How to Set Checkbox Property in React
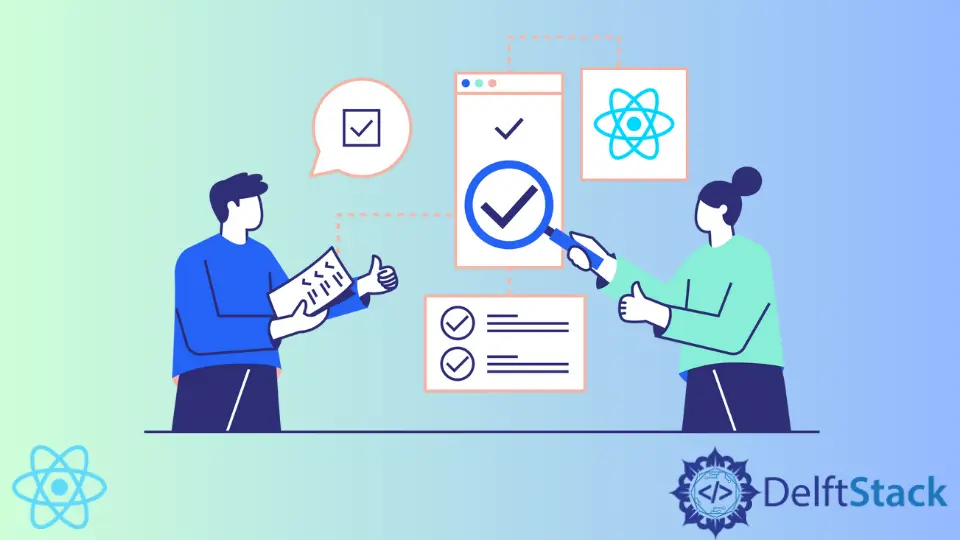
Forms defined in JSX are more than simple HTML elements. Forms are supposed to store and transfer internal data, so they need an internal state to manage it.
Let’s take HTML checkboxes as an example. In their natural state, HTML checkboxes can store the value of the checked
attribute. However, if you’re developing an app, storing the data in the element’s internal state is insufficient. You need to store the value of the checked
attribute in the internal state of your React app so that you can reference it throughout the app.
Checkbox in React
Checkbox elements in React must follow certain rules. 10 years ago, it may have been acceptable to create new attributes for HTML elements conditionally. This is against best practice recommendations in React. Any checkbox element must be created with or without the checked
attribute.
Checkbox elements initiated without the checked
attribute are uncontrolled:
<input type="checkbox" name="uncontrolled"></input>
Checkbox elements defined with checked
attribute are controlled:
<input type="checkbox" checked={true} name="controlled"></input>
A <input>
element must be initiated as a controlled or uncontrolled component. A common mistake is to set the checked
attribute to a null
or undefined
value. A JavaScript coder might expect these values to be automatically converted to a false
boolean value, but React works differently.
Confusing Error
When React encounters any attribute with a null
or undefined
value, it will ignore the attribute and not render it. Later, if the initial value becomes true
, a checked
attribute will appear again, which triggers the change from an uncontrolled component to a controlled one.
React will throw an error, telling you that components must be initialized as controlled or uncontrolled. If the checkbox component was defined without a checked
attribute, you could not add one later.
A lot of people do not know that setting the checked
attribute on a checkbox initializes it as a controlled component. That’s why they are confused by the error message, which reads:
Warning: AwesomeComponent is changing an uncontrolled input of type checkbox to be controlled. Input elements should not switch from uncontrolled to controlled (or vice versa). Decide between using a controlled or uncontrolled input element for the lifetime of the component.
Sett a Checkbox check
Property in React
Once you understand the problem, fixing it is very easy. If you intend to create a controlled checkbox component, you must ensure that the checked
attribute evaluates either to true
or false
. This is possible by setting the defaultProps
property and setting the checked
attribute to false
by default. Here’s a code sample:
class App extends Component {
constructor(props) {
super(props) this.state = {}
}
render() {
console.log('App started');
this.defaultProps = {
checked: false
} return <input type = 'checkbox' checked = {this.checked} name =
'controlled'>< /input>
}
}
Another advantage of using defaultProps
is that they only represent the default. The user can still interact with the app and change the status of the checked
attribute.
Setting the checked
attribute to true
or false
would mean that users wouldn’t be allowed to change its status.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn