How to Use Google Font in React Native
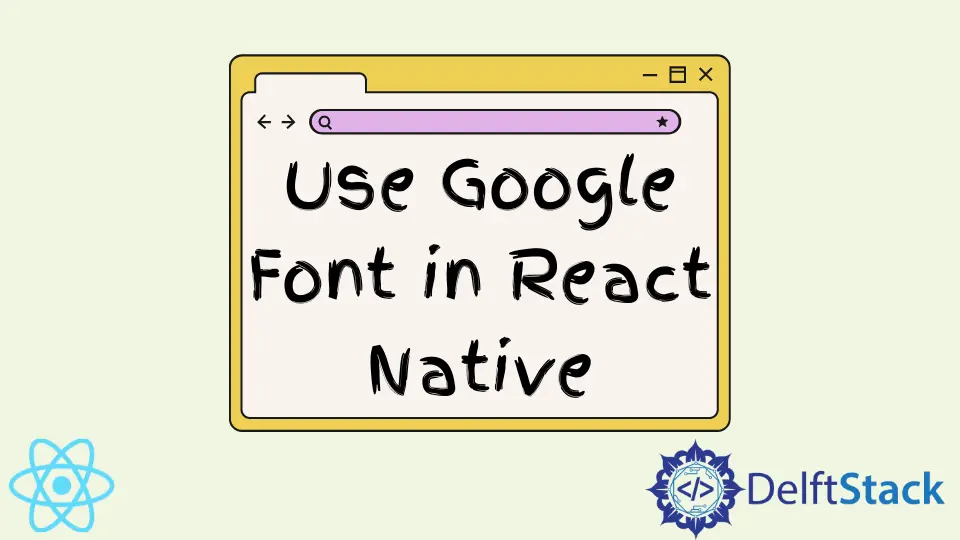
This post will demonstrate how you use the expo-google-fonts
library to import unique fonts into the React native application.
Use Google Font in React Native
Our program needs some boilerplate added as a starting step. Into your program, add the following code:
import {StatusBar} from 'expo-status-bar';
import {StyleSheet, Text, View} from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello World!</Text>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#000",
alignItems: "center",
justifyContent: "center",
},
text: {
fontSize: 24,
color: "white",
},
});
The above code uses the default font, and it looks something like this:
The moment has come to add Expo-Google-Fonts
and Expo-Font
to our project now that our boilerplate has been configured.
The first step is to install the libraries using the expo
. You can get expo-google-font
from here.
You can install expo-google-fonts
like this:
expo install @expo-google-fonts/finger-paint
Add the following import lines to your app’s header after that.
import {FingerPaint_400Regular, useFonts,} from '@expo-google-fonts/finger-paint';
We will use the FingerPaint_400Regular
font in this application, and useFonts
will help load our application.
The useFonts
hook will then be used to load our font into our program. The font family of our text style will then be set to FingerPaint_400Regular
.
import {FingerPaint_400Regular, useFonts,} from '@expo-google-fonts/finger-paint';
import {StatusBar} from 'expo-status-bar';
import {StyleSheet, Text, View} from 'react-native';
export default function App() {
let [fontsLoaded] = useFonts({
FingerPaint_400Regular,
});
return (
<View style={styles.container}>
<Text style={styles.text}>Hello World!</Text>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#000",
alignItems: "center",
justifyContent: "center",
},
text: {
fontSize: 24,
color: "white",
fontFamily: "FingerPaint_400Regular",
},
});
Most likely, the font did not change, or if it did, it did not change to the type you anticipated. This is because our application loads before our typeface do.
To correct this, we must utilize the AppLoading
component offered by React Native to maintain the visibility of our splash screen until our font is loaded.
The AppLoading
component must first be added to our project before utilizing it. To install the component, enter the following code into your terminal:
expo install expo-app-loading
And then import it into your app’s header:
import AppLoading from 'expo-app-loading';
We must perform some conditional rendering in our app to leverage AppLoading
.
The AppLoading
component will be displayed before our font has loaded. But after the fonts have loaded correctly, we’ll show our main content instead.
The code is as follows:
import {FingerPaint_400Regular, useFonts,} from '@expo-google-fonts/finger-paint';
import AppLoading from 'expo-app-loading';
import {StatusBar} from 'expo-status-bar';
import {StyleSheet, Text, View} from 'react-native';
export default function App() {
let [fontsLoaded] = useFonts({
FingerPaint_400Regular,
});
if (!fontsLoaded) {
return <AppLoading />;
} else
return (
<View style={styles.container}>
<Text style={styles.text}>Hello World!</Text>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#000",
alignItems: "center",
justifyContent: "center",
},
text: {
fontSize: 24,
color: "white",
fontFamily: "FingerPaint_400Regular",
},
});
The following screen ought to now appear in your app:
The discussion on this topic has come to a close, but there is still something we want to bring up. The import
statements required for each font listed here may be found in the expo-google-fonts
directory if you want to use a different font.
You must install the correct font because each has its import
statement and library.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn