How to Set a Component Style After onScroll Event
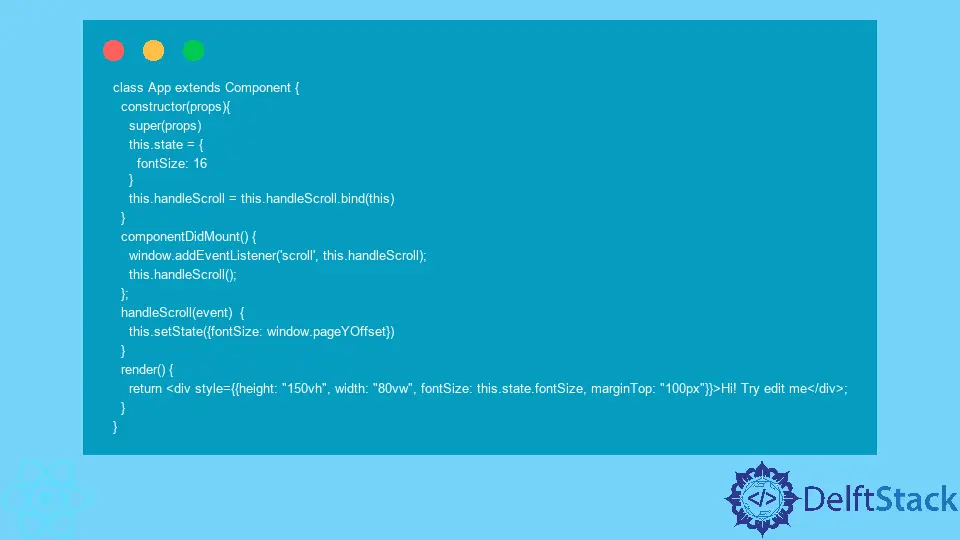
When developing React applications, often it’s necessary to listen to users’ input and update the state accordingly. For instance, you might want to highlight the components that are currently in the user’s view.
To conditionally update a component’s styles, it is recommended that you use the CSS-in-JS
solutions, like inline components or the styled-components
library.
Before proceeding any further, you must set up an event listener for scroll
event: window.addEventListener('scroll')
. Then you can define a handler function for the scroll event.
React onScroll
Event for Class Components
Despite a slightly different design, React class components are pretty similar to functional components. One big difference between the two is that class components don’t have hooks. Instead, we can use one of the lifecycle methods.
componentDidMount()
To keep things simple, let’s use a lifecycle method with the most predictable behavior. After setting up a listener for a scroll
event, we must pass a handler as a second argument to the window.addEventListener()
method. Then, within the lifecycle method, we must call the handler. Let’s look at this example from playcode.io:
class App extends Component {
constructor(props){
super(props)
this.state = {
fontSize: 16
}
this.handleScroll = this.handleScroll.bind(this)
}
componentDidMount() {
window.addEventListener('scroll', this.handleScroll);
this.handleScroll();
};
handleScroll(event) {
this.setState({fontSize: window.pageYOffset})
}
render() {
return <div style={{height: "150vh", width: "80vw", fontSize: this.state.fontSize, marginTop: "100px"}}>Hi! Try edit me</div>;
}
}
Every time the <div>
element is scrolled, our component will re-render because of the changes in the state. The handler updates the state based on the window object’s pageYOffset
property. This value of window.pageYOffset
shows how far we’ve scrolled down.
Head over to playcode.io and try it out for yourself. The further you scroll down, the bigger the font is going to be.
React onScroll
Event for Functional Components
To trigger the changes based on the scroll event, first, you must set up a listener for this event. This can be done by calling the window.addEventListener('scroll')
method. Note that you must pass a string that specifies the type of event you’re listening to in the call. In this case, we pass 'scroll'
. The second argument should be a reference to the event handler.
useEffect()
You can think of useEffect()
as a combination of all class components’ lifecycle hooks. In this case, to replicate the behavior of the componentDidMount()
hook, we’re going to pass an empty array as a second argument. Example:
const [fontSize, setFontSize] = useState(0);
const handleScroll = () => setFontSize(window.pageYOffset)
useEffect(() => {
window.addEventListener('scroll', handleScroll)
}, []);
The value of the fontSize
variable will change dynamically. You can reference it in your inline styles to change the element’s style.
<div style={{height: "150vh", width: "80vw", fontSize: fontSize, marginTop: "100px"}}>Hi! Try edit me</div>;
Note that we pass a simple reference to the state variable in functional components, not to the this.state
object.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn