How to Force React Components to Rerender
- Force React Components to Rerender With the Class Components
- Force React Components to Rerender With the Function Components
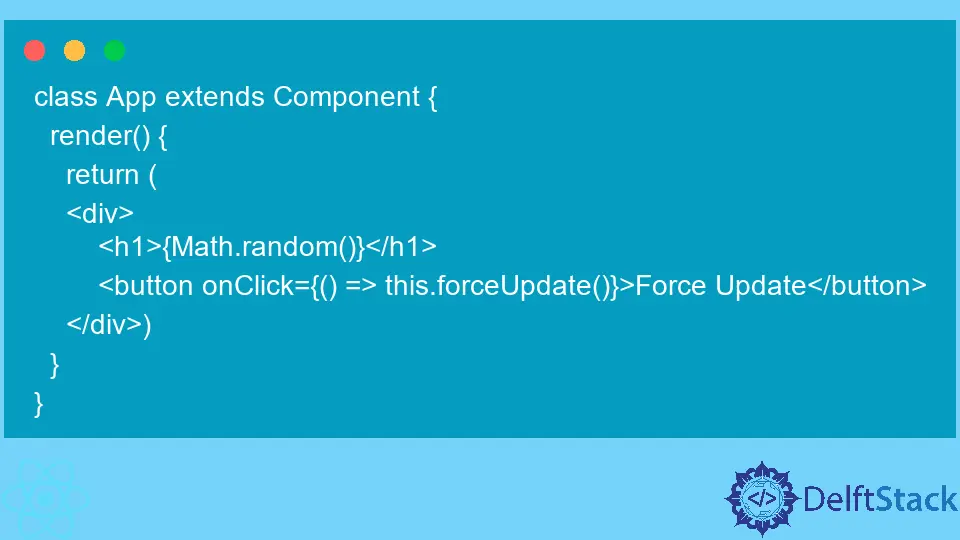
By default, the React components are triggered to re-render by the changes in their state
or props
. Most of the time, if you follow the best practices of React, this behavior is more than enough to achieve the desired results.
In some cases, the framework’s default behavior is not enough, and you need to re-render the component manually. Before you do so, carefully look over the code. Forcing a re-render is a viable option only in a limited number of circumstances.
Force React Components to Rerender With the Class Components
When used correctly, a call to React Class Component’s setState()
method should always trigger a re-render. shouldComponentUpdate()
lifecycle might contain a conditional logic that prevents this behavior.
If you’re calling setState()
but the component doesn’t update, there may be something wrong with your code. Make sure you have a valid reason to use the forceUpdate()
method.
this.forceUpdate()
If your render()
method relies on data outside of state
or props
, and you want to trigger the re-render based on changes in that data, you can use the forceUpdate()
method.
Example code:
class App extends Component {
render() {
return (
<div>
<h1>{Math.random()}</h1>
<button onClick={() => this.forceUpdate()}>Force Update</button>
</div>)
}
}
In this case, a change of state
or props
does not trigger the update. It is the forceUpdate()
method that does it.
Best Practices
React developers should only use forceUpdate()
as a last resort. The render function should only read from props
and state
. By following these guidelines, you can ensure the simplicity and efficiency of your React components.
It is not recommended to use the forceUpdate()
method frequently. If you habit using it a lot, you may want to look over your code and see if it could be improved.
Force React Components to Rerender With the Function Components
Function components do not include the forceUpdate()
method. However, it’s still possible to force them to re-render using either useState()
or useReducer
hooks.
useState
Similar to setState()
method of Class Components, useState()
hook always triggers an update, as long as the updated state
object has a different value.
You can create a custom hook that makes use of useState()
hook to force an update. Here’s a sample code for such reusable hook:
const useForceUpdate = () => {
const [ignored, newState] = useState();
return useCallback(() => newState({}), []);
}
As we all know, the useState()
hook returns two values: the current value of the state
and its setter. In this case, we only need the newState
setter.
useReducer
Since useState
hook internally uses useReducer
, you can use this hook directly to create a more elegant solution. The React official documents recommend solution uses useReducer()
as well. Here’s a sample solution:
const [any, forceUpdate] = useReducer(num => num + 1, 0);
function handleChange(){
forceUpdate();
}
useReducer()
hook returns current the state
and the dispatch
function. In the above example, we use [variableName, dispatchName]
syntax to store these values.
In this example, calling a handleChange()
handler would force your component to update every time.
Hooks aren’t intended to be used this way. Try to use these solutions for testing or outlier cases.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn