How to Loop Through an Object in React
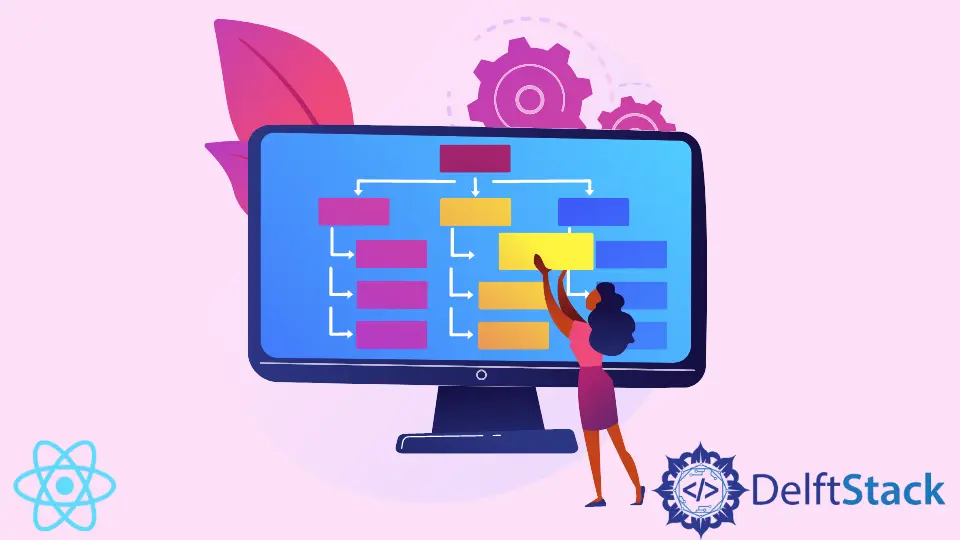
This tutorial demonstrates the use of forEach()
, Object.keys
& map()
methods to loop through an object in React.
Loop Through an Object in React
In React, we often use the .map()
method to generate components (or DOM elements) by taking data from the array.
For example, if you’re running an eCommerce store, you could have an array of objects, where each object contains detailed information about one product. Then you would loop over this array to generate components for each product.
In React, generating components by going over items in the array is a standard operation. However, looping through an object is a little more complicated and requires additional steps.
Let’s learn how?
Use Object.keys()
& map()
to Loop Through an Object in React
First, you need to generate the array of Object
properties using the Object.keys()
method, which takes the object as an argument.
Then you can use the .map()
method with this array and generate custom components for each property. Let’s look at this example:
export default function App() {
const person = {
firstName: "Irakli",
lastName: "Tchigladze",
age: 24,
occupation: "writer"
};
return (
<div className="App">
{Object.keys(person).map((key) => (
<h1>{key + " - " + person[key]}</h1>
))}
</div>
);
}
Here, we have a person
object with four properties to describe the person. Within JSX
, we use the Object.keys()
method to get the list of all object properties.
Then we use a .map()
method to return a new array of <h1>
elements that display the object property and its value. See the output below.
Output:
firstName - Irakli
lastName - Tchigladze
age - 24
occupation - writer
Use the forEach()
Method to Loop Through an Object in React
You can also successfully loop over the properties of an object by using the forEach()
method. First, however, it’s essential to understand the behaviour of this method.
It does not return a new array composed of modified items like the .map()
method does. Instead, we are required to create a new array, modify each item in the array and use the push()
method to add the modified item to the new array.
Let’s look at an example below:
const modifiedArray = [];
Object.keys(person).forEach(function (key) {
modifiedArray.push(<h1>{key + " - " + person[key]}</h1>);
});
Then you can reference the modifiedArray
within JSX
as follows:
<div className="App">{modifiedArray}</div>
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn