onKeyPress in React
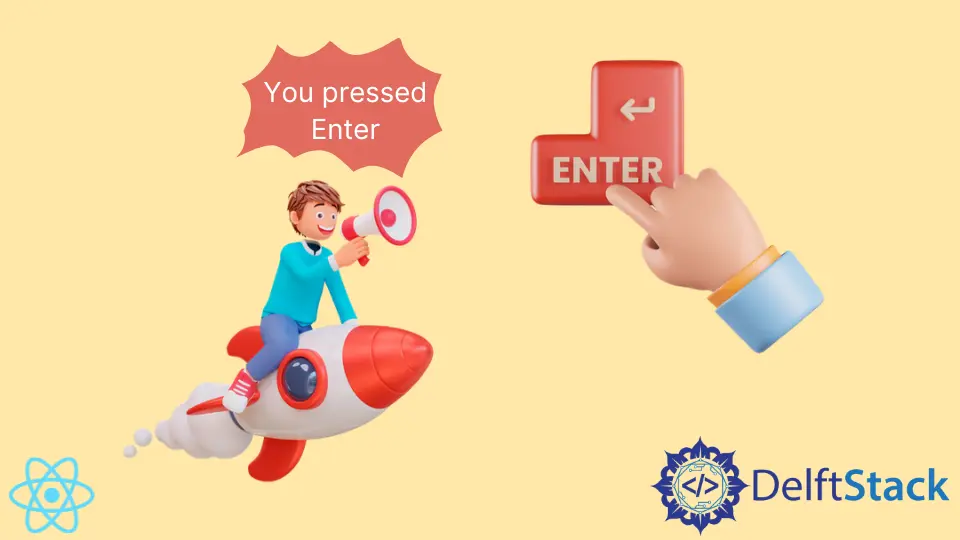
onKeyPress
is part of the interface for text fields. It can be used in all major JavaScript frameworks, including React. This event handler can be useful when you want to execute a function every time the users type a letter, a number, or something else in your fields. You can also specify which key should trigger the execution of an event.
onKeyPress
in React
According to Official documentation on MDN, the onKeyPress
event is deprecated. The developers at MDN do not recommend using it. However, don’t lose hope - there is a viable alternative, which we’ll discuss in later sections of the article.
If you want to go with the deprecated onKeyPress
event, here’s how to handle the onKeyPress
event in React.
class App extends Component {
render() {
console.log('App started');
this.handleKeyPress = (e) => {
if (e.key === 'Enter') {
console.log('You must have pressed Enter ')
}
} return (<div><input type = 'textfield' id = 'text' onKeyPress = {
this.handleKeyPress
} />
</div>);
}
}
This solution works in React version 0.14.7, and on the playcode.io React playground.
This solution uses a named key
attribute to check whether the user pressed down the Enter key or not.
if (e.key === 'Enter') {
console.log('You must have pressed Enter ')
}
Alternatively, you can also use charCode
or charKey
attributes to fire the event after the user presses a specific key. Again, let’s see how we can conditionally check that the user has pressed the Enter key, which has a charCode
of 13.
Code example:
if (e.charCode === 13) {
console.log('You must have pressed Enter ')
}
If you replaced the previously used conditional with this one, the result would be the same. In fact, the charCode
property is safer to use, because it is more widely supported in Browsers than named key
attributes.
keyCode
attribute is less predictable. In the above example, if we console.log(e.keyCode)
, we’re going to see a 0
. This is because some new coding environments don’t support it. If you encounter this problem while coding an app, you can use charCode
as an alternative.
onKeyPress
vs onKeyDown
in React
onKeyPress
was deprecated by the developers of HTML. In autumn 2021, many major browsers still support it, but once the newer browser versions are released, the support for onKeyPress
will dwindle. So unless you have a very specific reason for using onKeyPress
, use onKeyDown
instead, which does basically the same thing, with slight differences.
The keyPress
event fires only for the keyboard keys that produce alphanumeric values. These include all of the letters, numbers, punctuation, and keys used to type spaces. Modifier keys, such as Alt, Shift, or Ctrl can not fire onKeyPress
event.
onKeyDown
is fired every time any of the keys is pressed down. There’s no difference between the keys that produce alphanumeric values and those that don’t. Pressing the keyboard keys like Backspace and Caps Lock will generate the onKeyDown
event, but not onKeyPress
.
onKeyDown
in React
Except for the minor differences mentioned above, onKeyDown
event is essentially the same as onKeyPress
. The most important difference is that it is currently supported in all major browsers, and it will be supported for years to come.
onKeyDown
is also more consistent, regardless of which React version you use.
Here’s how the same solution would work with onKeyDown
:
class App extends Component {
render() {
console.log('App started');
this.handleKeyDown = (e) => {
if (e.keyCode === 13) {
console.log('You must have pressed Enter ')
}
} return (<div><input type = 'textfield' id = 'text' onKeyDown = {
this.handleKeyDown
} />
</div>);
}
}
Note that to check the key that fired onKeyDown
event, we use keyCode
property, which wasn’t accessible with onKeyPress
.
if (e.keyCode === 13) ```
Similarly, we can also use the named `key` attribute:
```javascript
if (e.key === 'Enter') ```
Either way, if we select the input and click <kbd>Enter</kbd> two times, this is what our console will look like:

Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn