How to Use Moment.js for React Apps
-
What Is
Moment.js
-
How to Use
Moment.js
With React -
Moment.js
Practical Example -
react-moment
Package - Other Viable Alternatives
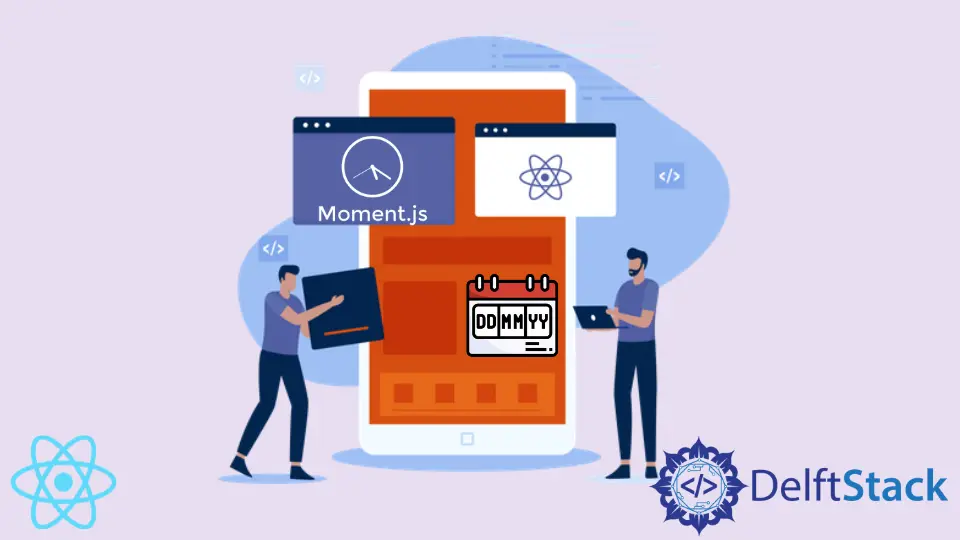
It’s hard to think of any modern web application involving dates and times. React applications are not the exception to the rule.
Managing date and time is at the heart of building dynamic applications in React. Because of this fact, JavaScript includes a Date
object, which is useful for simple operations involving date and time.
However, working with the Date
object is easier said than done. Date API is insufficient for formatting, internationalizing, and in general, manipulating the dates.
For this reason, the community of JavaScript developers has created many date libraries to perform some of the more advanced operations. These allow us to work with dates much more efficiently, without wasting time and energy.
What Is Moment.js
It is one of the most stable libraries for formatting dates in JavaScript. Moment library is mainly used for parsing and formatting data values, but it has many features that may be useful for building modern apps.
For instance, Moment
is useful for data validation as well. It works with both - Node.js and regular JavaScript.
It can be used for front-end applications as well as for server-side programming. It is also compatible with many popular JavaScript libraries, including React and TypeScript.
The library provides an API that wraps around the native Date object in JavaScript. This way, we don’t have to interact with the original Date
API or change it.
The Moment library is easy to learn and, as a bonus, supports many languages. So it can solve our internationalization problems easily.
At this moment, the library is in maintenance mode. It’s still stable, and all methods work like they’re supposed to.
However, the creators decided to stop releasing new versions. If you’re used to Moment
and want to use it for your React app, you’re free to do so.
Those concerned about the long-term future of their app might want to consider other JavaScript date libraries.
How to Use Moment.js
With React
Because Moment.js
is an external library, we must install it in our project folder. And while we’re at it, we can install the react-moment
package as well, which we’ll discuss later.
If our app needs the timezone functions, it is recommended to install moment-timezone
as well:
npm install --save moment moment-timezone
Once we’re done installing the packages, we must import them. Locate the file where we intend to use them and import them:
import moment from 'moment'
import Moment from 'react-moment'
import 'moment-timezone'
We capitalize Moment
because it is a React component created specifically for date manipulation in this library.
Moment.js
Practical Example
Let’s look at one example of using the Moment library in React.
let momentObject = moment()
In this example, we return a simple moment object, representing the code’s execution time. The Moment library includes many other methods that are much more interesting.
For instance, the .add()
method can mutate the object by adding a specific amount of time. Let’s look at an example:
moment().add(10, 'days')
As we can see, the first argument to the .add()
method specifies the quantity, and the second argument is necessary to specify the period of time. We can chain multiple methods to one another, for instance:
moment().add(10, 'days').add(1, 'months')
This code will add one more month to the date returned by the moment()
function.
react-moment
Package
react-moment
is based on the moment
package but provides the same features in a component. Let’s take a look at an example where we have to format the string as a date.
import React from 'react';
import Moment from 'react-moment';
class App extends React.Component {
render() {
return (
<Moment>{'1996-07-21T11:55-5500'}</Moment>
);
}
}
export default App
Alternatively, we can use the date
attribute on the Moment
component to pass the string. The date doesn’t even have to be a string - it can be an array, object, or the instance of the Date
object.
Moment
component provides an easy way to use the core features of the Moment library in React apps. The component accepts many props, such as: date
. format
, trim
, and many others.
For detailed information, check the official documentation.
Other Viable Alternatives
The Moment library is not the only library for working with dates in JavaScript. Packages like date-fns
provide a great alternative.
It has highly informative documentation and as many features as the Moment library. Every function in this library is documented.
Their names are user-friendly to make the function’s purpose easy to understand.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn