How to Check the Version of React App During Runtime
- Benefits of Knowing the React Version
-
Use React
DevTools
to Check React Version During Runtime -
Check React Version Without
DevTools
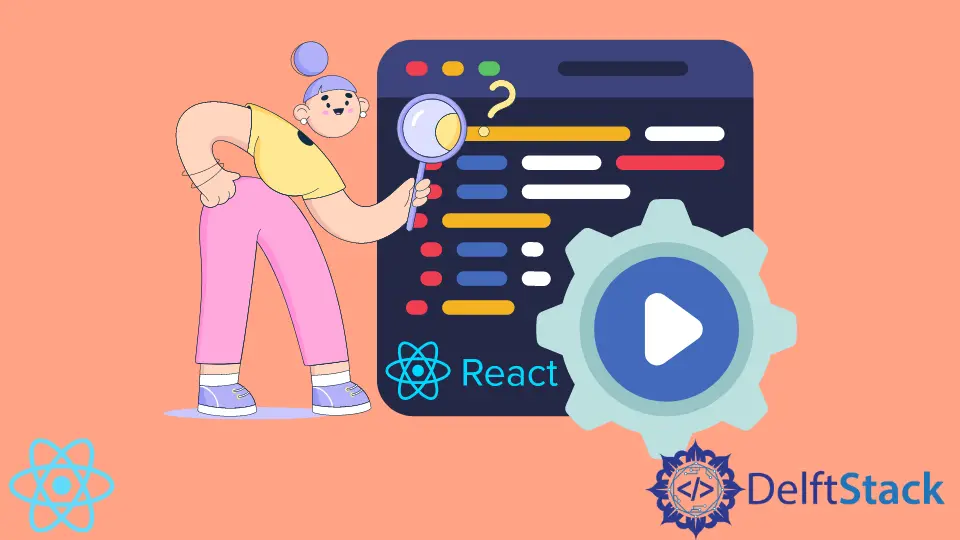
The package version is an important factor when developing front-end applications in React. The framework is constantly evolving, new features are being introduced.
Knowing the exact version of your React package is important to writing web applications free of bugs.
Benefits of Knowing the React Version
Certain features were only introduced in later versions of the framework. For instance, since React Version 16.8, hooks are available for functional components.
If you use hooks in components where they are not available, you’ll get errors.
It is also important to know the differences between versions. Sometimes you are rewriting legacy code to include the newest features in React.
This article explores how to find out the version of your React package while you’re running the app. A console is a useful tool for web development, and it’s commonly used to find the version of your framework during the runtime as well.
Use React DevTools
to Check React Version During Runtime
React DevTools
is an important part of any React developer’s toolkit. Once you have it installed, you can check the version of your React package by opening the console and entering the following code.
__REACT_DEVTOOLS_GLOBAL_HOOK__.renderers.values().next()["value"]["version"]
This command will return the version of your React web application. Let’s look at a practical example.
Check React Version Without DevTools
Another way to check the version of your React package is through the imported React module itself.
React is the core library necessary for building web applications. Once you have it installed in the environment, typically, you should import the library like below.
import React from "react";
Let’s take a look at an example of how to get the version of the React module while our application is running.
import React from "react";
export default function App() {
console.log(React.version);
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
</div>
);
}
The React.version
value will return the currently running React application version when it is read. Due to its placement, it will run every time the App
component is rendered.
Here is a live demo on CodeSandbox. If we check the app’s console, we’ll see that the value there matches the currently installed version of React.
Alternatively, you can place the React.version
value between the opening and closing tags of <h1>
. Let’s take a look at an example.
import React from "react";
export default function App() {
console.log(React.version)
return (
<div className="App">
<h1>{React.version}</h1>
</div>
);
}
Here, we use interpolation brackets, which allow us to tell JSX to interpret the expression between them as valid JavaScript.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn