Multiple Ways to Write Comments in React
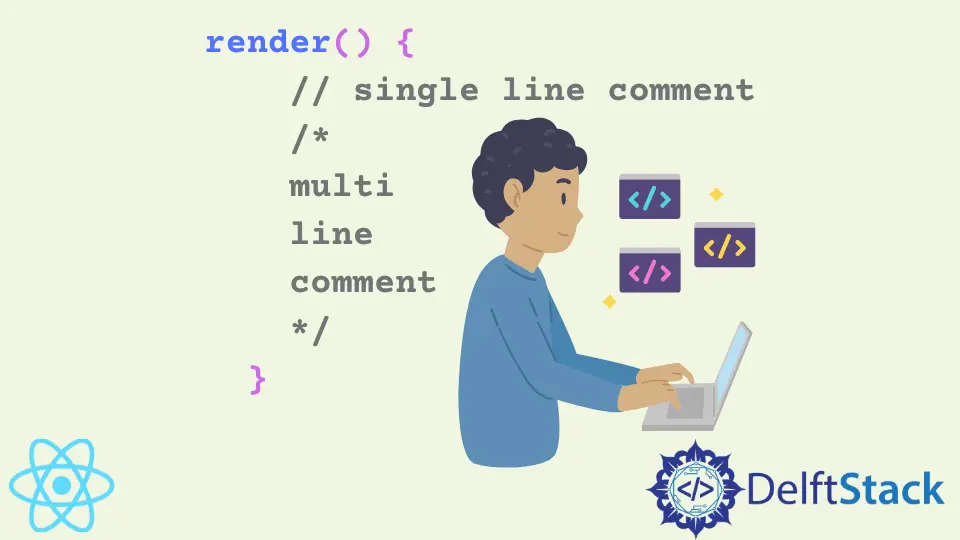
Writing helpful comments is one of the keys to achieving a more efficient coding process. They make it much easier to maintain, debug, and build upon existing web applications. Once programmers are done with a project, they move on to different tasks and forget the details of the code they just wrote. Programmers can use comments to describe their thinking and explain the most complex parts of the code. Comments allow you to come back and easily figure out the code later on. They can save you a lot of time if you need to add a feature, debug, or build upon existing code.
React components should be very simple and easy to understand in an ideal scenario, but that’s not always the case. Sometimes the purpose and functionality of the component may be unclear. Writing comments allows you to explain bits of difficult code.
Comments in React - JSX Syntax
JSX is an HTML-like syntax to facilitate writing React applications. It allows developers to structure an app intuitively instead of calling React.createElement()
methods dozens of times. Compilers take care of translating JSX code into vanilla JavaScript.
React components consist of regular JavaScript and JSX. You can write comments for both, but you have to do a bit of extra work to write comments in JSX. The creators of React decided not to allow regular JavaScript comments within JSX. They have no plans to add a separate commenting feature for JSX in the future either.
In regular JavaScript, you can use the normal syntax for writing comments. Here’s how to write comments outside JSX:
class App extends Component {
render() {
// single line comment
/*
multi
line
comment
*/
return <h1>Hi! Try edit me</h1>;
}
}
Even though it is very similar to HTML, the syntax for writing comments in JSX is different from HTML. You can’t use <!--
and -->
tags to mark the start and end of the comment.
Instead, you must use curly braces, which allow you to write vanilla JavaScript expressions within JSX. Without the curly braces, the comments in JSX will be parsed as regular text. You can type multi-line JavaScript comments in JSX as long as they’re written between curly braces.
Here’s an example:
class App extends Component {
render() {
return (
<div>
<h1>Sample heading</h1>
{/* The heading text is large */}
</div>
)
}
}
You can also write comments on multiple lines as long as you move the ending tag and both tags are still between curly braces.
Writing single-line comments in JSX is a bit more complicated. You can’t write something like {//comment}
because the //
tag automatically comments out the entire line, including the closing curly brace. If you want to add single-line comments, make sure that the closing curly braces are on the next line. Let’s take a look at the example:
class App extends Component {
render() {
return (
<div>
<h1>Sample heading</h1>
{// comment
}
</div>
)
}
}
This syntax can be a bit confusing. Most React developers stick with multi-line syntax to demarcate the start and end of the comment clearly.
If you’re interested in learning more about this, you can explore commenting in JSX on playcode.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn