can continue using your application when they dismiss the message.
In an ideal scenario, window.alert()
should only be triggered and display a popup message if something is wrong. If there are no significant errors, you can use other, less forceful ways to communicate with users.
The method takes one argument, a string that will represent the text in the alert box. It does not return anything.
To better understand how alert()
works, let’s look at this example.
import "./styles.css";
import { useState } from "react";
export default function App() {
const [error, setError] = useState(true);
const validateField = (e) => {
setError(e.target.value.indexOf("@") === -1 ? true : false);
};
const handleEmailSubmit = (e) => {
if (error) {
alert("please enter correct e-mail");
} else {
return null;
}
};
return (
<div className="App">
<input type="text" onChange={(e) => validateField(e)}></input>
<button onClick={() => handleEmailSubmit()}>Submit E-mail</button>
</div>
);
}
If you look at the live demo on codesandbox, you’ll see that we have a simple application with an input field and a button for submitting it.
Here, we import the useState()
hook to create a state variable error
and the function to update its value. Later on, we will check this state variable to decide whether or not to alert users and tell them to enter their email correctly.
We will set the state variable to true
by default because submitting an empty field should cause an error.
In the validateField()
function, we check if the value in the text input is a real email and update the error
state variable accordingly.
Specifically, we check if the text input contains the @
symbol, essential for any email. We use the ternary operator to update the state variable to true
if there is no @
symbol in the text field.
If otherwise, we will set the error
variable to false
.
We also have a handleEmailSubmit()
function, executed when the user clicks the button in our app. It checks the value of the error
state variable, and if there is an error, it calls the alert()
function.
In JSX, we have a <div>
container with two elements, <input>
and <button>
. The text input has an onChange
event handler, which will check if the input contains the @
symbol every time its value changes and update the value of the error
variable accordingly.
The button’s onClick
event handler calls the handleEmailSubmit
function, which displays an alert if there’s an error.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn