How to Apply Inline Styles in React
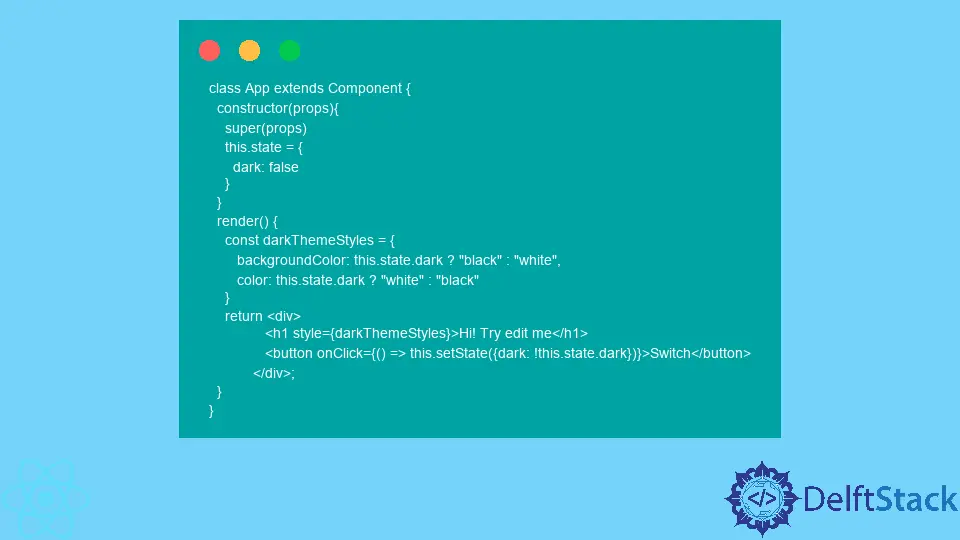
Styling HTML elements or components is at the heart of the front-end developer’s job. Styles are essential to ensure the proper placement and appearance of the HTML element. React developers apply styles using many different approaches. One of the most popular ways is to use inline styles. In today’s article, we wanted to discuss the benefits and drawbacks of using inline styles.
Inline Style in React
React developers usually have a preferred way to style their components. Some create separate CSS files for every component, while others combine all their styles in one file. When writing styles for an entire application in one file, you’re much more likely to run out of descriptive (and unique) names for classes.
Inline style definitions allow you to style components without creating two separate files for JavaScript and CSS. Styles defined this way are more readable than global CSS rules, and when using them, you’re less likely to run into naming issues.
Syntax
Inline styles in React work the way you’d expect if you’ve used them in HTML. Just like in HTML, inline styles in React are set using the style
attribute. Also, similarly to HTML, inline styles override the classes in React as well.
Now, let’s talk about differences. To style your components or elements in JSX, you must provide an object (or reference to an object) representing the styles. To make sure that JSX can decipher your style object, you must place it within a pair of curly braces. Here’s what a simple inline style declaration would look like:
<h1 style={{display: "none"}}>Hi! Try edit me</h1>;
In this example, the outer layer of curly braces ensures that the object within them is evaluated as a JavaScript expression.
When it comes to syntax, probably the biggest difference between inline styles in React vs HTML is that you can’t use regular CSS property names in React inline styles. Properties consisting of only one word are usually the same, but those consisting of multiple words are usually combined into one word and written in camelCase
. For instance, the font-size
property becomes fontSize
in React styles.
Essentially, inline styles in React are represented as an object, with key-value pairs that correspond to CSS properties and their values. A comma must separate Key-value pairs; otherwise, you might get an error.
React Conditional Inline Style
Your JSX code can contain JavaScript objects which can be compiled into valid styles. This is an extremely useful feature because it allows us to apply styles conditionally. We can use the JavaScript ternary operator within the curly braces to set up complex conditions to describe the instances when the styles should apply.
Let’s imagine that our website has a dark theme and a light theme. We can use the dark
property in the component’s state
object to conditionally switch between the two themes. Here’s an example.
class App extends Component {
constructor(props){
super(props)
this.state = {
dark: false
}
}
render() {
return <div>
<h1 style={{backgroundColor: this.state.dark ? "black" : "white", color: this.state.dark ? "white" : "black"}}>
Hi! Try edit me</h1>
<button onClick={() => this.setState({dark: !this.state.dark})}>Switch</button>
</div>;
}
}
The above is a simple demonstration of how useful inline styles can be.
In the code snippet above, you might’ve noticed that our style object is too long and can be hard to follow. Instead of writing the entire object within the curly braces, we can define it somewhere else and reference it. When you notice that your inline style object contains too many rules, move it outside the JSX. You can create a variable with a descriptive name to store the style object and use the variable name to reference it. This way, your styles are easier to read. Here’s an example.
class App extends Component {
constructor(props){
super(props)
this.state = {
dark: false
}
}
render() {
const darkThemeStyles = {
backgroundColor: this.state.dark ? "black" : "white",
color: this.state.dark ? "white" : "black"
}
return <div>
<h1 style={darkThemeStyles}>Hi! Try edit me</h1>
<button onClick={() => this.setState({dark: !this.state.dark})}>Switch</button>
</div>;
}
}
This way, the JSX code under your return
statement is easier to read and looks much cleaner.
Some React developers love inline styles because they’re easily readable, and their scope is limited to a single component instead of being globally defined. People working on a component can easily understand how the component is styled.
However, there are some cases when CSS is still useful. For instance, it has :hover
and many other selectors, which JavaScript does not have.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn